JavaScript Arithmetic Operators
Arithmetic operators perform arithmetic on numbers (literals or variables).
Operator
|
Description
|
+
|
Addition
|
-
|
Subtraction
|
*
|
Multiplication
|
**
|
Exponentiation (ES2016)
|
/
|
Division
|
%
|
Modulus (Remainder)
|
++
|
Increment
|
--
|
Decrement
|
Arithmetic Operations
A typical arithmetic operation operates on two numbers.
The two numbers can be literals:
Example
let x = 100 + 50;
or variables:
Example
let x = a + b;
or expressions:
Example
let x = (100 + 50) * a;
Operators and Operands
The numbers (in an arithmetic operation) are called operands.
The operation (to be performed between the two operands) is defined by an operator.
Operand
|
Operator
|
Operand
|
100
|
+
|
50
|
Adding
The addition operator (+) adds numbers:
Example
let x = 5; let y = 2; let z = x + y;
Subtracting
The subtraction operator (-) subtracts numbers.
Example
let x = 5; let y = 2; let z = x - y;
Multiplying
The multiplication operator (*) multiplies numbers.
Example
let x = 5; let y = 2; let z = x * y;
Dividing
The division operator (/) divides numbers.
Example
let x = 5; let y = 2; let z = x / y;
Remainder
The modulus operator (%) returns the division remainder.
Example
let x = 5; let y = 2; let z = x % y;
In arithmetic, the division of two integers produces a quotient and a remainder.
In mathematics, the result of a modulo operation is the remainder of an arithmetic division.
Incrementing
The increment operator (++) increments numbers.
Example
let x = 5; x++; let z = x;
Decrementing
The decrement operator (--) decrements numbers.
Example
let x = 5; x--; let z = x;
Exponentiation
The exponentiation operator (**) raises the first operand to the power of the second operand.
Example
let x = 5; let z = x ** 2;
x ** y produces the same result as Math.pow(x,y):
Example
let x = 5; let z = Math.pow(x,2);
Operator Precedence
Operator precedence describes the order in which operations are performed in an arithmetic expression.
Example
let x = 100 + 50 * 3;
Is the result of example above the same as 150 * 3, or is it the same as 100 + 150?
Is the addition or the multiplication done first?
As in traditional school mathematics, the multiplication is done first.
JavaScript Assignment Operators
Assignment operators assign values to JavaScript variables.
Operator
|
Example
|
Same As
|
=
|
x = y
|
x = y
|
+=
|
x += y
|
x = x + y
|
-=
|
x -= y
|
x = x - y
|
*=
|
x *= y
|
x = x * y
|
/=
|
x /= y
|
x = x / y
|
%=
|
x %= y
|
x = x % y
|
**=
|
x **= y
|
x = x ** y
|
Shift Assignment Operators
Operator
|
Example
|
Same As
|
<<=
|
x <<= y
|
x = x << y
|
>>=
|
x >>= y
|
x = x >> y
|
>>>=
|
x >>>= y
|
x = x >>> y
|
Bitwise Assignment Operators
Operator
|
Example
|
Same As
|
&=
|
x &= y
|
x = x & y
|
^=
|
x ^= y
|
x = x ^ y
|
|=
|
x |= y
|
x = x | y
|
Logical Assignment Operators
Operator
|
Example
|
Same As
|
&&=
|
x &&= y
|
x = x && (x = y)
|
||=
|
x ||= y
|
x = x || (x = y)
|
??=
|
x ??= y
|
x = x ?? (x = y)
|
Note
The Logical assignment operators are ES2020.
The = Operator
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
let x = 10;
let x = 10 + y;
The += Operator
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
let x = 10; x += 5;
let text = "Hello"; text += " World";
The -= Operator
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
let x = 10; x -= 5;
The *= Operator
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
let x = 10; x *= 5;
The **= Operator
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
let x = 10; x **= 5;
The /= Operator
The Division Assignment Operator divides a variable.
Division Assignment Example
let x = 10; x /= 5;
The %= Operator
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
let x = 10; x %= 5;
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
let x = -100; x <<= 5;
The >>= Operator
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
let x = -100; x >>= 5;
JavaScript has 8 Datatypes
1. String 2. Number 3. Bigint 4. Boolean 5. Undefined 6. Null 7. Symbol 8. Object
The Object Datatype
The object data type can contain:
1. An object 2. An array 3. A date
Examples
// Numbers: let length = 16; let weight = 7.5;
// Strings: let color = "Yellow"; let lastName = "Johnson";
// Booleans let x = true; let y = false;
// Object: const person = {firstName:"John", lastName:"Doe"};
// Array object: const cars = ["Saab", "Volvo", "BMW"];
// Date object: const date = new Date("2022-03-25");
Note
A JavaScript variable can hold any type of data.
The Concept of Data Types
In programming, data types is an important concept.
To be able to operate on variables, it is important to know something about the type.
Without data types, a computer cannot safely solve this:
let x = 16 + "Volvo";
Does it make any sense to add "Volvo" to sixteen? Will it produce an error or will it produce a result?
JavaScript will treat the example above as:
let x = "16" + "Volvo";
Note
When adding a number and a string, JavaScript will treat the number as a string.
Example
let x = 16 + "Volvo";
Example
let x = "Volvo" + 16;
JavaScript evaluates expressions from left to right. Different sequences can produce different results:
JavaScript:
let x = 16 + 4 + "Volvo";
Result:
20Volvo
JavaScript:
let x = "Volvo" + 16 + 4;
Result:
Volvo164
In the first example, JavaScript treats 16 and 4 as numbers, until it reaches "Volvo".
In the second example, since the first operand is a string, all operands are treated as strings.
JavaScript Types are Dynamic
JavaScript has dynamic types. This means that the same variable can be used to hold different data types:
Example
let x; // Now x is undefined x = 5; // Now x is a Number x = "John"; // Now x is a String
JavaScript Strings
A string (or a text string) is a series of characters like "John Doe".
Strings are written with quotes. You can use single or double quotes:
Example
// Using double quotes: let carName1 = "Volvo XC60";
// Using single quotes: let carName2 = 'Volvo XC60';
You can use quotes inside a string, as long as they don't match the quotes surrounding the string:
Example
// Single quote inside double quotes: let answer1 = "It's alright";
// Single quotes inside double quotes: let answer2 = "He is called 'Johnny'";
// Double quotes inside single quotes: let answer3 = 'He is called "Johnny"';
You will learn more about strings later in this tutorial.
JavaScript Numbers
All JavaScript numbers are stored as decimal numbers (floating point).
Numbers can be written with, or without decimals:
Example
// With decimals: let x1 = 34.00;
// Without decimals: let x2 = 34;
Exponential Notation
Extra large or extra small numbers can be written with scientific (exponential) notation:
Example
let y = 123e5; // 12300000 let z = 123e-5; // 0.00123
Note
Most programming languages have many number types:
Whole numbers (integers): byte (8-bit), short (16-bit), int (32-bit), long (64-bit)
Real numbers (floating-point): float (32-bit), double (64-bit).
Javascript numbers are always one type: double (64-bit floating point).
You will learn more about numbers later in this tutorial.
JavaScript BigInt
All JavaScript numbers are stored in a 64-bit floating-point format.
JavaScript BigInt is a new datatype (ES2020) that can be used to store integer values that are too big to be represented by a normal JavaScript Number.
Example
let x = BigInt("123456789012345678901234567890");
You will learn more about BigInt later in this tutorial.
JavaScript Booleans
Booleans can only have two values: true or false.
Example
let x = 5; let y = 5; let
A JavaScript function is a block of code designed to perform a particular task.
A JavaScript function is executed when "something" invokes it (calls it).
Example
// Function to compute the product of p1 and p2 function myFunction(p1, p2) { return p1 * p2; }
JavaScript Function Syntax
A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses ().
Function names can contain letters, digits, underscores, and dollar signs (same rules as variables).
The parentheses may include parameter names separated by commas: (parameter1, parameter2, ...)
The code to be executed, by the function, is placed inside curly brackets: {}
function name(parameter1, parameter2, parameter3) { // code to be executed }
Function parameters are listed inside the parentheses () in the function definition.
Function arguments are the values received by the function when it is invoked.
Inside the function, the arguments (the parameters) behave as local variables.
Function Invocation
The code inside the function will execute when "something" invokes (calls) the function:
- When an event occurs (when a user clicks a button)
- When it is invoked (called) from JavaScript code
- Automatically (self invoked)
You will learn a lot more about function invocation later in this tutorial.
Function Return
When JavaScript reaches a return statement, the function will stop executing.
If the function was invoked from a statement, JavaScript will "return" to execute the code after the invoking statement.
Functions often compute a return value. The return value is "returned" back to the "caller":
Example
Calculate the product of two numbers, and return the result:
// Function is called, the return value will end up in x let x = myFunction(4, 3);
function myFunction(a, b) { // Function returns the product of a and b return a * b; }
Why Functions?
With functions you can reuse code
You can write code that can be used many times.
You can use the same code with different arguments, to produce different results.
The () Operator
The () operator invokes (calls) the function:
Example
Convert Fahrenheit to Celsius:
function toCelsius(fahrenheit) { return (5/9) * (fahrenheit-32); }
let value = toCelsius(77);
Accessing a function with incorrect parameters can return an incorrect answer:
Example
function toCelsius(fahrenheit) { return (5/9) * (fahrenheit-32); }
let value = toCelsius();
Accessing a function without () returns the function and not the function result:
Example
function toCelsius(fahrenheit) { return (5/9) * (fahrenheit-32); }
let value = toCelsius;
Note
As you see from the examples above, toCelsius refers to the function object, and toCelsius() refers to the function result.
Functions Used as Variable Values
Functions can be used the same way as you use variables, in all types of formulas, assignments, and calculations.
Example
Instead of using a variable to store the return value of a function:
let x = toCelsius(77); let text = "The temperature is " + x + " Celsius";
You can use the function directly, as a variable value:
let text = "The temperature is " + toCelsius(77) + " Celsius";
You will learn a lot more about functions later in this tutorial.
Local Variables
Variables declared within a JavaScript function, become LOCAL to the function.
Local variables can only be accessed from within the function.
Example
// code here can NOT use carName
function myFunction() { let carName = "Volvo"; // code here CAN use carName }
// code here can NOT use carName
Since local variables are only recognized inside their functions, variables with the same name can be used in different functions.
Local variables are created when a function starts, and deleted when the function is completed.
Real Life Objects, Properties, and Methods
In real life, a car is an object.
A car has properties like weight and color, and methods like start and stop:
Object
|
Properties
|
Methods
|
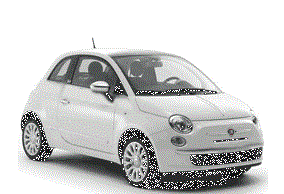
|
car.name = Fiat
car.model = 500
car.weight = 850kg
car.color = white
|
car.start()
car.drive()
car.brake()
car.stop()
|
All cars have the same properties, but the property values differ from car to car.
All cars have the same methods, but the methods are performed at different times.
JavaScript Objects
You have already learned that JavaScript variables are containers for data values.
This code assigns a simple value (Fiat) to a variable named car:
let car = "Fiat";
Objects are variables too. But objects can contain many values.
This code assigns many values (Fiat, 500, white) to a variable named car:
const car = {type:"Fiat", model:"500", color:"white"};
The values are written as name:value pairs (name and value separated by a colon).
It is a common practice to declare objects with the const keyword.
Learn more about using const with objects in the chapter: JS Const.
Object Definition
You define (and create) a JavaScript object with an object literal:
Example
const person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
Spaces and line breaks are not important. An object definition can span multiple lines:
Example
const person = { firstName: "John", lastName: "Doe", age: 50, eyeColor: "blue" };
Object Properties
The name:values pairs in JavaScript objects are called properties:
Property
|
Property Value
|
firstName
|
John
|
lastName
|
Doe
|
age
|
50
|
eyeColor
|
blue
|
Accessing Object Properties
You can access object properties in two ways:
objectName.propertyName
or
objectName["propertyName"]
Example1
person.lastName;
Example2
person["lastName"];
JavaScript objects are containers for named values called properties.
Object Methods
Objects can also have methods.
Methods are actions that can be performed on objects.
Methods are stored in properties as function definitions.
Property
|
Property Value
|
firstName
|
John
|
lastName
|
Doe
|
age
|
50
|
eyeColor
|
blue
|
fullName
|
function() {return this.firstName + " " + this.lastName;}
|
A method is a function stored as a property.
Example
const person = { firstName: "John", lastName : "Doe", id : 5566, fullName : function() { return this.firstName + " " + this.lastName; } };
In the example above, this refers to the person object:
this.firstName means the firstName property of person.
this.lastName means the lastName property of person.
What is this?
In JavaScript, the this keyword refers to an object.
Which object depends on how this is being invoked (used or called).
The this keyword refers to different objects depending on how it is used:
In an object method, this refers to the object.
|
Alone, this refers to the global object.
|
In a function, this refers to the global object.
|
In a function, in strict mode, this is undefined.
|
In an event, this refers to the element that received the event.
|
Methods like call(), apply(), and bind() can refer this to any object.
|
Note
this
HTML events are "things" that happen to HTML elements.
When JavaScript is used in HTML pages, JavaScript can "react" on these events.
HTML Events
An HTML event can be something the browser does, or something a user does.
Here are some examples of HTML events:
- An HTML web page has finished loading
- An HTML input field was changed
- An HTML button was clicked
Often, when events happen, you may want to do something.
JavaScript lets you execute code when events are detected.
HTML allows event handler attributes, with JavaScript code, to be added to HTML elements.
With single quotes:
<element event='some JavaScript'>
With double quotes:
<element event="some JavaScript">
In the following example, an onclick attribute (with code), is added to a <button> element:
Example
<button onclick="document.getElementById('demo').innerHTML = Date()">The time is?</button>
In the example above, the JavaScript code changes the content of the element with id="demo".
In the next example, the code changes the content of its own element (using this.innerHTML):
Example
<button onclick="this.innerHTML = Date()">The time is?</button>
JavaScript code is often several lines long. It is more common to see event attributes calling functions:
Example
<button onclick="displayDate()">The time is?</button>
Common HTML Events
Here is a list of some common HTML events:
Event
|
Description
|
onchange
|
An HTML element has been changed
|
onclick
|
The user clicks an HTML element
|
onmouseover
|
The user moves the mouse over an HTML element
|
onmouseout
|
The user moves the mouse away from an HTML element
|
onkeydown
|
The user pushes a keyboard key
|
onload
|
The browser has finished loading the page
|
The list is much longer: W3Schools JavaScript Reference HTML DOM Events.
JavaScript Event Handlers
Event handlers can be used to handle and verify user input, user actions, and browser actions:
- Things that should be done every time a page loads
- Things that should be done when the page is closed
- Action that should be performed when a user clicks a button
- Content that should be verified when a user inputs data
- And more ...
Many different methods can be used to let JavaScript work with events:
- HTML event attributes can execute JavaScript code directly
- HTML event attributes can call JavaScript functions
- You can assign your own event handler functions to HTML elements
- You can prevent events from being sent or being handled
- And more ...
You will learn a lot more about events and event handlers in the HTML DOM chapters.
Strings are for storing text
Strings are written with quotes
Using Quotes
A JavaScript string is zero or more characters written inside quotes.
Example
let text = "John Doe";
You can use single or double quotes:
Example
let carName1 = "Volvo XC60"; // Double quotes let carName2 = 'Volvo XC60'; // Single quotes
Note
Strings created with single or double quotes works the same.
There is no difference between the two.
Quotes Inside Quotes
You can use quotes inside a string, as long as they don't match the quotes surrounding the string:
Example
let answer1 = "It's alright"; let answer2 = "He is called 'Johnny'"; let answer3 = 'He is called "Johnny"';
Template Strings
Templates were introduced with ES6 (JavaScript 2016).
Templates are strings enclosed in backticks (`This is a template string`).
Templates allow single and double quotes inside a string:
Example
let text = `He's often called "Johnny"`;
Note
Templates are not supported in Internet Explorer.
String Length
To find the length of a string, use the built-in length property:
Example
let text = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let length = text.length;
Escape Characters
Because strings must be written within quotes, JavaScript will misunderstand this string:
let text = "We are the so-called "Vikings" from the north.";
The string will be chopped to "We are the so-called ".
To solve this problem, you can use an backslash escape character.
The backslash escape character () turns special characters into string characters:
Code
|
Result
|
Description
|
'
|
'
|
Single quote
|
"
|
"
|
Double quote
|
\
|
|
Backslash
|
Examples
" inserts a double quote in a string:
let text = "We are the so-called "Vikings" from the north.";
' inserts a single quote in a string:
let text= 'It's alright.';
\ inserts a backslash in a string:
let text = "The character \ is called backslash.";
Six other escape sequences are valid in JavaScript:
Code
|
Result
|
b
|
Backspace
|
f
|
Form Feed
|
n
|
New Line
|
r
|
Carriage Return
|
t
|
Horizontal Tabulator
|
v
|
Vertical Tabulator
|
Note
The 6 escape characters above were originally designed to control typewriters, teletypes, and fax machines. They do not make any sense in HTML.
Breaking Long Lines
For readability, programmers often like to avoid long code lines.
A safe way to break up a statement is after an operator:
Example
document.getElementById("demo").innerHTML = "Hello Dolly!";
A safe way to break up a string is by using string addition:
Example
document.getElementById("demo").innerHTML = "Hello " + "Dolly!";
Template Strings
Templates were introduced with ES6 (JavaScript 2016).
Templates are strings enclosed in backticks (`This is a template string`).
Templates allow multiline strings:
Example
let text = `The quick brown fox jumps over the lazy dog`;
Note
Templates are not supported in Internet Explorer.
JavaScript Strings as Objects
Normally, JavaScript strings are primitive values, created from literals:
let x = "John";
But strings can also be defined as objects with the keyword new:
let y = new String("John");
Example
let x = "John"; let y = new String("John");
Do not create Strings objects.
The new keyword complicates the code and slows down execution speed.
String objects can produce unexpected results:
When using the == operator, x and y are equal:
Basic String Methods
Javascript strings are primitive and immutable: All string methods produces a new string without altering the original string.
JavaScript String Length
The length property returns the length of a string:
Example
let text = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let length = text.length;
Extracting String Characters
There are 4 methods for extracting string characters:
- The at(position) Method
- The charAt(position) Method
- The charCodeAt(position) Method
- Using property access [] like in arrays
JavaScript String charAt()
The charAt() method returns the character at a specified index (position) in a string:
Example
let text = "HELLO WORLD"; let char = text.charAt(0);
JavaScript String charCodeAt()
The charCodeAt() method returns the code of the character at a specified index in a string:
The method returns a UTF-16 code (an integer between 0 and 65535).
Example
let text = "HELLO WORLD"; let char = text.charCodeAt(0);
JavaScript String at()
ES2022 introduced the string method at():
Examples
Get the third letter of name:
const name = "W3Schools"; let letter = name.at(2);
Get the third letter of name:
const name = "W3Schools"; let letter = name[2];
The at() method returns the character at a specified index (position) in a string.
The at() method is supported in all modern browsers since March 2022:
Note
The at() method is a new addition to JavaScript.
It allows the use of negative indexes while charAt() do not.
Now you can use myString.at(-2) instead of charAt(myString.length-2).
Browser Support
at() is an ES2022 feature.
JavaScript 2022 (ES2022) is supported in all modern browsers since March 2023:
|
|
|
|
|
Chrome 94
|
Edge 94
|
Firefox 93
|
Safari 16.4
|
Opera 79
|
Sep 2021
|
Sep 2021
|
Oct 2021
|
Mar 2023
|
Oct 2021
|
Property Access [ ]
Example
let text = "HELLO WORLD"; let char = text[0];
Note
Property access might be a little unpredictable:
· It makes strings look like arrays (but they are not)
· If no character is found, [ ] returns undefined, while charAt() returns an empty string.
· It is read only. str[0] = "A" gives no error (but does not work!)
Example
let text = "HELLO WORLD"; text[0] = "A"; // Gives no error, but does not work
Extracting String Parts
There are 3 methods for extracting a part of a string:
- slice(start, end)
- substring(start, end)
- substr(start, length)
JavaScript String slice()
slice() extracts a part of a string and returns the extracted part in a new string.
The method takes 2 parameters: start position, and end position (end not included).
Example
Slice out a portion of a string from position 7 to position 13:
let text = "Apple, Banana, Kiwi"; let part = text.slice(7, 13);
Note
String Search Methods
String indexOf() String lastIndexOf() String search()
See Also:
Basic String Methods String Templates
|
String match() String matchAll() String includes() String startsWith() String endsWith()
|
JavaScript String indexOf()
The indexOf() method returns the index (position) of the first occurrence of a string in a string, or it returns -1 if the string is not found:
Example
let text = "Please locate where 'locate' occurs!"; let index = text.indexOf("locate");
Note
JavaScript counts positions from zero.
0 is the first position in a string, 1 is the second, 2 is the third, ...
JavaScript String lastIndexOf()
The lastIndexOf() method returns the index of the last occurrence of a specified text in a string:
Example
let text = "Please locate where 'locate' occurs!"; let index = text.lastIndexOf("locate");
Both indexOf(), and lastIndexOf() return -1 if the text is not found:
Example
let text = "Please locate where 'locate' occurs!"; let index = text.lastIndexOf("John");
Both methods accept a second parameter as the starting position for the search:
Example
let text = "Please locate where 'locate' occurs!"; let index = text.indexOf("locate", 15);
The lastIndexOf() methods searches backwards (from the end to the beginning), meaning: if the second parameter is 15, the search starts at position 15, and searches to the beginning of the string.
Example
let text = "Please locate where 'locate' occurs!"; text.lastIndexOf("locate", 15);
JavaScript String search()
The search() method searches a string for a string (or a regular expression) and returns the position of the match:
Examples
let text = "Please locate where 'locate' occurs!"; text.search("locate");
let text = "Please locate where 'locate' occurs!"; text.search(/locate/);
Did You Notice?
The two methods, indexOf() and search(), are equal?
They accept the same arguments (parameters), and return the same value?
The two methods are NOT equal. These are the differences:
- The search() method cannot take a second start position argument.
- The indexOf() method cannot take powerful search values (regular expressions).
You will learn more about regular expressions in a later chapter.
JavaScript String match()
The match() method returns an array containing the results of matching a string against a string (or a regular expression).
Examples
Perform a search for "ain":
let text = "The rain in SPAIN stays mainly in the plain"; text.match("ain");
Perform a search for "ain":
let text = "The rain in SPAIN stays mainly in the plain"; text.match(/ain/);
Perform a global search for "ain":
let text = "The rain in SPAIN stays mainly in the plain"; text.match(/ain/g);
Perform a global, case-insensitive search for "ain":
let text = "The rain in SPAIN stays mainly in the plain"; text.match(/ain/gi);
Note
If a regular expression does not include the g modifier (global search), match() will return only the first match in the string.
Read more about regular expressions in the chapter JS RegExp.
JavaScript String matchAll()
The matchAll() method returns an iterator containing the results of matching a string against a string (or a regular expression).
Example
const iterator = text.matchAll("Cats");
If the parameter is a regular expression, the global flag (g) must be set, otherwise a TypeError is thrown.
Example
const iterator = text.matchAll(/Cats/g);
If you want to search case insensitive, the insensitive flag (i) must be set:
Example
const iterator = text.matchAll(/Cats/gi);
Notes
matchAll() is an ES2020 feature.
matchAll() does not work in Internet Explorer.
JavaScript String includes()
The includes() method returns true if a string contains a specified value.
Otherwise it returns false.
Examples
Check if a string includes "world":
let text = "Hello world, welcome to the universe."; text.includes("world");
Check if a string includes "world". Start at position 12:
let text = "Hello world, welcome to the universe."; text.includes("world", 12);
Notes
includes() is case sensitive.
includes() is an ES6 feature.
includes() is not supported in Internet Explorer.
JavaScript String startsWith()
The startsWith() method returns
String Templates
Template Strings
Template Literals
Beloved child has many names
Back-Tics Syntax
Template Strings use back-ticks (``) rather than the quotes ("") to define a string:
Example
let text = `Hello World!`;
Quotes Inside Strings
Template Strings allow both single and double quotes inside a string:
Example
let text = `He's often called "Johnny"`;
Multiline Strings
Template Strings allow multiline strings:
Example
let text = `The quick brown fox jumps over the lazy dog`;
Interpolation
Template String provide an easy way to interpolate variables and expressions into strings.
The method is called string interpolation.
The syntax is:
${...}
Variable Substitutions
Template Strings allow variables in strings:
Example
let firstName = "John"; let lastName = "Doe";
let text = `Welcome ${firstName}, ${lastName}!`;
Automatic replacing of variables with real values is called string interpolation.
Expression Substitution
Template Strings allow expressions in strings:
Example
let price = 10; let VAT = 0.25;
let total = `Total: ${(price * (1 + VAT)).toFixed(2)}`;
Automatic replacing of expressions with real values is called string interpolation.
HTML Templates
Example
let header = "Template Strings"; let tags = ["template strings", "javascript", "es6"];
let html = `<h2>${header}</h2><ul>`; for (const x of tags) { html += `<li>${x}</li>`; }
html += `</ul>`;
Browser Support
Template Strings is an ES6 feature (JavaScript 2015).
ES6 is fully supported in all modern browsers since June 2017:
|
|
|
|
|
Chrome 51
|
Edge 15
|
Firefox 54
|
Safari 10
|
Opera 38
|
May 2016
|
Apr 2017
|
Jun 2017
|
Sep 2016
|
Jun 2016
|
Template Strings is not supported in Internet Explorer.
Complete String Reference
For a complete String reference, go to our:
Complete JavaScript String Reference.
The reference contains descriptions and examples of all string properties and methods.
JavaScript has only one type of number. Numbers can be written with or without decimals.
Example
let x = 3.14; // A number with decimals let y = 3; // A number without decimals
Extra large or extra small numbers can be written with scientific (exponent) notation:
Example
let x = 123e5; // 12300000 let y = 123e-5; // 0.00123
JavaScript Numbers are Always 64-bit Floating Point
Unlike many other programming languages, JavaScript does not define different types of numbers, like integers, short, long, floating-point etc.
JavaScript numbers are always stored as double precision floating point numbers, following the international IEEE 754 standard.
This format stores numbers in 64 bits, where the number (the fraction) is stored in bits 0 to 51, the exponent in bits 52 to 62, and the sign in bit 63:
Value (aka Fraction/Mantissa)
|
Exponent
|
Sign
|
52 bits (0 - 51)
|
11 bits (52 - 62)
|
1 bit (63)
|
Integer Precision
Integers (numbers without a period or exponent notation) are accurate up to 15 digits.
Example
let x = 999999999999999; // x will be 999999999999999 let y = 9999999999999999; // y will be 10000000000000000
The maximum number of decimals is 17.
Floating Precision
Floating point arithmetic is not always 100% accurate:
let x = 0.2 + 0.1;
To solve the problem above, it helps to multiply and divide:
let x = (0.2 * 10 + 0.1 * 10) / 10;
Adding Numbers and Strings
WARNING !!
JavaScript uses the + operator for both addition and concatenation.
Numbers are added. Strings are concatenated.
If you add two numbers, the result will be a number:
Example
let x = 10; let y = 20; let z = x + y;
If you add two strings, the result will be a string concatenation:
Example
let x = "10"; let y = "20"; let z = x + y;
If you add a number and a string, the result will be a string concatenation:
Example
let x = 10; let y = "20"; let z = x + y;
If you add a string and a number, the result will be a string concatenation:
Example
let x = "10"; let y = 20; let z = x + y;
A common mistake is to expect this result to be 30:
Example
let x = 10; let y = 20; let z = "The result is: " + x + y;
A common mistake is to expect this result to be 102030:
Example
let x = 10; let y = 20; let z = "30"; let result = x + y + z;
The JavaScript interpreter works from left to right.
First 10 + 20 is added because x and y are both numbers.
Then 30 + "30" is concatenated because z is a string.
Numeric Strings
JavaScript strings can have numeric content:
let x = 100; // x is a number
let y = "100"; // y is a string
JavaScript will try to convert strings to numbers in all numeric operations:
This will work:
let x = "100"; let y = "10"; let z = x / y;
This will also work:
let x = "100"; let y = "10"; let z = x * y;
And this will work:
let x = "100"; let y = "10"; let z = x - y;
But this will not work:
let x = "100"; let y = "10"; let z = x + y;
In the last example JavaScript uses the + operator to concatenate the strings.
NaN - Not a Number
NaN is a JavaScript reserved word indicating that a n
JavaScript BigInt variables are used to store big integer values that are too big to be represented by a normal JavaScript Number.
JavaScript Integer Accuracy
JavaScript integers are only accurate up to 15 digits:
Integer Precision
let x = 999999999999999; let y = 9999999999999999;
In JavaScript, all numbers are stored in a 64-bit floating-point format (IEEE 754 standard).
With this standard, large integer cannot be exactly represented and will be rounded.
Because of this, JavaScript can only safely represent integers:
Up to 9007199254740991 +(253-1)
and
Down to -9007199254740991 -(253-1).
Integer values outside this range lose precision.
How to Create a BigInt
To create a BigInt, append n to the end of an integer or call BigInt():
Examples
let x = 9999999999999999; let y = 9999999999999999n;
let x = 1234567890123456789012345n; let y = BigInt(1234567890123456789012345)
BigInt: A new JavaScript Datatype
The JavaScript typeof a BigInt is "bigint":
Example
let x = BigInt(999999999999999); let type = typeof x;
BigInt is the second numeric data type in JavaScript (after Number).
With BigInt the total number of supported data types in JavaScript is 8:
1. String 2. Number 3. Bigint 4. Boolean 5. Undefined 6. Null 7. Symbol 8. Object
BigInt Operators
Operators that can be used on a JavaScript Number can also be used on a BigInt.
BigInt Multiplication Example
let x = 9007199254740995n; let y = 9007199254740995n; let z = x * y;
Notes
Arithmetic between a BigInt and a Number is not allowed (type conversion lose information).
Unsigned right shift (>>>) can not be done on a BigInt (it does not have a fixed width).
BigInt Decimals
A BigInt can not have decimals.
BigInt Division Example
let x = 5n; let y = x / 2; // Error: Cannot mix BigInt and other types, use explicit conversion.
let x = 5n; let y = Number(x) / 2;
BigInt Hex, Octal and Binary
BigInt can also be written in hexadecimal, octal, or binary notation:
BigInt Hex Example
let hex = 0x20000000000003n; let oct = 0o400000000000000003n; let bin = 0b100000000000000000000000000000000000000000000000000011n;
Precision Curiosity
Rounding can compromise program security:
MAX_SAFE_INTEGER Example
9007199254740992 === 9007199254740993; // is true !!!
Browser Support
BigInt is supported in all browsers since September 2020:
|
|
|
|
|
Chrome 67
|
Edge 79
|
Firefox 68
|
Safari 14
|
Opera 54
|
May 2018
|
Jan 2020
|
Jul 2019
|
Sep 2020
|
Jun 2018
|
Minimum and Maximum Safe Integers
ES6 added max and min properties to the Number object:
- MAX_SAFE_INTEGER
- MIN_SAFE_INTEGER
MAX_SAFE_INTEGER Example
let x = Number.MAX_SAFE_INTEGER;
MIN_SAFE_INTEGER Example
let x = Number.MIN_SAFE_INTEGER;
New Number Methods
ES6 also added 2 new methods to the Number object:
- Number.isInteger()
- Number.isSafeInteger()
The Number.isInteger() Method
The Number.isInteger() method returns true if the argument is an integer.
Example: isInteger()
Number.isInteger(10); Number.isInteger(10.5);
The Number.isSafeInteger() Method
A safe integer is an integer that can be exactly represented as a double precision number.
The Number.isSafeInteger() method returns true if the argument is a safe integer.
Example isSafeInteger()
Number.isSafeInteger(10); Number.isSafeInteger(12345678901234567890);
Safe integers are all integers from -(253 - 1) to +(253 - 1). This is safe: 9007199254740991. This is not safe: 9007199254740992.
JavaScript Number Methods
These number methods can be used on all JavaScript numbers:
Method
|
Description
|
toString()
|
Returns a number as a string
|
toExponential()
|
Returns a number written in exponential notation
|
toFixed()
|
Returns a number written with a number of decimals
|
toPrecision()
|
Returns a number written with a specified length
|
valueOf()
|
Returns a number as a number
|
The toString() Method
The toString() method returns a number as a string.
All number methods can be used on any type of numbers (literals, variables, or expressions):
Example
let x = 123; x.toString(); (123).toString(); (100 + 23).toString();
The toExponential() Method
toExponential() returns a string, with a number rounded and written using exponential notation.
A parameter defines the number of characters behind the decimal point:
Example
let x = 9.656; x.toExponential(2); x.toExponential(4); x.toExponential(6);
The parameter is optional. If you don't specify it, JavaScript will not round the number.
The toFixed() Method
toFixed() returns a string, with the number written with a specified number of decimals:
Example
let x = 9.656; x.toFixed(0); x.toFixed(2); x.toFixed(4); x.toFixed(6);
toFixed(2) is perfect for working with money.
The toPrecision() Method
toPrecision() returns a string, with a number written with a specified length:
Example
let x = 9.656; x.toPrecision(); x.toPrecision(2); x.toPrecision(4); x.toPrecision(6);
The valueOf() Method
valueOf() returns a number as a number.
Example
let x = 123; x.valueOf(); (123).valueOf(); (100 + 23).valueOf();
In JavaScript, a number can be a primitive value (typeof = number) or an object (typeof = object).
The valueOf() method is used internally in JavaScript to convert Number objects to primitive values.
There is no reason to use it in your code.
All JavaScript data types have a valueOf() and a toString() method.
Converting Variables to Numbers
There are 3 JavaScript methods that can be used to convert a variable to a number:
Method
|
Description
|
Number()
|
Returns a number converted from its argument.
|
parseFloat()
|
Parses its argument and returns a floating point number
|
parseInt()
|
Parses its argument and returns a whole number
|
The methods above are not number methods. They are global JavaScript methods.
The Number() Method
The Number() method can be used to convert JavaScript variables to numbers:
Example
Number(true); Number(false); Number("10"); Number(" 10"); Number("10 "); Number(" 10 "); Number("10.33"); Number("10,33"); Number("10 33"); Number("John");
If the number cannot be converted, NaN (Not a Number) is returned.
The Number() Method Used on Dates
Number() can also convert a date to a number.
Example
Number(new Date("1970-01-01"))
Note
The Date() method returns the number of milliseconds since 1.1.1970.
The number of milliseconds between 1970-01-02 and 1970-01-01 is 86400000:
Example
Number(new Date("1970-01-02"))
Example
Number(new Date("2017-09-30"))
The parseInt() Method
parseInt() parses a string and returns a whole number. Spaces are allowed. Only the first number is returned:
Example
parseInt("-10"); parseInt("-10.33"); parseInt("10"); parseInt("10.33"); parseInt("10 20 30"); parseInt("10 years"); parseInt("years 10");
If the number cannot be converted, NaN (Not a Number) is returned.
The parseFloat() Method
parseFloat() parses a string and returns a number. Spaces are allowed. Only the first number is returned:
Example
parseFloat("10"
Property
|
Description
|
EPSILON
|
The difference between 1 and the smallest number > 1.
|
MAX_VALUE
|
The largest number possible in JavaScript
|
MIN_VALUE
|
The smallest number possible in JavaScript
|
MAX_SAFE_INTEGER
|
The maximum safe integer (253 - 1)
|
MIN_SAFE_INTEGER
|
The minimum safe integer -(253 - 1)
|
POSITIVE_INFINITY
|
Infinity (returned on overflow)
|
NEGATIVE_INFINITY
|
Negative infinity (returned on overflow)
|
NaN
|
A "Not-a-Number" value
|
JavaScript EPSILON
Number.EPSILON is the difference between the smallest floating point number greater than 1 and 1.
Example
let x = Number.EPSILON;
Note
Number.EPSILON is an ES6 feature.
It does not work in Internet Explorer.
JavaScript MAX_VALUE
Number.MAX_VALUE is a constant representing the largest possible number in JavaScript.
Example
let x = Number.MAX_VALUE;
Number Properties Cannot be Used on Variables
Number properties belong to the JavaScript Number Object.
These properties can only be accessed as Number.MAX_VALUE.
Using x.MAX_VALUE, where x is a variable or a value, will return undefined:
Example
let x = 6; x.MAX_VALUE
JavaScript MIN_VALUE
Number.MIN_VALUE is a constant representing the lowest possible number in JavaScript.
Example
let x = Number.MIN_VALUE;
JavaScript MAX_SAFE_INTEGER
Number.MAX_SAFE_INTEGER represents the maximum safe integer in JavaScript.
Number.MAX_SAFE_INTEGER is (253 - 1).
Example
let x = Number.MAX_SAFE_INTEGER;
JavaScript MIN_SAFE_INTEGER
Number.MIN_SAFE_INTEGER represents the minimum safe integer in JavaScript.
Number.MIN_SAFE_INTEGER is -(253 - 1).
Example
let x = Number.MIN_SAFE_INTEGER;
Note
MAX_SAFE_INTEGER and MIN_SAFE_INTEGER are ES6 features.
They do not work in Internet Explorer.
JavaScript POSITIVE_INFINITY
Example
let x = Number.POSITIVE_INFINITY;
POSITIVE_INFINITY is returned on overflow:
let x = 1 / 0;
JavaScript NEGATIVE_INFINITY
Example
let x = Number.NEGATIVE_INFINITY;
NEGATIVE_INFINITY is returned on overflow:
let x = -1 / 0;
JavaScript NaN - Not a Number
NaN is a JavaScript reserved word for a number that is not a legal number.
Examples
let x = Number.NaN;
Trying to do arithmetic with a non-numeric string will result in NaN (Not a Number):
let x = 100 / "Apple";
Complete JavaScript Number Reference
For a complete Number reference, visit our:
Complete JavaScript Number Reference.
The reference contains descriptions and examples of all Number properties and methods.
An array is a special variable, which can hold more than one value:
const cars = ["Saab", "Volvo", "BMW"];
Why Use Arrays?
If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this:
let car1 = "Saab"; let car2 = "Volvo"; let car3 = "BMW";
However, what if you want to loop through the cars and find a specific one? And what if you had not 3 cars, but 300?
The solution is an array!
An array can hold many values under a single name, and you can access the values by referring to an index number.
Creating an Array
Using an array literal is the easiest way to create a JavaScript Array.
Syntax:
const array_name = [item1, item2, ...];
It is a common practice to declare arrays with the const keyword.
Learn more about const with arrays in the chapter: JS Array Const.
Example
const cars = ["Saab", "Volvo", "BMW"];
Spaces and line breaks are not important. A declaration can span multiple lines:
Example
const cars = [ "Saab", "Volvo", "BMW" ];
You can also create an array, and then provide the elements:
Example
const cars = []; cars[0]= "Saab"; cars[1]= "Volvo"; cars[2]= "BMW";
Using the JavaScript Keyword new
The following example also creates an Array, and assigns values to it:
Example
const cars = new Array("Saab", "Volvo", "BMW");
The two examples above do exactly the same.
There is no need to use new Array().
For simplicity, readability and execution speed, use the array literal method.
Accessing Array Elements
You access an array element by referring to the index number:
const cars = ["Saab", "Volvo", "BMW"]; let car = cars[0];
Note: Array indexes start with 0.
[0] is the first element. [1] is the second element.
Changing an Array Element
This statement changes the value of the first element in cars:
cars[0] = "Opel";
Example
const cars = ["Saab", "Volvo", "BMW"]; cars[0] = "Opel";
Converting an Array to a String
The JavaScript method toString() converts an array to a string of (comma separated) array values.
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"]; document.getElementById("demo").innerHTML = fruits.toString();
Result:
Banana,Orange,Apple,Mango
Access the Full Array
With JavaScript, the full array can be accessed by referring to the array name:
Example
const cars = ["Saab", "Volvo", "BMW"]; document.getElementById("demo").innerHTML = cars;
Arrays are Objects
Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays.
But, JavaScript arrays are best described as arrays.
Arrays use numbers to access its "elements". In this example, person[0] returns John:
Array:
const person = ["John", "Doe", 46];
Objects use names to access its "members". In this example, person.firstName returns John:
Object:
const person = {firstName:"John", lastName:"Doe", age:46};
Array Elements Can Be Objects
JavaScript variables can be objects. Arrays are special kinds of objects.
Because of this, you can have variables of different types in the same Array.
You can have objects in an Array. You can have functions in an Array. You can have arrays in an Array:
myArray[0] = Date.now; myArray[1] = myFunction; myArray[2] = myCars;
Array Properties and Methods
The real strength of JavaScript arrays are the built-in array properties and methods:
cars.length // Returns the number of elements cars.sort() // Sorts the array
Array methods are covered in the next chapters.
The length Property
The length property of an array returns the length of an array (the number of array elements).
Example
const fruits = [
Array length
Array toString()
Array pop()
Array push()
Array shift()
Array unshift()
|
Array join()
Array delete()
Array concat()
Array flat()
Array splice()
Array slice()
|
The methods are listed in the order they appear in this tutorial page |
JavaScript Array length
The length property returns the length (size) of an array:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
let size = fruits.length;
JavaScript Array toString()
The JavaScript method toString() converts an array to a
string of (comma separated) array values.
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
document.getElementById("demo").innerHTML = fruits.toString();
Result:
Banana,Orange,Apple,Mango
The join() method also joins all array elements into a string.
It behaves just like toString() , but in addition you can specify the separator:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
document.getElementById("demo").innerHTML = fruits.join(" * ");
Result:
Banana * Orange * Apple * Mango
Popping and Pushing
When you work with arrays, it is easy to remove elements and add
new elements.
This is what popping and pushing is:
Popping items out of an array, or pushing
items into an array.
JavaScript Array pop()
The pop() method removes the last element from an array:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.pop();
The pop() method returns the value that was "popped out":
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
let fruit = fruits.pop();
JavaScript Array push()
The push() method adds a new element to an array (at the end):
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.push("Kiwi");
The push() method returns the new array length:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
let length = fruits.push("Kiwi");
Shifting Elements
Shifting is equivalent to popping, but working on the first element instead of
the last.
JavaScript Array shift()
The shift() method removes the first array element and "shifts" all
other elements to a lower index.
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.shift();
The shift() method returns the value that was "shifted out":
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
let fruit = fruits.shift();
JavaScript Array unshift()
The unshift() method adds a new element to an array (at the beginning), and "unshifts"
older elements:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.unshift("Lemon");
The unshift() method returns the new array length:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.unshift("Lemon");
Changing Elements
Array elements are accessed using their index number:
Array indexes start with 0:
[0] is the first array element [1] is the second [2] is the third ...
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits[0] = "Kiwi";
JavaScript Array length
The length property provides an easy way to append a new element to an array:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits[fruits.length] = "Kiwi";
JavaScript Array delete()
Warning !
Array elements can be deleted using the JavaScript operator delete .
Using delete leaves undefined holes in the
array.
Use pop() or shift() instead.
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
delete fruits[0];
Merging (Concatenating) Arrays
The concat() method creates a new array by merging (concatenating)
existing arrays:
Example (Merging Two Arrays)
const myGirls = ["Cecilie", "Lone"];
const myBoys = ["Emil", "Tobias", "Linus"];
const myChildren = myGirls.concat(myBoys);
The concat() method does not change the existing arrays. It always returns a new array.
The concat() method can take any number of array arguments:
Example (Merging Three Arrays)
const arr1 = ["Cecilie", "Lone"];
const arr2 = ["Emil", "Tobias", "Linus"];
const arr3 = ["Robin", "Morgan"];
const myChildren = arr1.concat(arr2, arr3);
The concat() method can also take strings as arguments:
Example (Merging an Array with Values)
const arr1 = ["Emil", "Tobias", "Linus"];
const myChildren = arr1.concat("Peter");Â
Flattening an Array
Flattening an array is the process of reducing the dimensionality of an array.
The flat() method creates a new array with sub-array elements concatenated to a specified depth.
Example
const myArr = [[1,2],[3,4],[5,6]];
const newArr = myArr.flat();
Browser Support
JavaScript Array flat() is supported in all modern browsers since January 2020:
|
|
|
|
|
Chrome 69 |
Edge 79 |
Firefox 62 |
Safari 12 |
Opera 56 |
Sep 2018 |
Jan 2020 |
Sep 2018 |
Sep 2018 |
Sep 2018 |
Splicing and Slicing Arrays
The splice() method adds new items to an array.
The slice() method slices out a piece of an array.
JavaScript Array splice()
The splice() method can be used to add new items to an array:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.splice(2, 0, "Lemon", "Kiwi");
The first parameter (2) defines the position where new elements should be
added (spliced in).
The second parameter (0) defines how many elements should be
removed.
The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be
added.
The splice() method returns an array with the deleted items:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.splice(2, 2, "Lemon", "Kiwi");
Using splice() to Remove Elements
With clever parameter setting, you can use splice() to remove elements without leaving
"holes" in the array:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.splice(0, 1);
The first parameter (0) defines the position where new elements should be
added (spliced in).
The second parameter (1) defines how many elements should be
removed.
The rest of the parameters are omitted. No new elements will be added.
JavaScript Array slice()
The slice() method slices out a piece of an array into a new
array.
This example slices out a part of an array starting from array element 1
("Orange"):
Example
const fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"];
const citrus = fruits.slice(1);
Note
The slice() method creates a new array.
The slice() method does not remove any elements from the source array.
This example slices out a part of an array starting from array element 3
("Apple"):
Example
const fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"];
const citrus = fruits.slice(3);
The slice() method can take two arguments like slice(1, 3) .
The method then selects elements from the start argument, and up to (but not
including) the end argument.
Example
const fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"];
const citrus = fruits.slice(1, 3);
If the end argument is omitted, like in the first examples, the slice()
method slices out the rest of the array.
Example
const fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"];
const citrus = fruits.slice(2);
Automatic toString()
JavaScript automatically converts an array to a comma separated string when a
primitive value is expected.
This is always the case when you try to output an array.
These two examples will produce the same result:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
document.getElementById("demo").innerHTML = fruits.toString();
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
document.getElementById("demo").innerHTML = fruits;
Note
All JavaScript objects have a toString() method.
Finding Max and Min Values in an Array
There are no built-in functions for finding the highest
or lowest value in a JavaScript array.
You will learn how you solve this problem in the next
chapter of this tutorial.
Sorting Arrays
Sorting arrays are covered in the next chapter of this tutorial.
Complete Array Reference
For a complete Array reference, go to our:
.
The reference contains descriptions and examples of all Array
properties and methods.
JavaScript Sorting Arrays
Sorting an Array
The sort() method sorts an array alphabetically:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.sort();
Reversing an Array
The reverse() method reverses the elements in an array.
You can use it to
sort an array in descending order:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.sort();
fruits.reverse();
Numeric Sort
By default, the sort() function sorts values as strings.
This works well for strings ("Apple" comes before "Banana").
However, if numbers are sorted as strings, "25" is bigger than "100",
because "2" is bigger than "1".
Because of this, the sort() method will produce incorrect result when sorting
numbers.
You can fix this by providing a compare function:
Example
const points = [40, 100, 1, 5, 25, 10];
points.sort(function(a, b){return a - b});
Use the same trick to sort an array descending:
Example
const points = [40, 100, 1, 5, 25, 10];
points.sort(function(a, b){return b - a});
The Compare Function
The purpose of the compare function is to define an alternative sort
order.
The compare function should return a negative, zero, or positive value, depending on
the arguments:
function(a, b){return a - b}
When the sort() function compares two values, it sends the values to the
compare function, and sorts the values according to the returned (negative,
zero, positive) value.
If the result is negative, a is sorted before
b .
If the result is positive, b is sorted
before a .
If the result is 0, no changes are done with the sort order of the two
values.
Example:
The compare function compares all the values in the array, two values at a
time (a, b) .
When comparing 40 and 100, the sort() method calls the compare function(40, 100).
The function calculates 40 - 100 (a - b) , and
since the result is negative (-60), the sort function will sort 40 as a value lower than 100.
You can use this code snippet to experiment with numerically and
alphabetically sorting:
<button onclick="myFunction1()">Sort Alphabetically</button> <button
onclick="myFunction2()">Sort Numerically</button>
<p id="demo"></p>
<script> const points = [40, 100, 1, 5, 25, 10];
document.getElementById("demo").innerHTML = points;
function
myFunction1() { Â Â points.sort(); Â Â document.getElementById("demo").innerHTML
= points; }
function myFunction2() { Â points.sort(function(a, b){return
a - b}); Â Â document.getElementById("demo").innerHTML = points; }
</script>
Sorting an Array in Random Order
Example
const points = [40, 100, 1, 5, 25, 10];
points.sort(function(){return 0.5 - Math.random()});
The Fisher Yates Method
The above example, array.sort(), is not accurate. It will favor some
numbers over the others.
The most popular correct method, is called the Fisher Yates shuffle, and was
introduced in data science as early as 1938!
In JavaScript the method can be translated to this:
Example
const points = [40, 100, 1, 5, 25, 10];
for (let i = points.length -1; i > 0; i--) {
 let j = Math.floor(Math.random() * (i+1));
 let k = points[i]; Â
points[i] = points[j];
 points[j] = k; }
Find the Highest (or Lowest) Array Value
There are no built-in functions for finding the max or min
value in an array.
However, after you have sorted an array, you can use the
index to obtain the highest and lowest values.
Sorting ascending:
Example
const points = [40, 100, 1, 5, 25, 10];
points.sort(function(a, b){return a - b});
// now points[0] contains the lowest value
// and points[points.length-1] contains the highest value
Sorting descending:
Example
const points = [40, 100, 1, 5, 25, 10];
points.sort(function(a, b){return b - a});
// now points[0] contains the highest value
// and points[points.length-1] contains the lowest value
Sorting a whole array is a very inefficient method if you only want to find the highest (or lowest) value.
Using Math.max() on an Array
You can use Math.max.apply to find the highest number in an array:
Example
function myArrayMax(arr) {
 return Math.max.apply(null, arr); }
Math.max.apply(null, [1, 2, 3]) is equivalent to Math.max(1, 2, 3) .
Using Math.min() on an Array
You can use Math.min.apply to find the lowest number in an array:
Example
function myArrayMin(arr) {
  return Math.min.apply(null, arr); }
Math.min.apply(null, [1, 2, 3]) is equivalent to Math.min(1, 2, 3) .
My Min / Max JavaScript Methods
The fastest solution is to use a "home made" method.
This function loops through an array comparing each value with the highest
value found:
Example (Find Max)
function myArrayMax(arr) {
  let len = arr.length;
  let max = -Infinity;
  while (len--) {
  Â
if (arr[len] > max) { Â Â Â Â Â
max = arr[len]; Â Â Â Â } Â Â } Â return max; }
This function loops through an array comparing each value with the lowest
value found:
Example (Find Min)
function myArrayMin(arr) { Â Â let len = arr.length; Â Â let min = Infinity; Â Â while (len--) { Â Â Â
if (arr[len] < min) { Â Â Â Â Â
min = arr[len]; Â Â Â Â } Â Â } Â Â return min; }
Sorting Object Arrays
JavaScript arrays often contain objects:
Example
const cars = [
Â
{type:"Volvo", year:2016},
Â
{type:"Saab", year:2001},
Â
{type:"BMW", year:2010} ];
Even if objects have properties of different data types, the sort() method
can be used to sort the array.
The solution is to write a compare function to compare the property values:
Example
cars.sort(function(a, b){return a.year - b.year});
Comparing string properties is a little more complex:
Example
cars.sort(function(a, b){
  let x = a.type.toLowerCase();
  let y = b.type.toLowerCase();
 if (x < y) {return -1;} Â
if (x > y) {return 1;} Â return 0; });
Stable Array sort()
revised the Array sort() method.
Before 2019, the specification allowed unstable sorting algorithms such as QuickSort.
After ES2019, browsers must use a stable sorting algorithm:
When sorting elements on a value, the elements must keep their relative position to other elements with the same value.
Example
const myArr = [
 {name:"X00",price:100 },
 {name:"X01",price:100 },
 {name:"X02",price:100 },
 {name:"X03",price:100 },
 {name:"X04",price:110 },
 {name:"X05",price:110 },
 {name:"X06",price:110 },
 {name:"X07",price:110 }
];
In the example above, when sorting on price, the result is not allowed to come out with the names
in an other relative position like this:
X01 100
X03 100
X00 100
X03 100
X05 110
X04 110
X06 110
X07 110
Complete Array Reference
For a complete Array reference, go to our:
.
The reference contains descriptions and examples of all Array
properties and methods.
Array iteration methods operate on every array item.
JavaScript Array forEach()
The forEach() method calls a function (a callback function) once for each array element.
Example
const numbers = [45, 4, 9, 16, 25];
let txt = "";
numbers.forEach(myFunction);
function myFunction(value, index, array) {
Â
txt += value + "<br>";
}
Note that the function takes 3 arguments:
- The item value
- The item index
- The array itself
The example above uses only the value parameter. The example can be rewritten
to:
Example
const numbers = [45, 4, 9, 16, 25];
let txt = "";
numbers.forEach(myFunction);
function myFunction(value) {
Â
txt += value + "<br>";
}
JavaScript Array map()
The map() method creates a new array by performing a function on each array element.
The map() method does not execute the function for array
elements without values.
The map() method does not change the original array.
This example multiplies each array value by 2:
Example
const numbers1 = [45, 4, 9, 16, 25];
const numbers2 = numbers1.map(myFunction);
function myFunction(value, index, array) {
 return value * 2;
}
Note that the function takes 3 arguments:
- The item value
- The item index
- The array itself
When a callback function uses only the value parameter, the index and array
parameters can be omitted:
Example
const numbers1 = [45, 4, 9, 16, 25];
const numbers2 = numbers1.map(myFunction);
function myFunction(value) {
 return value * 2;
}
JavaScript Array flatMap()
added the Array flatMap() method to JavaScript.
The flatMap() method first maps all elements of an array
and then creates a new array by flattening the array.
Example
const myArr = [1, 2, 3, 4, 5, 6];
const newArr = myArr.flatMap((x) => x * 2);
Browser Support
JavaScript Array flatMap() is supported in all modern browsers since January 2020:
|
|
|
|
|
Chrome 69 |
Edge 79 |
Firefox 62 |
Safari 12 |
Opera 56 |
Sep 2018 |
Jan 2020 |
Sep 2018 |
Sep 2018 |
Sep 2018 |
JavaScript Array filter()
The filter() method creates a new array with array elements that pass a test.
This example creates a new array from elements with a value larger than 18:
Example
const numbers = [45, 4, 9, 16, 25];
const over18 = numbers.filter(myFunction);
function myFunction(value, index, array) { Â Â return value > 18; }
Note that the function takes 3 arguments:
- The item value
- The item index
- The array itself
In the example above, the callback function does not use the index and array
parameters, so they can be omitted:
Example
const numbers = [45, 4, 9, 16, 25];
const over18 =
numbers.filter(myFunction);
function myFunction(value) { Â Â return value > 18; }
JavaScript Array reduce()
The reduce() method runs a function on each array element to produce (reduce it to) a single value.
The reduce() method works from left-to-right in the array. See also reduceRight() .
The reduce() method does not reduce the original array.
This example finds the sum of all numbers in an array:
Example
const numbers = [45, 4, 9, 16, 25];
let sum = numbers.reduce(myFunction);
function myFunction(total, value, index, array) { Â
return total + value; }
Note that the function takes 4 arguments:
- The total (the initial value / previously returned value)
- The item value
- The item index
- The array itself
The example above does not use the index and array parameters. It can be
rewritten to:
Example
const numbers = [45, 4, 9, 16, 25];
let sum = numbers.reduce(myFunction);
function myFunction(total, value) { Â
return total + value; }
The reduce() method can accept an initial value:
Example
const numbers = [45, 4, 9, 16, 25];
let sum = numbers.reduce(myFunction,
100);
function myFunction(total, value) { Â Â return total + value; }
JavaScript Array reduceRight()
The reduceRight() method runs a function on each array element to produce (reduce it to) a single value.
The reduceRight() works from right-to-left in the array. See also reduce() .
The reduceRight() method does not reduce the original array.
This example finds the sum of all numbers in an array:
Example
const numbers = [45, 4, 9, 16, 25];
let sum = numbers.reduceRight(myFunction);
function myFunction(total, value, index, array) { Â
return total + value; }
Note that the function takes 4 arguments:
- The total (the initial value / previously returned value)
- The item value
- The item index
- The array itself
The example above does not use the index and array parameters. It can be
rewritten to:
Example
const numbers = [45, 4, 9, 16, 25];
let sum = numbers.reduceRight(myFunction);
function myFunction(total, value) { Â Â return total + value; }
JavaScript Array every()
The every() method checks if all array values pass a test.
This example checks if all array values are larger than 18:
Example
const numbers = [45, 4, 9, 16, 25];
let allOver18 =
numbers.every(myFunction);
function myFunction(value, index, array) {
 return
value > 18; }
Note that the function takes 3 arguments:
- The item value
- The item index
- The array itself
When a callback function uses the first parameter only (value), the other
parameters can be omitted:
Example
const numbers = [45, 4, 9, 16, 25];
let allOver18 =
numbers.every(myFunction);
function myFunction(value) { Â return
value > 18; }
JavaScript Array some()
The some() method checks if some array values pass a test.
This example checks if some array values are larger than 18:
Example
const numbers = [45, 4, 9, 16, 25];
let someOver18 = numbers.some(myFunction);
function myFunction(value, index, array) {
 return
value > 18; }
Note that the function takes 3 arguments:
- The item value
- The item index
- The array itself
JavaScript Array indexOf()
The indexOf() method searches an array for an element value and returns its position.
Note: The first item has position 0, the second item has position 1, and so on.
Example
Search an array for the item "Apple":
const fruits = ["Apple", "Orange", "Apple", "Mango"];
let position = fruits.indexOf("Apple") + 1;
Syntax
array.indexOf(item, start)
item |
Required. The item to search for. |
start |
Optional. Where to start the search. Negative values will start at the given position counting from the end, and search to the end. |
Array.indexOf() returns -1 if the item is not found.
If the item is present more than once, it returns the position of the first
occurrence.
JavaScript Array lastIndexOf()
Array.lastIndexOf() is the same as Array.indexOf() , but
returns the position of the last occurrence of the specified element.
Example
Search an array for the item "Apple":
const fruits = ["Apple", "Orange", "Apple", "Mango"];
let position = fruits.lastIndexOf("Apple") + 1;
Syntax
array.lastIndexOf(item, start)
item |
Required. The item to search for |
start |
Optional. Where to start the search. Negative values will start at the given position counting from the end, and search to the beginning |
JavaScript Array find()
The find() method returns the value of the first array element that passes a
test function.
This example finds (returns the value of) the first element that is larger
than 18:
Example
const numbers = [4, 9, 16, 25, 29];
let first =
numbers.find(myFunction);
function myFunction(value, index, array) { Â Â return
value > 18; }
Note that the function takes 3 arguments:
- The item value
- The item index
- The array itself
Browser Support
find() is an (JavaScript 2015).
It is supported in all modern browsers:
|
|
|
|
|
Chrome |
Edge |
Firefox |
Safari |
Opera |
Yes |
Yes |
Yes |
Yes |
Yes |
find() is not supported in Internet Explorer.
JavaScript Array findIndex()
The findIndex() method returns the index of the first array element that
passes a test function.
This example finds the index of the first element that is larger than 18:
Example
const numbers = [4, 9, 16, 25, 29];
let first =
numbers.findIndex(myFunction);
function myFunction(value, index, array) {
 return
value > 18; }
Note that the function takes 3 arguments:
- The item value
- The item index
- The array itself
Browser Support
findIndex() is an (JavaScript 2015).
It is supported in all modern browsers:
|
|
|
|
|
Chrome |
Edge |
Firefox |
Safari |
Opera |
Yes |
Yes |
Yes |
Yes |
Yes |
findIndex() is not supported in Internet Explorer.
JavaScript Array.from()
The Array.from() method returns an Array object from any object with a length
property or any iterable object.
Example
Create an Array from a String:
Array.from("ABCDEFG");
Browser Support
from() is an (JavaScript 2015).
It is supported in all modern browsers:
|
|
|
|
|
Chrome |
Edge |
Firefox |
Safari |
Opera |
Yes |
Yes |
Yes |
Yes |
Yes |
from() is not supported in Internet Explorer.
JavaScript Array Keys()
The Array.keys() method returns an Array Iterator object with the keys of an array.
Example
Create an Array Iterator object, containing the keys of the array:
const fruits = ["Banana", "Orange", "Apple", "Mango"];
const keys = fruits.keys();
for (let x of keys) {
 text += x + "<br>"; }
Browser Support
keys() is an (JavaScript 2015).
It is supported in all modern browsers:
|
|
|
|
|
Chrome |
Edge |
Firefox |
Safari |
Opera |
Yes |
Yes |
Yes |
Yes |
Yes |
keys() is not supported in Internet Explorer.
JavaScript Array entries()
Example
Create an Array Iterator, and then iterate over the key/value pairs:
const fruits = ["Banana", "Orange", "Apple", "Mango"];
const f = fruits.entries();
for (let x of f) {
 document.getElementById("demo").innerHTML += x; }
The entries() method returns an Array Iterator object with key/value pairs:
[0, "Banana"] [1, "Orange"] [2, "Apple"] [3, "Mango"]
The entries() method does not change the original array.
Browser Support
entries() is an (JavaScript 2015).
It is supported in all modern browsers:
|
|
|
|
|
Chrome |
Edge |
Firefox |
Safari |
Opera |
Yes |
Yes |
Yes |
Yes |
Yes |
entries() is not supported in Internet Explorer.
JavaScript Array includes()
ECMAScript 2016 introduced Array.includes() to arrays.
This allows us to check if an element is present in an array (including NaN, unlike indexOf).
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.includes("Mango"); // is true
Syntax
array.includes(search-item)
Array.includes() allows to check for NaN values. Unlike Array.indexOf().
Browser Support
includes() is an feature.
It is supported in all modern browsers:
|
|
|
|
|
Chrome |
Edge |
Firefox |
Safari |
Opera |
Yes |
Yes |
Yes |
Yes |
Yes |
includes() is not supported in Internet Explorer.
JavaScript Array Spread (...)
The ... operator expands an iterable (like an array) into more elements:
Example
const q1 = ["Jan", "Feb", "Mar"];
const q2 = ["Apr", "May", "Jun"];
const q3 = ["Jul", "Aug", "Sep"];
const q4 = ["Oct", "Nov", "May"];
const year = [...q1, ...q2, ...q3, ...q4];
Browser Support
... is an (JavaScript 2015).
It is supported in all modern browsers:
|
|
|
|
|
Chrome |
Edge |
Firefox |
Safari |
Opera |
Yes |
Yes |
Yes |
Yes |
Yes |
... is not supported in Internet Explorer.
Complete Array Reference
For a complete Array reference, go to our:
.
The reference contains descriptions and examples of all Array
properties and methods.
ECMAScript 2015 (ES6)
In 2015, JavaScript introduced an important new keyword: const.
It has become a common practice to declare arrays using const:
Example
const cars = ["Saab", "Volvo", "BMW"];
Cannot be Reassigned
An array declared with const cannot be reassigned:
Example
const cars = ["Saab", "Volvo", "BMW"]; cars = ["Toyota", "Volvo", "Audi"]; // ERROR
Arrays are Not Constants
The keyword const is a little misleading.
It does NOT define a constant array. It defines a constant reference to an array.
Because of this, we can still change the elements of a constant array.
Elements Can be Reassigned
You can change the elements of a constant array:
Example
// You can create a constant array: const cars = ["Saab", "Volvo", "BMW"];
// You can change an element: cars[0] = "Toyota";
// You can add an element: cars.push("Audi");
Browser Support
The const keyword is not supported in Internet Explorer 10 or earlier.
The following table defines the first browser versions with full support for the const keyword:
|
|
|
|
|
Chrome 49
|
IE 11 / Edge
|
Firefox 36
|
Safari 10
|
Opera 36
|
Mar, 2016
|
Oct, 2013
|
Feb, 2015
|
Sep, 2016
|
Mar, 2016
|
Assigned when Declared
JavaScript const variables must be assigned a value when they are declared:
Meaning: An array declared with const must be initialized when it is declared.
Using const without initializing the array is a syntax error:
Example
This will not work:
const cars; cars = ["Saab", "Volvo", "BMW"];
Arrays declared with var can be initialized at any time.
You can even use the array before it is declared:
Example
This is OK:
cars = ["Saab", "Volvo", "BMW"]; var cars;
Const Block Scope
An array declared with const has Block Scope.
An array declared in a block is not the same as an array declared outside the block:
Example
const cars = ["Saab", "Volvo", "BMW"]; // Here cars[0] is "Saab" { const cars = ["Toyota", "Volvo", "BMW"]; // Here cars[0] is "Toyota" } // Here cars[0] is "Saab"
An array declared with var does not have block scope:
Example
var cars = ["Saab", "Volvo", "BMW"]; // Here cars[0] is "Saab" { var cars = ["Toyota", "Volvo", "BMW"]; // Here cars[0] is "Toyota" } // Here cars[0] is "Toyota"
You can learn more about Block Scope in the chapter: JavaScript Scope.
Redeclaring Arrays
Redeclaring an array declared with var is allowed anywhere in a program:
Example
var cars = ["Volvo", "BMW"]; // Allowed var cars = ["Toyota", "BMW"]; // Allowed cars = ["Volvo", "Saab"]; // Allowed
Redeclaring or reassigning an array to const, in the same scope, or in the same block, is not allowed:
Example
var cars = ["Volvo", "BMW"]; // Allowed const cars = ["Volvo", "BMW"];
JavaScript Date Objects let us work with dates:
Examples
const d = new Date();
const d = new Date("2022-03-25");
Note
Date objects are static. The "clock" is not "running".
The computer clock is ticking, date objects are not.
JavaScript Date Output
By default, JavaScript will use the browser's time zone and display a date as a full text string:
You will learn much more about how to display dates, later in this tutorial.
Creating Date Objects
Date objects are created with the
new Date() constructor.
There are 9 ways to create a new date object:
new Date()
new Date(date string)
new Date(year,month)
new Date(year,month,day)
new Date(year,month,day,hours)
new Date(year,month,day,hours,minutes)
new Date(year,month,day,hours,minutes,seconds)
new Date(year,month,day,hours,minutes,seconds,ms)
new Date(milliseconds)
JavaScript new Date()
new Date() creates a date object with the current date and time:
Example
const d = new Date();
new Date(date string)
new Date(date string) creates a date object from a date string:
Examples
const d = new Date("October 13, 2014 11:13:00");
const d = new Date("2022-03-25");
Date string formats are described in the next chapter.
new Date(year, month, ...)
new Date(year, month, ...) creates a date object with a specified date and time.
7 numbers specify year, month, day, hour, minute, second, and millisecond (in that order):
Example
const d = new Date(2018, 11, 24, 10, 33, 30, 0);
Note
JavaScript counts months from 0 to 11:
January = 0.
December = 11.
Specifying a month higher than 11, will not result in an error but add the overflow to the next year:
Specifying:
const d = new Date(2018, 15, 24, 10, 33, 30);
Is the same as:
const d = new Date(2019, 3, 24, 10, 33, 30);
Specifying a day higher than max, will not result in an error but add the overflow to the next month:
Specifying:
const d = new Date(2018, 5, 35, 10, 33, 30);
Is the same as:
const d = new Date(2018, 6, 5, 10, 33, 30);
Using 6, 4, 3, or 2 Numbers
6 numbers specify year, month, day, hour, minute, second:
Example
const d = new Date(2018, 11, 24, 10, 33, 30);
5 numbers specify year, month, day, hour, and minute:
Example
const d = new Date(2018, 11, 24, 10, 33);
4 numbers specify year, month, day, and hour:
Example
const d = new Date(2018, 11, 24, 10);
3 numbers specify year, month, and day:
Example
const d = new Date(2018, 11, 24);
2 numbers specify year and month:
Example
const d = new Date(2018, 11);
You cannot omit month. If you supply only one parameter it will be treated as milliseconds.
Example
const d = new Date(2018);
Previous Century
One and two digit years will be interpreted as 19xx:
Example
const d = new Date(99, 11, 24);
Example
const d = new Date(9, 11, 24);
JavaScript Stores Dates as Milliseconds
JavaScript stores dates as number of milliseconds since January 01, 1970.
Zero time is January 01, 1970 00:00:00 UTC.
One day (24 hours) is 86 400 000 milliseconds.
Now the time is: milliseconds past January 01, 1970
new Date(milliseconds)
new Date(milliseconds) creates a new date object as milliseconds plus zero time:
Examples
01 January 1970 plus 100 000 000 000 milliseconds is:
const d = new Date(100000000000);
January 01 1970 minus 100 000 000 000 milliseconds is:
const d = new Date(-100000000000);
January 01 1970 plus 24 hours is:
const d = new Date(24 * 60 * 60 * 1000);
// or
const d = new Date(86400000);
01 January 1970 plus 0 milliseconds is:
const d = new Date(0);
Date Methods
When a date object is created, a number of methods allow you to operate on
it.
Date methods allow you to get and set the year, month, day, hour,
minute, second, and millisecond of date objects, using either local time or UTC
(universal, or GMT) time.
Date methods and time zones are covered in the next chapters.
Displaying Dates
JavaScript will (by default) output dates using the toString() method.
This is a string representation of the date, including the time zone.
The format is specified in the ECMAScript specification:
When you display a date object in HTML, it is automatically converted to a
string, with the toString() method.
Example
const d = new Date();
d.toString();
The toDateString() method converts a date to a more readable
format:
Example
const d = new Date();
d.toDateString();
The toUTCString() method converts a date to a string using the UTC standard:
Example
const d = new Date();
d.toUTCString();
The toISOString() method converts a date to a string using the ISO standard:
Example
const d = new Date();
d.toISOString();
Complete JavaScript Date Reference
For a complete Date reference, go to our:
.
The reference contains descriptions and examples of all Date properties and
methods.
JavaScript Date Input
There are generally 3 types of JavaScript date input formats:
Type
|
Example
|
ISO Date
|
"2015-03-25" (The International Standard)
|
Short Date
|
"03/25/2015"
|
Long Date
|
"Mar 25 2015" or "25 Mar 2015"
|
The ISO format follows a strict standard in JavaScript.
The other formats are not so well defined and might be browser specific.
JavaScript Date Output
Independent of input format, JavaScript will (by default) output dates in full text string format:
Mon Apr 08 2024 20:56:48 GMT+0530 (India Standard Time)
JavaScript ISO Dates
ISO 8601 is the international standard for the representation of dates and times.
The ISO 8601 syntax (YYYY-MM-DD) is also the preferred JavaScript date format:
Example (Complete date)
const d = new Date("2015-03-25");
The computed date will be relative to your time zone. Depending on your time zone, the result above will vary between March 24 and March 25.
ISO Dates (Year and Month)
ISO dates can be written without specifying the day (YYYY-MM):
Example
const d = new Date("2015-03");
Time zones will vary the result above between February 28 and March 01.
ISO Dates (Only Year)
ISO dates can be written without month and day (YYYY):
Example
const d = new Date("2015");
Time zones will vary the result above between December 31 2014 and January 01 2015.
ISO Dates (Date-Time)
ISO dates can be written with added hours, minutes, and seconds (YYYY-MM-DDTHH:MM:SSZ):
Example
const d = new Date("2015-03-25T12:00:00Z");
Date and time is separated with a capital T.
UTC time is defined with a capital letter Z.
If you want to modify the time relative to UTC, remove the Z and add +HH:MM or -HH:MM instead:
Example
const d = new Date("2015-03-25T12:00:00-06:30");
UTC (Universal Time Coordinated) is the same as GMT (Greenwich Mean Time).
Omitting T or Z in a date-time string can give different results in different browsers.
Time Zones
When setting a date, without specifying the time zone, JavaScript will use the browser's time zone.
When getting a date, without specifying the time zone, the result is converted to the browser's time zone.
In other words: If a date/time is created in GMT (Greenwich Mean Time), the date/time will be converted to CDT (Central US Daylight Time) if a user browses from central US.
JavaScript Short Dates.
Short dates are written with an "MM/DD/YYYY" syntax like this:
Example
const d = new Date("03/25/2015");
WARNINGS !
In some browsers, months or days with no leading zeroes may produce an error:
const d = new Date("2015-3-25");
The behavior of "YYYY/MM/DD" is undefined. Some browsers will try to guess the format. Some will return NaN.
const d = new Date("2015/03/25");
The behavior of "DD-MM-YYYY" is also undefined. Some browsers will try to guess the format. Some will return NaN.
const d = new Date("25-03-2015");
JavaScript Long Dates.
Long dates are most often written with a "MMM DD YYYY" syntax like this:
Example
const d = new Date("Mar 25 2015");
Month and day can be in any order:
Example
const d = new Date("25 Mar 2015");
And, month can be written in full (January), or abbreviated (Jan):
Example
const d = new Date("January 25 2015");
Example
const d = new Date("Jan 25 2015");
Commas are ignored. Names are case insensitive:
Example
const d = new Date("JANUARY, 25, 2015");
Date Input - Parsing Dates
If you have a valid date string, you can use the Date.parse() method to convert it to milliseconds.
Date.parse() returns the number of milliseconds between the date and January 1, 1970:
Example
let msec = Date.parse("March 21, 2012");
You can then use the number of milliseconds to convert it to a date object:
Example
let msec = Date.parse("March 21, 2012"); const d = new Date(msec);
Complete JavaScript Date Reference
For a complete Date reference, go to our:
Complete JavaScript Date Reference.
The reference contains descriptions and examples of all Date properties and methods.
The new Date() Constructor
In JavaScript, date objects are created with new Date().
new Date() returns a date object with the current date and time.
Get the Current Time
const date = new Date();
Date Get Methods
Method
|
Description
|
getFullYear()
|
Get year as a four digit number (yyyy)
|
getMonth()
|
Get month as a number (0-11)
|
getDate()
|
Get day as a number (1-31)
|
getDay()
|
Get weekday as a number (0-6)
|
getHours()
|
Get hour (0-23)
|
getMinutes()
|
Get minute (0-59)
|
getSeconds()
|
Get second (0-59)
|
getMilliseconds()
|
Get millisecond (0-999)
|
getTime()
|
Get time (milliseconds since January 1, 1970)
|
Note 1
The get methods above return Local time.
Universal time (UTC) is documented at the bottom of this page.
Note 2
The get methods return information from existing date objects.
In a date object, the time is static. The "clock" is not "running".
The time in a date object is NOT the same as current time.
The getFullYear() Method
The getFullYear() method returns the year of a date as a four digit number:
Examples
const d = new Date("2021-03-25"); d.getFullYear();
const d = new Date(); d.getFullYear();
Warning !
Old JavaScript code might use the non-standard method getYear().
getYear() is supposed to return a 2-digit year.
getYear() is deprecated. Do not use it!
The getMonth() Method
The getMonth() method returns the month of a date as a number (0-11).
Note
In JavaScript, January is month number 0, February is number 1, ...
Finally, December is month number 11.
Examples
const d = new Date("2021-03-25"); d.getMonth();
const d = new Date(); d.getMonth();
Note
You can use an array of names to return the month as a name:
Examples
const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"];
const d = new Date("2021-03-25"); let month = months[d.getMonth()];
const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"];
const d = new Date(); let month = months[d.getMonth()];
The getDate() Method
The getDate() method returns the day of a date as a number (1-31):
Examples
const d = new Date("2021-03-25"); d.getDate();
const d = new Date(); d.getDate();
The getHours() Method
The getHours() method returns the hours of a date as a number (0-23):
Examples
const d = new Date("2021-03-25"); d.getHours();
const d = new Date(); d.getHours();
The getMinutes() Method
The getMinutes() method returns the minutes of a date as a number (0-59):
Examples
const d = new Date("2021-03-25"); d.getMinutes();
const d = new Date(); d.getMinutes();
The getSeconds() Method
The getSeconds() method returns the seconds of a date as a number (0-59):
Examples
const d = new
JavaScript Set Date Methods
Set Date methods let you set date values (years,
months, days, hours, minutes, seconds, milliseconds) for a Date Object.
Set Date Methods
Set Date methods are used for setting a part of a date:
Method |
Description |
setDate() |
Set the day as a number (1-31) |
setFullYear() |
Set the year (optionally month and day) |
setHours() |
Set the hour (0-23) |
setMilliseconds() |
Set the milliseconds (0-999) |
setMinutes() |
Set the minutes (0-59) |
setMonth() |
Set the month (0-11) |
setSeconds() |
Set the seconds (0-59) |
setTime() |
Set the time (milliseconds since January 1, 1970) |
The setFullYear() Method
The setFullYear() method sets the year of a date object. In this example to 2020:
Example
const d = new Date();
d.setFullYear(2020);
The setFullYear() method can optionally set month and day:
Example
const d = new Date();
d.setFullYear(2020, 11, 3);
The setMonth() Method
The setMonth() method sets the month of a date object (0-11):
Example
const d = new Date();
d.setMonth(11);
The setDate() Method
The setDate() method sets the day of a date object (1-31):
Example
const d = new Date();
d.setDate(15);
The setDate() method can also be used to add days to a date:
Example
const d = new Date();
d.setDate(d.getDate() + 50);
If adding days shifts the month or year, the changes are handled automatically by the Date object.
The setHours() Method
The setHours() method sets the hours of a date object (0-23):
Example
const d = new Date();
d.setHours(22);
The setMinutes() Method
The setMinutes() method sets the minutes of a date object (0-59):
Example
const d = new Date();
d.setMinutes(30);
The setSeconds() Method
The setSeconds() method sets the seconds of a date object (0-59):
Example
const d = new Date();
d.setSeconds(30);
Compare Dates
Dates can easily be compared.
The following example compares today's date with January 14, 2100:
Example
let text = "";
const today = new Date();
const someday = new Date();
someday.setFullYear(2100, 0, 14);
if (someday > today) { Â text = "Today is before January 14, 2100."; } else {
 text = "Today is after January 14, 2100."; }
JavaScript counts months from 0 to 11. January is 0. December is 11.
Complete JavaScript Date Reference
For a complete Date reference, go to our:
.
The reference contains descriptions and examples of all Date properties and
methods.
The JavaScript Math object allows you to perform mathematical tasks on numbers.
Example
Math.PI;
The Math Object
Unlike other objects, the Math aobject has no constructor.
The Math object is static.
All methods and properties can be used without creating a Math object first.
Math Properties (Constants)
The syntax for any Math property is : Math.property.
JavaScript provides 8 mathematical constants that can be accessed as Math properties:
Example
Math.E // returns Euler's number Math.PI // returns PI Math.SQRT2 // returns the square root of 2 Math.SQRT1_2 // returns the square root of 1/2 Math.LN2 // returns the natural logarithm of 2 Math.LN10 // returns the natural logarithm of 10 Math.LOG2E // returns base 2 logarithm of E Math.LOG10E // returns base 10 logarithm of E
Math Methods
The syntax for Math any methods is : Math.method(number)
Number to Integer
There are 4 common methods to round a number to an integer:
|
Math.round(x)
|
Returns x rounded to its nearest integer
|
|
|
Math.ceil(x)
|
Returns x rounded up to its nearest integer
|
|
|
Math.floor(x)
|
Returns x rounded down to its nearest integer
|
|
|
Math.trunc(x)
|
Returns the integer part of x (new in ES6)
|
Math.round()
Math.round(x) returns the nearest integer:
Examples
Math.round(4.6);
Math.round(4.5);
Math.round(4.4);
Math.ceil()
Math.ceil(x) returns the value of x rounded up to its nearest integer:
Example
Math.ceil(4.9); Math.ceil(4.7); Math.ceil(4.4); Math.ceil(4.2); Math.ceil(-4.2);
Math.floor()
Math.floor(x) returns the value of x rounded down to its nearest integer:
Example
Math.floor(4.9); Math.floor(4.7); Math.floor(4.4); Math.floor(4.2); Math.floor(-4.2);
Math.trunc()
Math.trunc(x) returns the integer part of x:
Example
Math.trunc(4.9); Math.trunc(4.7); Math.trunc(4.4); Math.trunc(4.2); Math.trunc(-4.2);
Math.sign()
Math.sign(x) returns if x is negative, null or positive:
Example
Math.sign(-4); Math.sign(0); Math.sign(4);
Math.trunc() and Math.sign() were added to JavaScript 2015 - ES6.
Math.pow()
Math.pow(x, y) returns the value of x to the power of y:
Example
Math.pow(8, 2);
Math.sqrt()
Math.sqrt(x) returns the square root of x:
Example
Math.sqrt(64);
Math.abs()
Math.abs(x) returns the absolute (positive) value of x:
Example
Math.abs(-4.7);
Math.sin()
Math.sin(x) returns the sine (a value between -1 and 1) of the angle x (given in radians).
If you want to use degrees instead of radians, you have to convert degrees to radians:
Angle in radians = Angle in degrees x PI / 180.
Example
Math.sin(90 * Math.PI / 180); // returns 1 (the sine of 90 degrees)
Math.cos()
Math.cos(x) returns the cosine (a value between -1 and 1) of the angle x (given in radians).
If you want to use degrees instead of radians, you have to convert degrees to radians:
Angle in radians = Angle in degrees x PI / 180.
Example
Math.cos(0 * Math.PI / 180); // returns 1 (the cos of 0 degrees)
Math.min() and Math.max()
Math.min() and Math.max() can be used to find the lowest or highest value in a list of arguments:
Example
Math.min(0, 150, 30, 20, -8, -200);
Example
Math.max(0, 150, 30, 20, -8, -200);
Math.random()
Math.random() returns a random number between 0 (inclusive), and 1 (exclusive):
Example
// Returns a random number: Math.random();
Math.random() always returns a number lower than 1.
JavaScript Random Integers
Math.random() used with Math.floor() can be used to return random integers.
There is no such thing as JavaScript integers.
We are talking about numbers with no decimals here.
Example
// Returns a random integer from 0 to 9: Math.floor(Math.random() * 10);
Example
// Returns a random integer from 0 to 10: Math.floor(Math.random() * 11);
Example
// Returns a random integer from 0 to 99: Math.floor(Math.random() * 100);
Example
// Returns a random integer from 0 to 100: Math.floor(Math.random() * 101);
Example
// Returns a random integer from 1 to 10: Math.floor(Math.random() * 10) + 1;
Example
// Returns a random integer from 1 to 100: Math.floor(Math.random() * 100) + 1;
A Proper Random Function
As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes.
This JavaScript function always returns a random number between min (included) and max (excluded):
Example
function getRndInteger(min, max) { return Math.floor(Math.random() * (max - min) ) + min; }
This JavaScript function always returns a random number between min and max (both included):
Example
function getRndInteger(min, max) { return Math.floor(Math.random() * (max - min + 1) ) + min;
A JavaScript Boolean represents one of two values: true or false.
Boolean Values
Very often, in programming, you will need a data type that can only have one of two values, like
- YES / NO
- ON / OFF
- TRUE / FALSE
For this, JavaScript has a Boolean data type. It can only take the values true or false.
The Boolean() Function
You can use the Boolean() function to find out if an expression (or a variable) is true:
Example
Boolean(10 > 9)
Or even easier:
Example
(10 > 9) 10 > 9
Comparisons and Conditions
The chapter JS Comparisons gives a full overview of comparison operators.
The chapter JS If Else gives a full overview of conditional statements.
Here are some examples:
Operator
|
Description
|
Example
|
==
|
equal to
|
if (day == "Monday")
|
>
|
greater than
|
if (salary > 9000)
|
<
|
less than
|
if (age < 18)
|
The Boolean value of an expression is the basis for all JavaScript comparisons and conditions.
Everything With a "Value" is True
Examples
100
3.14
-15
"Hello"
"false"
7 + 1 + 3.14
Everything Without a "Value" is False
The Boolean value of 0 (zero) is false:
let x = 0; Boolean(x);
The Boolean value of -0 (minus zero) is false:
let x = -0; Boolean(x);
The Boolean value of "" (empty string) is false:
let x = ""; Boolean(x);
The Boolean value of undefined is false:
let x; Boolean(x);
The Boolean value of null is false:
let x = null; Boolean(x);
The Boolean value of false is (you guessed it) false:
let x = false; Boolean(x);
The Boolean value of NaN is false:
let x = 10 / "Hallo"; Boolean(x);
JavaScript Booleans as Objects
Normally JavaScript booleans are primitive values created from literals:
let x = false;
But booleans can also be defined as objects with the keyword new:
let y = new Boolean(false);
Example
let x = false; let y = new Boolean(false);
// typeof x returns boolean // typeof y returns object
Do not create Boolean objects.
The new keyword complicates the code and slows down execution speed.
Boolean objects can produce unexpected results:
When using the == operator, x and y are equal:
let x = false; let y = new Boolean(false);
When using the === operator, x and y are not equal:
let x = false; let y = new Boolean(false);
Note the difference between (x==y) and (x===y).
(x == y) true or false?
let x = new Boolean(false); let y = new Boolean(false);
(x === y) true or false?
let x = new Boolean(false); let y = new Boolean(false);
Comparing two JavaScript objects always return false.
Complete Boolean Reference
For a complete reference, go to our Complete JavaScript Boolean Reference.
The reference contains descriptions and examples of all Boolean properties and methods.
Comparison and Logical operators are used to test for true or false.
Comparison Operators
Comparison operators are used in logical statements to determine equality or difference between variables or values.
Given that x = 5, the table below explains the comparison operators:
Operator
|
Description
|
Comparing
|
Returns
|
|
==
|
equal to
|
x == 8
|
false
|
|
x == 5
|
true
|
|
x == "5"
|
true
|
|
===
|
equal value and equal type
|
x === 5
|
true
|
|
x === "5"
|
false
|
|
!=
|
not equal
|
x != 8
|
true
|
|
!==
|
not equal value or not equal type
|
x !== 5
|
false
|
|
x !== "5"
|
true
|
|
x !== 8
|
true
|
|
>
|
greater than
|
x > 8
|
false
|
|
<
|
less than
|
x < 8
|
true
|
|
>=
|
greater than or equal to
|
x >= 8
|
false
|
|
<=
|
less than or equal to
|
x <= 8
|
true
|
|
How Can it be Used
Comparison operators can be used in conditional statements to compare values and take action depending on the result:
if (age < 18) text = "Too young to buy alcohol";
You will learn more about the use of conditional statements in the next chapter of this tutorial.
Logical Operators
Logical operators are used to determine the logic between variables or values.
Given that x = 6 and y = 3, the table below explains the logical operators:
Operator
|
Description
|
Example
|
|
&&
|
and
|
(x < 10 && y > 1) is true
|
|
||
|
or
|
(x == 5 || y == 5) is false
|
|
!
|
not
|
!(x == y) is true
|
|
Conditional (Ternary) Operator
JavaScript also contains a conditional operator that assigns a value to a variable based on some condition.
Syntax
variablename = (condition) ? value1:value2
Example
let voteable = (age < 18) ? "Too young":"Old enough";
If the variable age is a value below 18, the value of the variable voteable will be "Too young", otherwise the value of voteable will be "Old enough".
Comparing Different Types
Comparing data of different types may give unexpected results.
When comparing a string with a number, JavaScript will convert the string to a number when doing the comparison. An empty string converts to 0. A non-numeric string converts to NaN which is always false.
Case
|
Value
|
|
2 < 12
|
true
|
|
2 < "12"
|
true
|
|
2 < "John"
|
false
|
|
2 > "John"
|
false
|
|
2 == "John"
|
false
|
|
"2" < "12"
|
false
|
|
"2" > "12"
|
true
|
|
"2" == "12"
|
false
|
|
When comparing two strings, "2" will be greater than "12", because (alphabetically) 1 is less than 2.
To secure a proper result, variables should be converted to the proper type before comparison:
age = Number(age); if (isNaN(age)) { voteable = "Input is not a number"; } else { voteable = (age < 18) ? "Too young" : "Old enough"; }
Conditional statements are used to perform different actions based on different conditions.
Conditional Statements
Very often when you write code, you want to perform different actions for different decisions.
You can use conditional statements in your code to do this.
In JavaScript we have the following conditional statements:
- Use if to specify a block of code to be executed, if a specified condition is true
- Use else to specify a block of code to be executed, if the same condition is false
- Use else if to specify a new condition to test, if the first condition is false
- Use switch to specify many alternative blocks of code to be executed
The switch statement is described in the next chapter.
The if Statement
Use the if statement to specify a block of JavaScript code to be executed if a condition is true.
Syntax
if (condition) { // block of code to be executed if the condition is true }
Note that if is in lowercase letters. Uppercase letters (If or IF) will generate a JavaScript error.
Example
Make a "Good day" greeting if the hour is less than 18:00:
if (hour < 18) { greeting = "Good day"; }
The result of greeting will be:
The else Statement
Use the else statement to specify a block of code to be executed if the condition is false.
if (condition) { // block of code to be executed if the condition is true } else { // block of code to be executed if the condition is false }
Example
If the hour is less than 18, create a "Good day" greeting, otherwise "Good evening":
if (hour < 18) { greeting = "Good day"; } else { greeting = "Good evening"; }
The result of greeting will be:
Good evening
The else if Statement
Use the else if statement to specify a new condition if the first condition is false.
Syntax
if (condition1) { // block of code to be executed if condition1 is true } else if (condition2) { // block of code to be executed if the condition1 is false and condition2 is true } else { // block of code to be executed if the condition1 is false and condition2 is false }
Example
If time is less than 10:00, create a "Good morning" greeting, if not, but time is less than 20:00, create a "Good day" greeting, otherwise a "Good evening":
if (time < 10) { greeting = "Good morning"; } else if (time < 20) { greeting = "Good day"; } else { greeting = "Good evening"; }
The result of greeting will be:
Good evening
More Examples
Random link This example will write a link to either W3Schools or to the World Wildlife Foundation (WWF). By using a random number, there is a 50% chance for each of the links.
The switch statement is used to perform different actions based on different conditions.
The JavaScript Switch Statement
Use the switch statement to select one of many code blocks to be executed.
Syntax
switch(expression) { case x: // code block break; case y: // code block break; default: // code block }
This is how it works:
- The switch expression is evaluated once.
- The value of the expression is compared with the values of each case.
- If there is a match, the associated block of code is executed.
- If there is no match, the default code block is executed.
Example
The getDay() method returns the weekday as a number between 0 and 6.
(Sunday=0, Monday=1, Tuesday=2 ..)
This example uses the weekday number to calculate the weekday name:
switch (new Date().getDay()) { case 0: day = "Sunday"; break; case 1: day = "Monday"; break; case 2: day = "Tuesday"; break; case 3: day = "Wednesday"; break; case 4: day = "Thursday"; break; case 5: day = "Friday"; break; case 6: day = "Saturday"; }
The result of day will be:
Tuesday
The break Keyword
When JavaScript reaches a break keyword, it breaks out of the switch block.
This will stop the execution inside the switch block.
It is not necessary to break the last case in a switch block. The block breaks (ends) there anyway.
Note: If you omit the break statement, the next case will be executed even if the evaluation does not match the case.
The default Keyword
The default keyword specifies the code to run if there is no case match:
Example
The getDay() method returns the weekday as a number between 0 and 6.
If today is neither Saturday (6) nor Sunday (0), write a default message:
switch (new Date().getDay()) { case 6: text = "Today is Saturday"; break; case 0: text = "Today is Sunday"; break; default: text = "Looking forward to the Weekend"; }
The result of text will be:
Looking forward to the Weekend
The default case does not have to be the last case in a switch block:
Example
switch (new Date().getDay()) { default: text = "Looking forward to the Weekend"; break; case 6: text = "Today is Saturday"; break; case 0: text = "Today is Sunday"; }
If default is not the last case in the switch block, remember to end the default case with a break.
Common Code Blocks
Sometimes you will want different switch cases to use the same code.
In this example case 4 and 5 share the same code block, and 0 and 6 share another code block:
Example
switch (new Date().getDay()) { case 4: case 5: text = "Soon it is Weekend"; break; case 0: case 6: text = "It is Weekend"; break; default: text = "Looking forward to the Weekend"; }
Switching Details
If multiple cases matches a case value, the first case is selected.
If no matching cases are found, the program continues to the default label.
If no default label is found, the program continues to the statement(s) after the switch.
Strict Comparison
Switch cases use strict comparison (===).
The values must be of the same type to match.
A strict comparison can only be true if the operands are of the same type.
In this example there will be no match for x:
Example
let x = "0"; switch (x) { case 0: text =
JavaScript For Loop
Loops can execute a block of code a number of times.
JavaScript Loops
Loops are handy, if you want to run the same code over and over again, each
time with a different value.
Often this is the case when working with arrays:
Instead of writing:
text += cars[0] + "<br>"; text += cars[1] + "<br>";
text += cars[2] + "<br>"; text += cars[3] + "<br>";
text += cars[4] + "<br>"; text += cars[5] + "<br>";
You can write:
for (let i = 0; i < cars.length; i++) { Â Â text += cars[i] + "<br>";
}
Different Kinds of Loops
JavaScript supports different kinds of loops:
-
for - loops through a block of code a number of times
-
for/in - loops through the properties of an object
-
for/of - loops through the values of an
iterable object
-
while - loops through a block of code while a specified condition is true
-
do/while - also loops through a block of code while a specified condition is true
The For Loop
The for statement creates a loop with 3 optional expressions:
for (expression 1; expression 2; expression 3) {
 // code block to be executed
}
Expression 1 is executed (one time) before the execution of the code block.
Expression 2 defines the condition for executing the code block.
Expression 3 is executed (every time) after the code block has been executed.
Example
for (let i = 0; i < 5; i++) {
 text += "The number is " + i + "<br>";
}
From the example above, you can read:
Expression 1 sets a variable before the loop starts (let i = 0).
Expression 2 defines the condition for the loop to run (i must be less than
5).
Expression 3 increases a value (i++) each time the code block in the loop has
been executed.
Expression 1
Normally you will use expression 1 to initialize the variable used in the loop (let i = 0).
This is not always the case. JavaScript doesn't care. Expression 1 is
optional.
You can initiate many values in expression 1 (separated by comma):
Example
for (let i = 0, len = cars.length, text = ""; i < len; i++) { Â text += cars[i] + "<br>";
}
And you can omit expression 1 (like when your values are set
before the loop starts):
Example
let i = 2;
let len = cars.length;
let text = ""; for (; i < len; i++) {
 text += cars[i] + "<br>";
}
Expression 2
Often expression 2 is used to evaluate the condition of the initial variable.
This is not always the case. JavaScript doesn't care. Expression 2 is
also optional.
If expression 2 returns true, the loop will start over again. If it returns false, the
loop will end.
If you omit expression 2, you must provide a break inside the
loop. Otherwise the loop will never end. This will crash your browser.
Read about breaks in a later chapter of this tutorial.
Expression 3
Often expression 3 increments the value of the initial variable.
This is not always the case. JavaScript doesn't care. Expression 3 is
optional.
Expression 3 can do anything like negative increment (i--), positive
increment (i = i + 15), or anything else.
Expression 3 can also be omitted (like when you increment your values inside the loop):
Example
let i = 0;
let len = cars.length;
let text = "";
for (; i < len; ) { Â text += cars[i] + "<br>";
Â
i++; }
Loop Scope
Using var in a loop:
Example
var i = 5;
for (var i = 0; i < 10; i++) {
  // some code }
// Here i is 10
Using let in a loop:
Example
let i = 5;
for (let i = 0; i < 10; i++) {
 // some code }
// Here i is 5
In the first example, using var , the variable declared in
the loop redeclares the variable outside the loop.
In the second example, using let , the variable declared in
the loop does not redeclare the variable outside the loop.
When let is used to declare the i variable in a loop, the i
variable will only be visible within the loop.
For/Of and For/In Loops
The for/in loop and the for/of loop are explained in the next chapter.
While Loops
The while loop and the do/while are explained in the next chapters.
The For In Loop
The JavaScript for in statement loops through the properties of an Object:
Syntax
for (key in object) { // code block to be executed }
Example
const person = {fname:"John", lname:"Doe", age:25};
let text = ""; for (let x in person) { text += person[x]; }
Example Explained
- The for in loop iterates over a person object
- Each iteration returns a key (x)
- The key is used to access the value of the key
- The value of the key is person[x]
For In Over Arrays
The JavaScript for in statement can also loop over the properties of an Array:
Syntax
for (variable in array) { code }
Example
const numbers = [45, 4, 9, 16, 25];
let txt = ""; for (let x in numbers) { txt += numbers[x]; }
Do not use for in over an Array if the index order is important.
The index order is implementation-dependent, and array values may not be accessed in the order you expect.
It is better to use a for loop, a for of loop, or Array.forEach() when the order is important.
Array.forEach()
The forEach() method calls a function (a callback function) once for each array element.
Example
const numbers = [45, 4, 9, 16, 25];
let txt = ""; numbers.forEach(myFunction);
function myFunction(value, index, array) { txt += value; }
Note that the function takes 3 arguments:
- The item value
- The item index
- The array itself
The example above uses only the value parameter. It can be rewritten to:
Example
const numbers = [45, 4, 9, 16, 25];
let txt = ""; numbers.forEach(myFunction);
function myFunction(value) { txt += value; }
The For Of Loop
The JavaScript for of statement loops through the values of an iterable object.
It lets you loop over iterable data structures such as Arrays, Strings, Maps, NodeLists, and more:
Syntax
for (variable of iterable) { // code block to be executed }
variable - For every iteration the value of the next property is assigned to the variable. Variable can be declared with const, let, or var.
iterable - An object that has iterable properties.
Browser Support
For/of was added to JavaScript in 2015 (ES6)
Safari 7 was the first browser to support for of:
|
|
|
|
|
Chrome 38
|
Edge 12
|
Firefox 51
|
Safari 7
|
Opera 25
|
Oct 2014
|
Jul 2015
|
Oct 2016
|
Oct 2013
|
Oct 2014
|
For/of is not supported in Internet Explorer.
Looping over an Array
Example
const cars = ["BMW", "Volvo", "Mini"];
let text = ""; for (let x of cars) { text += x; }
Looping over a String
Example
let language = "JavaScript";
let text = ""; for (let x of language) { text += x; }
The While Loop
The while loop and the do/while loop are explained in the next chapter.
Loops can execute a block of code as long as a specified condition is true.
The While Loop
The while loop loops through a block of code as long as a specified condition is true.
Syntax
while (condition) { // code block to be executed }
Example
In the following example, the code in the loop will run, over and over again, as long as a variable (i) is less than 10:
Example
while (i < 10) { text += "The number is " + i; i++; }
If you forget to increase the variable used in the condition, the loop will never end. This will crash your browser.
The Do While Loop
The do while loop is a variant of the while loop. This loop will execute the code block once, before checking if the condition is true, then it will repeat the loop as long as the condition is true.
Syntax
do { // code block to be executed } while (condition);
Example
The example below uses a do while loop. The loop will always be executed at least once, even if the condition is false, because the code block is executed before the condition is tested:
Example
do { text += "The number is " + i; i++; } while (i < 10);
Do not forget to increase the variable used in the condition, otherwise the loop will never end!
Comparing For and While
If you have read the previous chapter, about the for loop, you will discover that a while loop is much the same as a for loop, with statement 1 and statement 3 omitted.
The loop in this example uses a for loop to collect the car names from the cars array:
Example
const cars = ["BMW", "Volvo", "Saab", "Ford"]; let i = 0; let text = "";
for (;cars[i];) { text += cars[i]; i++; }
The loop in this example uses a while loop to collect the car names from the cars array:
Example
const cars = ["BMW", "Volvo", "Saab", "Ford"]; let i = 0; let text = "";
while (cars[i]) { text += cars[i]; i++; }
The break statement "jumps out" of a loop.
The continue statement "jumps over" one iteration in the loop.
The Break Statement
You have already seen the break statement used in an earlier chapter of this tutorial. It was used to "jump out" of a switch() statement.
The break statement can also be used to jump out of a loop:
Example
for (let i = 0; i < 10; i++) { if (i === 3) { break; } text += "The number is " + i + "<br>"; }
In the example above, the break statement ends the loop ("breaks" the loop) when the loop counter (i) is 3.
The Continue Statement
The continue statement breaks one iteration (in the loop), if a specified condition occurs, and continues with the next iteration in the loop.
This example skips the value of 3:
Example
for (let i = 0; i < 10; i++) { if (i === 3) { continue; } text += "The number is " + i + "<br>"; }
JavaScript Labels
To label JavaScript statements you precede the statements with a label name and a colon:
label: statements
The break and the continue statements are the only JavaScript statements that can "jump out of" a code block.
Syntax:
break labelname;
continue labelname;
The continue statement (with or without a label reference) can only be used to skip one loop iteration.
The break statement, without a label reference, can only be used to jump out of a loop or a switch.
With a label reference, the break statement can be used to jump out of any code block:
Example
const cars = ["BMW", "Volvo", "Saab", "Ford"]; list: { text += cars[0] + "<br>"; text += cars[1] + "<br>"; break list; text += cars[2] + "<br>"; text += cars[3] + "<br>"; }
A code block is a block of code between { and }.
Iterables are iterable objects (like Arrays).
Iterables can be accessed with simple and efficient code.
Iterables can be iterated over with for..of loops
The For Of Loop
The JavaScript for..of statement loops through the elements of an iterable object.
Syntax
for (variable of iterable) { // code block to be executed }
Iterating
Iterating is easy to understand.
It simply means looping over a sequence of elements.
Here are some easy examples:
- Iterating over a String
- Iterating over an Array
Iterating Over a String
You can use a for..of loop to iterate over the elements of a string:
Example
const name = "W3Schools";
for (const x of name) { // code block to be executed }
Iterating Over an Array
You can use a for..of loop to iterate over the elements of an Array:
Example
const letters = ["a","b","c"];
for (const x of letters) { // code block to be executed }
You can learn more details about Iterables in the chapter JS Object Iterables.
Iterating Over a Set
You can use a for..of loop to iterate over the elements of a Set:
Example
const letters = new Set(["a","b","c"]);
for (const x of letters) { // code block to be executed }
Sets and Maps are covered in the next chapters.
Iterating Over a Map
You can use a for..of loop to iterate over the elements of a Map:
Example
const fruits = new Map([ ["apples", 500], ["bananas", 300], ["oranges", 200] ]);
for (const x of fruits) { // code block to be executed }
A JavaScript Set is a collection of unique values.
Each value can only occur once in a Set.
Essential Set Methods
Method
|
Description
|
new Set()
|
Creates a new Set
|
add()
|
Adds a new element to the Set
|
delete()
|
Removes an element from a Set
|
has()
|
Returns true if a value exists in the Set
|
forEach()
|
Invokes a callback for each element in the Set
|
values()
|
Returns an iterator with all the values in a Set
|
Property
|
Description
|
size
|
Returns the number of elements in a Set
|
How to Create a Set
You can create a JavaScript Set by:
- Passing an Array to new Set()
- Create a new Set and use add() to add values
- Create a new Set and use add() to add variables
The new Set() Method
Pass an Array to the new Set() constructor:
Example
// Create a Set const letters = new Set(["a","b","c"]);
Create a Set and add values:
Example
// Create a Set const letters = new Set();
// Add Values to the Set letters.add("a"); letters.add("b"); letters.add("c");
Create a Set and add variables:
Example
// Create a Set const letters = new Set();
// Create Variables const a = "a"; const b = "b"; const c = "c";
// Add Variables to the Set letters.add(a); letters.add(b); letters.add(c);
The add() Method
Example
letters.add("d"); letters.add("e");
If you add equal elements, only the first will be saved:
Example
letters.add("a"); letters.add("b"); letters.add("c"); letters.add("c"); letters.add("c"); letters.add("c"); letters.add("c"); letters.add("c");
The forEach() Method
The forEach() method invokes (calls) a function for each Set element:
Example
// Create a Set const letters = new Set(["a","b","c"]);
// List all Elements let text = ""; letters.forEach (function(value) { text += value; })
The values() Method
The values() method returns a new iterator object containing all the values in a Set:
Example
letters.values() // Returns [object Set Iterator]
Now you can use the Iterator object to access the elements:
Example
// List all Elements let text = ""; for (const x of letters.values()) { text += x; }
A Map holds key-value pairs where the keys can be any datatype.
A Map remembers the original insertion order of the keys.
Essential Map Methods
Method
|
Description
|
new Map()
|
Creates a new Map
|
set()
|
Sets the value for a key in a Map
|
get()
|
Gets the value for a key in a Map
|
delete()
|
Removes a Map element specified by the key
|
has()
|
Returns true if a key exists in a Map
|
forEach()
|
Calls a function for each key/value pair in a Map
|
entries()
|
Returns an iterator with the [key, value] pairs in a Map
|
Property
|
Description
|
size
|
Returns the number of elements in a Map
|
How to Create a Map
You can create a JavaScript Map by:
- Passing an Array to
new Map()
- Create a Map and use
Map.set()
The new Map() Method
You can create a Map by passing an Array to the new Map() constructor:
Example
The set() Method
You can add elements to a Map with the set() method:
Example
The set() method can also be used to change existing Map values:
Example
fruits.set("apples", 200);
The get() Method
The get() method gets the value of a key in a Map:
Example
fruits.get("apples");
The size Property
The size property returns the number of elements in a Map:
Example
fruits.size;
The delete() Method
The delete() method removes a Map element:
Example
fruits.delete("apples");
The has() Method
The has() method returns true if a key exists in a Map:
Example
fruits.has("apples");
Try This:
fruits.delete("apples"); fruits.has("apples");
JavaScript Objects vs Maps
Differences between JavaScript Objects and Maps:
|
Object
|
Map
|
Iterable
|
Not directly iterable
|
Directly iterable
|
Size
|
Do not have a size property
|
Have a size property
|
Key Types
|
Keys must be Strings (or Symbols)
|
Keys can be any datatype
|
Key Order
|
Keys are not well ordered
|
Keys are ordered by insertion
|
Defaults
|
Have default keys
|
Do not have default keys
|
The forEach() Method
The forEach() method calls a function for each key/value pair in a Map:
Example
The entries() Method
The entries() method returns an iterator object with the [key, values] in a Map:
Example
Browser Support
JavaScript Maps are supported in all browsers, except Internet Explorer:
Chrome
|
Edge
|
Firefox
|
Safari
|
Opera
|
In JavaScript there are 5 different data types that can contain values:
string
number
boolean
object
function
There are 6 types of objects:
Object
Date
Array
String
Number
Boolean
And 2 data types that cannot contain values:
The typeof Operator
You can use the typeof operator to find the data type of a
JavaScript variable.
Example
typeof "John"Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â
// Returns "string"
typeof 3.14Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â
// Returns "number"
typeof NaN Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â
// Returns "number"
typeof false                Â
// Returns "boolean"
typeof [1,2,3,4]Â Â Â Â Â Â Â Â Â Â Â Â Â Â // Returns
"object"
typeof {name:'John', age:34}Â
// Returns "object" typeof new Date()Â Â Â Â Â Â Â Â Â Â Â Â
// Returns "object" typeof function () {} Â Â Â Â Â Â Â // Returns
"function"
typeof myCar                Â
// Returns "undefined" *
typeof null                 Â
// Returns "object"
Please observe:
- The data type of NaN is number
- The data type of an array is object
- The data type of a date is object
- The data type of null is object
- The data type of an undefined variable is undefined
*
- The data type of a variable that has not been assigned a value is
also undefined *
You cannot use typeof to determine if a JavaScript object is an array (or a date).
Primitive Data
A primitive data value is a single simple data value with no additional
properties and methods.
The typeof operator can return one of these primitive types:
string
number
boolean
undefined
Example
typeof "John"Â Â Â Â Â Â Â Â Â Â Â Â Â Â // Returns
"string"
typeof 3.14Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â // Returns
"number"
typeof true                // Returns
"boolean" typeof false               // Returns
"boolean" typeof x                 Â
// Returns "undefined" (if x has no value)
Complex Data
The typeof operator can return one of two complex types:
The typeof operator returns "object" for objects, arrays, and null.
The typeof operator does not return "object" for functions.
Example
typeof {name:'John', age:34}Â // Returns "object"
typeof [1,2,3,4]Â Â Â Â Â Â Â Â Â Â Â Â
// Returns "object" (not "array", see note below)
typeof null                 // Returns
"object" typeof function myFunc(){}Â Â // Returns "function"
The typeof operator returns "object " for arrays because in JavaScript arrays are objects.
The Data Type of typeof
The typeof operator is not a variable. It is an operator. Operators ( + - * /
) do not have any data type.
But, the typeof operator always returns a string (containing
the type of the operand).
The constructor Property
The constructor property returns the constructor
function for all JavaScript variables.
Example
"John".constructor              Â
// Returns function String()Â {[native code]}
(3.14).constructor              Â
// Returns function Number()Â {[native code]}
false.constructor                 // Returns
function Boolean() {[native code]}
[1,2,3,4].constructor           Â
// Returns function Array()Â Â {[native code]}
{name:'John',age:34}.constructorÂ
// Returns function Object()Â {[native code]}
new Date().constructor          Â
// Returns function Date()Â Â {[native code]}
function () {}.constructor        // Returns
function Function(){[native code]}
You can check the constructor property to find out if an object is an Array
(contains the word "Array"):
Example
function isArray(myArray) {
 return myArray.constructor.toString().indexOf("Array") > -1;
}
Or even simpler, you can check if the object is an Array function:
Example
function isArray(myArray) {
 return myArray.constructor
=== Array;
}
You can check the constructor property to find out if an object is a
Date (contains the word "Date"):
Example
function isDate(myDate) {
 return myDate.constructor.toString().indexOf("Date") > -1;
}
Or even simpler, you can check if the object is a Date function:
Example
function isDate(myDate) {
 return myDate.constructor === Date;
}
Undefined
In JavaScript, a variable without a value, has the value undefined .
The type is also undefined .
Example
let car;Â Â Â // Value is undefined,
type is undefined
Any variable can be emptied, by setting the value to undefined .
The type will also be undefined .
Example
car = undefined;Â Â Â // Value is undefined,
type is undefined
Empty Values
An empty value has nothing to do with undefined .
An empty string has both a legal value and a type.
Example
let car = "";Â Â Â //
The value is
"", the typeof is "string"
Null
In JavaScript null is "nothing". It is supposed to be something that doesn't exist.
Unfortunately, in JavaScript, the data type of null is an object.
You can consider it a bug in JavaScript that typeof null is an object. It should be null .
You can empty an object by setting it to null :
Example
let person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"}; person = null;Â Â Â //
Now value is null,
but type is still an object
You can also empty an object by setting it to undefined :
Example
let person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"}; person = undefined;Â Â //
Now both value and type is undefined
Difference Between Undefined and Null
undefined and null are equal in value but different in type:
typeof undefined         Â
// undefined typeof null              Â
// object
null === undefined       Â
// false null == undefined        Â
// true
The instanceof Operator
The instanceof operator returns true if an object is an instance of the specified object:
Example
const cars = ["Saab", "Volvo", "BMW"];
(cars instanceof Array);
(cars instanceof Object);
(cars instanceof String);
(cars instanceof Number);
The void Operator
The void operator evaluates an expression and returns
undefined. This operator is often used to obtain the undefined
primitive value, using "void(0)" (useful when evaluating an expression without
using the return value).
Example
<a href="javascript:void(0);"> Â Useless link </a>
<a href="javascript:void(document.body.style.backgroundColor='red');"> Â Click me to change the background color of body to red </a>
· Converting Strings to Numbers
· Converting Numbers to Strings
· Converting Dates to Numbers
· Converting Numbers to Dates
· Converting Booleans to Numbers
· Converting Numbers to Booleans
JavaScript Type Conversion
JavaScript variables can be converted to a new variable and another data type:
- By the use of a JavaScript function
- Automatically by JavaScript itself
Converting Strings to Numbers
The global method Number() converts a variable (or a value) into a number.
A numeric string (like "3.14") converts to a number (like 3.14).
An empty string (like "") converts to 0.
A non numeric string (like "John") converts to NaN (Not a Number).
Examples
These will convert:
Number("3.14") Number(Math.PI) Number(" ") Number("")
These will not convert:
Number("99 88") Number("John")
Number Methods
In the chapter Number Methods, you will find more methods that can be used to convert strings to numbers:
Method
|
Description
|
Number()
|
Returns a number, converted from its argument
|
|
|
parseFloat()
|
Parses a string and returns a floating point number
|
parseInt()
|
Parses a string and returns an integer
|
The Unary + Operator
The unary + operator can be used to convert a variable to a number:
Example
let y = "5"; // y is a string let x = + y; // x is a number
If the variable cannot be converted, it will still become a number, but with the value NaN (Not a Number):
Example
let y = "John"; // y is a string let x = + y; // x is a number (NaN)
Converting Numbers to Strings
The global method String() can convert numbers to strings.
It can be used on any type of numbers, literals, variables, or expressions:
Example
String(x) // returns a string from a number variable x String(123) // returns a string from a number literal 123 String(100 + 23) // returns a string from a number from an expression
The Number method toString() does the same.
Example
x.toString() (123).toString() (100 + 23).toString()
More Methods
In the chapter Number Methods, you will find more methods that can be used to convert numbers to strings:
Method
|
Description
|
toExponential()
|
Returns a string, with a number rounded and written using exponential notation.
|
toFixed()
|
Returns a string, with a number rounded and written with a specified number of decimals.
|
toPrecision()
|
Returns a string, with a number written with a specified length
|
Converting Dates to Numbers
The global method Number() can be used to convert dates to numbers.
d = new Date(); Number(d) // returns 1404568027739
The date method getTime() does the same.
d = new Date(); d.getTime() // returns 1404568027739
Converting Dates to Strings
The global method String() can convert dates to strings.
String(Date()) // returns "Thu Jul 17 2014 15:38:19 GMT+0200 (W. Europe Daylight Time)"
The Date method toString() does the same.
Example
Date().toString() // returns "Thu Jul 17 2014 15:38:19 GMT+0200 (W. Europe Daylight Time)"
In the chapter Date Methods, you will find more methods that can be used to convert dates to strings:
Method
|
Description
|
getDate()
|
Get the day as a number (1-31)
|
getDay()
|
Get the weekday a number (0-6)
|
getFullYear()
|
Get the four digit year (yyyy)
|
getHours()
|
Get the hour (0-23)
|
getMilliseconds()
|
Get the milliseconds (0-999)
|
getMinutes()
|
Get the minutes (0-59)
|
getMonth()
|
Get the month (0-11)
|
getSeconds()
|
Get the seconds (0-59)
|
getTime()
|
Get the time (milliseconds since January 1, 1970)
|
Co
JavaScript Bitwise Operators
Operator
|
Name
|
Description
|
&
|
AND
|
Sets each bit to 1 if both bits are 1
|
|
|
OR
|
Sets each bit to 1 if one of two bits is 1
|
^
|
XOR
|
Sets each bit to 1 if only one of two bits is 1
|
~
|
NOT
|
Inverts all the bits
|
<<
|
Zero fill left shift
|
Shifts left by pushing zeros in from the right and let the leftmost bits fall off
|
>>
|
Signed right shift
|
Shifts right by pushing copies of the leftmost bit in from the left, and let the rightmost bits fall off
|
>>>
|
Zero fill right shift
|
Shifts right by pushing zeros in from the left, and let the rightmost bits fall off
|
Examples
Operation
|
Result
|
Same as
|
Result
|
5 & 1
|
1
|
0101 & 0001
|
0001
|
5 | 1
|
5
|
0101 | 0001
|
0101
|
~ 5
|
10
|
~0101
|
1010
|
5 << 1
|
10
|
0101 << 1
|
1010
|
5 ^ 1
|
4
|
0101 ^ 0001
|
0100
|
5 >> 1
|
2
|
0101 >> 1
|
0010
|
5 >>> 1
|
2
|
0101 >>> 1
|
0010
|
JavaScript Uses 32 bits Bitwise Operands
JavaScript stores numbers as 64 bits floating point numbers, but all bitwise operations are performed on 32 bits binary numbers.
Before a bitwise operation is performed, JavaScript converts numbers to 32 bits signed integers.
After the bitwise operation is performed, the result is converted back to 64 bits JavaScript numbers.
The examples above uses 4 bits unsigned binary numbers. Because of this ~ 5 returns 10.
Since JavaScript uses 32 bits signed integers, it will not return 10. It will return -6.
00000000000000000000000000000101 (5)
11111111111111111111111111111010 (~5 = -6)
A signed integer uses the leftmost bit as the minus sign.
JavaScript Bitwise AND
When a bitwise AND is performed on a pair of bits, it returns 1 if both bits are 1.
One bit example:
Operation
|
Result
|
0 & 0
|
0
|
0 & 1
|
0
|
1 & 0
|
0
|
1 & 1
|
1
|
4 bits example:
Operation
|
Result
|
1111 & 0000
|
0000
|
1111 & 0001
|
0001
|
1111 & 0010
|
0010
|
1111 & 0100
|
0100
|
JavaScript Bitwise OR
When a bitwise OR is performed on a pair of bits, it returns 1 if one of the bits is 1:
One bit example:
Operation
|
Result
|
0 | 0
|
0
|
0 | 1
|
1
|
1 | 0
|
1
|
1 | 1
|
1
|
4 bits example:
Operation
|
Result
|
1111 | 0000
|
1111
|
1111 | 0001
|
1111
|
1111 | 0010
|
1111
|
1111 | 0100
|
1111
|
JavaScript Bitwise XOR
When a bitwise XOR is performed on a pair of bits, it returns 1 if the bits are different:
One bit example:
Operation
|
Result
|
A regular expression is a sequence of characters that forms a search pattern.
The search pattern can be used for text search and text replace operations.
What Is a Regular Expression?
A regular expression is a sequence of characters that forms a search pattern.
When you search for data in a text, you can use this search pattern to describe what you are searching for.
A regular expression can be a single character, or a more complicated pattern.
Regular expressions can be used to perform all types of text search and text replace operations.
Syntax
/pattern/modifiers;
Example
/w3schools/i;
Example explained:
/w3schools/i is a regular expression.
w3schools is a pattern (to be used in a search).
i is a modifier (modifies the search to be case-insensitive).
Using String Methods
In JavaScript, regular expressions are often used with the two string methods: search() and replace().
The search() method uses an expression to search for a match, and returns the position of the match.
The replace() method returns a modified string where the pattern is replaced.
Using String search() With a String
The search() method searches a string for a specified value and returns the position of the match:
Example
Use a string to do a search for "W3schools" in a string:
let text = "Visit W3Schools!"; let n = text.search("W3Schools");
The result in n will be:
6
Using String search() With a Regular Expression
Example
Use a regular expression to do a case-insensitive search for "w3schools" in a string:
let text = "Visit W3Schools"; let n = text.search(/w3schools/i);
The result in n will be:
6
Using String replace() With a String
The replace() method replaces a specified value with another value in a string:
let text = "Visit Microsoft!"; let result = text.replace("Microsoft", "W3Schools");
Use String replace() With a Regular Expression
Example
Use a case insensitive regular expression to replace Microsoft with W3Schools in a string:
let text = "Visit Microsoft!"; let result = text.replace(/microsoft/i, "W3Schools");
The result in res will be:
Visit W3Schools!
Did You Notice?
Regular expression arguments (instead of string arguments) can be used in the methods above. Regular expressions can make your search much more powerful (case insensitive for example).
Regular Expression Modifiers
Modifiers can be used to perform case-insensitive more global searches:
Modifier
|
Description
|
|
i
|
Perform case-insensitive matching
|
|
g
|
Perform a global match (find all)
|
|
m
|
Perform multiline matching
|
|
d
|
Perform start and end matching (New in ES2022)
|
|
Regular Expression Patterns
Brackets are used to find a range of characters:
Expression
|
Description
|
|
[abc]
|
Find any of the characters between the brackets
|
|
[0-9]
|
Find any of the digits between the brackets
|
|
(x|y)
|
Find any of the alternatives separated with |
|
|
Metacharacters are characters with a special meaning:
Metacharacter
|
Description
|
|
d
|
Find a digit
|
|
s
|
Find a whitespace character
|
|
b
|
Find a match at the beginning of a word like this: bWORD, or at the end of a word like this: WORDb
|
|
uxxxx
|
Find the Unicode character specified by the hexadecimal number xxxx
|
|
Quantifiers define quantities:
Quantifier
|
Description
|
|
n+
|
Matches any string that contains at least one n
|
|
n*
|
Matches any string that contains zero or more occurrences of n
|
|
n?
|
Matches any string that contains zero or one occurrences of n
|
|
Using the RegExp Object
In JavaScript, the RegExp object is a regular expression object with predefined properties and methods.
Using test()
The test() method is a RegExp expression method.
It searches a string for a pattern, and returns true or false, depending on the result.
The following example searches a string for the character "e":
Example
const pattern = /e/; pattern.test("The best things in life are free!");
Since there is an "e" in the string, the output of the code above will be:
true
Operator precedence describes the order in which operations are performed in an arithmetic expression.
Multiplication (*) and division (/) have higher precedence than addition (+) and subtraction (-).
As in traditional mathematics, multiplication is done first:
let x = 100 + 50 * 3;
When using parentheses, operations inside the parentheses are computed first:
let x = (100 + 50) * 3;
Operations with the same precedence (like * and /) are computed from left to right:
let x = 100 / 50 * 3;
Operator Precedence Values
Expressions in parentheses are computed before the rest of the expression Function are executed before the result is used in the rest of the expression
|
Val
|
Operator
|
Description
|
Example
|
18
|
( )
|
Expression Grouping
|
(100 + 50) * 3
|
17
|
.
|
Member Of
|
person.name
|
17
|
[]
|
Member Of
|
person["name"]
|
17
|
?.
|
Optional Chaining ES2020
|
x ?. y
|
17
|
()
|
Function Call
|
myFunction()
|
17
|
new
|
New with Arguments
|
new Date("June 5,2022")
|
16
|
new
|
New without Arguments
|
new Date()
|
Increment Operators Postfix increments are executed before prefix increments
|
15
|
++
|
Postfix Increment
|
i++
|
15
|
--
|
Postfix Decrement
|
i--
|
14
|
++
|
Prefix Increment
|
++i
|
14
|
--
|
Prefix Decrement
|
--i
|
NOT Operators
|
14
|
!
|
Logical NOT
|
!(x==y)
|
14
|
~
|
Bitwise NOT
|
~x
|
Unary Operators
|
14
|
+
|
Unary Plus
|
+x
|
14
|
-
|
Unary Minus
|
-x
|
14
|
typeof
|
Data Type
|
typeof x
|
14
|
void
|
Evaluate Void
|
void(0)
|
14
|
delete
|
Property Delete
|
delete myCar.color
|
Arithmetic Operators Exponentiations are executed before multiplications Multiplications and divisions are executed before additions and subtractions
|
13
|
**
|
Exponentiation ES2016
|
10 ** 2
|
12
|
*
|
Multiplication
|
10 * 5
|
12
|
/
|
Division
|
10 / 5
|
12
|
%
|
Division Remainder
|
10 % 5
|
11
|
+
|
Addition
|
10 + 5
|
11
|
-
|
Subtraction
|
10 - 5
|
11
|
+
|
Concatenation
|
"John" + "Doe"
|
Shift Operators
|
10
|
<<
|
Shift Left
|
x << 2
|
10
|
>>
|
Shift Right (signed)
|
x >> 2
|
10
|
>>>
|
Shift Right (unsigned)
|
x >>> 2
|
Relational Operators
|
9
|
Throw, and Try...Catch...Finally
The try statement defines a code block to run (to try).
The catch statement defines a code block to handle any error.
The finally statement defines a code block to run regardless of the result.
The throw statement defines a custom error.
Errors Will Happen!
When executing JavaScript code, different errors can occur.
Errors can be coding errors made by the programmer, errors due to wrong input, and other unforeseeable things.
Example
In this example we misspelled "alert" as "adddlert" to deliberately produce an error:
<p id="demo"></p>
<script> try { adddlert("Welcome guest!"); } catch(err) { document.getElementById("demo").innerHTML = err.message; } </script>
JavaScript catches adddlert as an error, and executes the catch code to handle it.
JavaScript try and catch
The try statement allows you to define a block of code to be tested for errors while it is being executed.
The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
The JavaScript statements try and catch come in pairs:
try { Block of code to try } catch(err) { Block of code to handle errors }
JavaScript Throws Errors
When an error occurs, JavaScript will normally stop and generate an error message.
The technical term for this is: JavaScript will throw an exception (throw an error).
JavaScript will actually create an Error object with two properties: name and message.
The throw Statement
The throw statement allows you to create a custom error.
Technically you can throw an exception (throw an error).
The exception can be a JavaScript String, a Number, a Boolean or an Object:
throw "Too big"; // throw a text throw 500; // throw a number
If you use throw together with try and catch, you can control program flow and generate custom error messages.
Input Validation Example
This example examines input. If the value is wrong, an exception (err) is thrown.
The exception (err) is caught by the catch statement and a custom error message is displayed:
<!DOCTYPE html> <html> <body>
<p>Please input a number between 5 and 10:</p>
<input id="demo" type="text"> <button type="button" onclick="myFunction()">Test Input</button> <p id="p01"></p>
<script> function myFunction() { const message = document.getElementById("p01"); message.innerHTML = ""; let x = document.getElementById("demo").value; try { if(x.trim() == "") throw "empty"; if(isNaN(x)) throw "not a number"; x = Number(x); if(x < 5) throw "too low"; if(x > 10) throw "too high"; } catch(err) { message.innerHTML = "Input is " + err; } } </script>
</body> </html>
HTML Validation
The code above is just an example.
Modern browsers will often use a combination of JavaScript and built-in HTML validation, using predefined validation rules defined in HTML attributes:
<input id="demo" type="number" min="5" max="10" step="1">
You can read more about forms validation in a later chapter of this tutorial.
The finally Statement
The finally statement lets you execute code, after try and catch, regardless of the result:
Syntax
try { Block of code to try } catch(err) { Block of code to handle errors } finally { Block of code to be executed regardless of the try
Java Scope
In Java, variables are only accessible inside the region they are created. This is called scope.
Method Scope
Variables declared directly inside a method are available anywhere in the method following the line of code in which they were declared:
Exampl
public class Main {
public static void main(String[] args) {
// Code here CANNOT use x
int x = 100;
// Code here can use x
System.out.println(x);
}
}
Block Scope
A block of code refers to all of the code between curly braces {}.
Variables declared inside blocks of code are only accessible by the code between the curly braces, which follows the line in which the variable was declared:
Example
public class Main {
public static void main(String[] args) {
// Code here CANNOT use x
{ // This is a block
// Code here CANNOT use x
int x = 100;
// Code here CAN use x
System.out.println(x);
} // The block ends here
// Code here CANNOT use x
}
}
A block of code may exist on its own or it can belong to an if, while or for statement. In the case of for statements, variables declared in the statement itself are also available inside the block's scope.
JavaScript Hoisting
Hoisting is JavaScript's default behavior of moving declarations to the
top.
JavaScript Declarations are Hoisted
In JavaScript, a variable can be declared after it has been used.
In other words; a variable can be used before it has been declared.
Example 1 gives the same result as Example 2:
Example 1
x = 5; // Assign 5 to x
elem = document.getElementById("demo"); // Find an element
elem.innerHTML = x;Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â
// Display x in the element
var x; // Declare x
Example 2
var x; // Declare x
x = 5; // Assign 5 to x
elem = document.getElementById("demo"); // Find an element elem.innerHTML = x;Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â
// Display x in the element
To understand this, you have to understand the term "hoisting".
Hoisting is JavaScript's default behavior of moving all declarations to the
top of the current scope (to the top of the current script or the current function).
The let and const Keywords
Variables defined with let and const are hoisted to the top
of the block, but not initialized.
Meaning: The block of code is aware of the
variable, but it cannot be used until it has been declared.
Using a let variable before it is declared will result in a
ReferenceError .
The variable is in a "temporal dead zone" from the start
of the block until it is declared:
Example
This will result in a ReferenceError :
carName = "Volvo";
let carName;
Using a const variable before it is declared, is a syntax error,
so the code will simply not run.
Example
This code will not run.
carName = "Volvo";
const carName;
Read more about let and const in .
JavaScript Initializations are Not Hoisted
JavaScript only hoists declarations, not initializations.
Example 1 does not give the same result as
Example 2:
Example 1
var x = 5; // Initialize x var y = 7; // Initialize y
elem = document.getElementById("demo"); // Find an element
elem.innerHTML = x + " " + y;Â Â Â Â Â Â Â Â Â Â Â // Display x and y
Example 2
var x = 5; // Initialize x
elem = document.getElementById("demo"); // Find an element
elem.innerHTML = x + " " + y;Â Â Â Â Â Â Â Â Â Â Â // Display x and y
var y = 7; // Initialize y
Does it make sense that y is undefined in the last example?
This is because only the declaration (var y), not the initialization (=7) is hoisted to the top.
Because of hoisting, y has been declared before it is used, but because
initializations are not hoisted, the value of y is undefined.
Example 2 is the same as writing:
Example
var x = 5; // Initialize x
var y;Â Â Â Â // Declare y
elem = document.getElementById("demo"); // Find an element
elem.innerHTML = x + " " + y;Â Â Â Â Â Â Â Â Â Â Â // Display x and y
y = 7;Â Â Â // Assign 7 to y
Declare Your Variables At the Top !
Hoisting is (to many developers) an unknown or overlooked behavior of
JavaScript.
If a developer doesn't understand hoisting, programs may contain bugs (errors).
To avoid bugs, always declare all variables at the beginning of
every scope.
Since this is how JavaScript interprets the
code, it is always a good rule.
JavaScript in strict mode does not allow variables to be used if they are
not declared. Study "use strict" in the next chapter.
"use strict"; Defines that JavaScript code should be executed in "strict mode".
The "use strict" Directive
The "use strict" directive was new in ECMAScript version 5.
It is not a statement, but a literal expression, ignored by earlier versions of JavaScript.
The purpose of "use strict" is to indicate that the code should be executed in "strict mode".
With strict mode, you can not, for example, use undeclared variables.
All modern browsers support "use strict" except Internet Explorer 9 and lower:
|
|
|
|
|
|
"use strict"
|
13.0
|
10.0
|
4.0
|
6.0
|
12.1
|
The numbers in the table specify the first browser version that fully supports the directive.
You can use strict mode in all your programs. It helps you to write cleaner code, like preventing you from using undeclared variables.
"use strict" is just a string, so IE 9 will not throw an error even if it does not understand it.
Declaring Strict Mode
Strict mode is declared by adding "use strict"; to the beginning of a script or a function.
Declared at the beginning of a script, it has global scope (all code in the script will execute in strict mode):
Example
"use strict"; x = 3.14; // This will cause an error because x is not declared
Example
"use strict"; myFunction();
function myFunction() { y = 3.14; // This will also cause an error because y is not declared }
Declared inside a function, it has local scope (only the code inside the function is in strict mode):
x = 3.14; // This will not cause an error. myFunction();
function myFunction() { "use strict"; y = 3.14; // This will cause an error }
The "use strict"; Syntax
The syntax, for declaring strict mode, was designed to be compatible with older versions of JavaScript.
Compiling a numeric literal (4 + 5;) or a string literal ("John Doe";) in a JavaScript program has no side effects. It simply compiles to a non existing variable and dies.
So "use strict"; only matters to new compilers that "understand" the meaning of it.
Why Strict Mode?
Strict mode makes it easier to write "secure" JavaScript.
Strict mode changes previously accepted "bad syntax" into real errors.
As an example, in normal JavaScript, mistyping a variable name creates a new global variable. In strict mode, this will throw an error, making it impossible to accidentally create a global variable.
In normal JavaScript, a developer will not receive any error feedback assigning values to non-writable properties.
In strict mode, any assignment to a non-writable property, a getter-only property, a non-existing property, a non-existing variable, or a non-existing object, will throw an error.
Not Allowed in Strict Mode
Using a variable, without declaring it, is not allowed:
"use strict"; x = 3.14; // This will cause an error
Objects are variables too.
Using an object, without declaring it, is not allowed:
"use strict"; x = {p1:10, p2:20}; // This will cause an error
Deleting a variable (or object) is not allowed.
"use strict"; let x = 3.14; delete x; // This will cause an error
Deleting a function is not allowed.
"use strict"; function x(p1, p2) {}; delete x; // This will cause an error
Duplicating a parameter name is not allowed:
"use strict"; function x(p1, p1) {}; // This will cause an error
Octal numeric literals are not allowed:
"use strict"; let x = 010; // This will cause an error
Octal escape characters are not allowed:
"use strict"; let x = " 10"; // This will cause an error
Writing to a read-only property is not allowed:
"use strict"; const obj = {}; Object.defineProperty(obj, "x", {value:0, writable:false});
obj.x = 3.14; // This will cause an error
Writing to a get-only property is not allowed:
"use strict"; const obj = {get x() {return 0} };
obj.x = 3.14; // This will cause an error
Deleting an undeletable property is not allowed:
"use strict"; delete Object.prototype; // This will cause an error
The word eval cannot be used as a variable:
"use strict"; let eval = 3.14; // This will cause an error
The word arguments cannot be used as a variable:
"use strict"; let arguments = 3.14; // This will cause an error
The with statement is not allowed:
"use strict"; with (Math){x = cos(2)}; // This will cause an error
For security reasons, eval() is not allowed to create variables in the scope from which it was called.
In strict mode, a variable can not be used before it is declared:
"use strict"; eval
Example
const person = {
 firstName: "John",
  lastName : "Doe",
 id      : 5566,
 fullName : function() {
   return this.firstName + " " + this.lastName;
  }
};
What is this?
In JavaScript, the this keyword refers to an object.
Which object depends on how this is being invoked (used or called).
The this keyword refers to different objects depending on how it is used:
In an object method, this refers to the object. |
Alone, this refers to the global object. |
In a function, this refers to the global object. |
In a function, in strict mode, this is undefined . |
In an event, this refers to the element that received the event. |
Methods like call() , apply() ,
and bind() can refer this to any object. |
Note
this is not a variable. It is a keyword. You cannot change the value of this .
this in a Method
When used in an object method, this refers to the object.
In the example on top of this page, this refers to the person object.
Because the fullName method is a method of the person object.
fullName : function() {
 return this.firstName + " " + this.lastName; }
this Alone
When used alone, this refers to the global object.
Because this is running in the global scope.
In a browser window the global object is [object Window] :
 In strict mode, when used alone, this also refers to the global object:
Example
"use strict"; let x = this;
this in a Function (Default)
In a function, the global object is the default binding for this .
In a browser window the global object is [object Window] :
Example
function myFunction() {
 return this;
}
this in a Function (Strict)
JavaScript strict mode does not allow default binding.
So, when used in a function, in strict mode, this is undefined .
Example
"use strict"; function myFunction() {
 return this;
}
this in Event Handlers
In HTML event handlers, this refers to the HTML element that received the
event:
Example
<button onclick="this.style.display='none'"> Â Click to
Remove Me! </button>
Object Method Binding
In these examples, this is the person object:
Example
const person = {
 firstName : "John",
 lastName  : "Doe",
 id        : 5566,
 myFunction : function() {
   return this;   }
};
Example
const person = {
 firstName: "John",
 lastName : "Doe",
 id      : 5566,
 fullName : function() {
    return this.firstName + " " +
this.lastName; Â Â }
};
i.e. this.firstName is the firstName property of this (the person object).
Explicit Function Binding
The call() and apply() methods are predefined JavaScript methods.
They can both be used to call an object method with another object as argument.
The example below calls person1.fullName with person2 as an argument, this refers to person2,
even if fullName is a method of person1:
Example
const person1 = {
 fullName: function() {
   return this.firstName + " " + this.lastName;
  } }
const person2 = {
 firstName:"John",
 lastName: "Doe",
}
// Return "John Doe":
person1.fullName.call(person2);
Function Borrowing
With the bind() method, an object can borrow a method from another object.
This example creates 2 objects (person and member).
The member object borrows the fullname method from the person object:
Example
const person = {
  firstName:"John",
  lastName: "Doe",
 fullName: function () {
   return this.firstName + " " + this.lastName;
  }
}
const member = {
  firstName:"Hege",
  lastName: "Nilsen",
}
let fullName = person.fullName.bind(member);
This Precedence
To determine which object this refers to; use the following precedence of order.
Precedence |
Object |
1 |
bind() |
2 |
apply() and call() |
3 |
Object method |
4 |
Global scope |
Is this in a function being called using bind()?
Is this in a function being called using apply()?
Is this in a function being called using call()?
Is this in an object function (method)?
Is this in a function in the global scope.
Arrow functions were introduced in ES6.
Arrow functions allow us to write shorter function syntax:
let myFunction = (a, b) => a * b;
Before Arrow:
hello = function() { return "Hello World!"; }
With Arrow Function:
hello = () => { return "Hello World!"; }
It gets shorter! If the function has only one statement, and the statement returns a value, you can remove the brackets and the return keyword:
Arrow Functions Return Value by Default:
hello = () => "Hello World!";
Note: This works only if the function has only one statement.
If you have parameters, you pass them inside the parentheses:
Arrow Function With Parameters:
hello = (val) => "Hello " + val;
In fact, if you have only one parameter, you can skip the parentheses as well:
Arrow Function Without Parentheses:
hello = val => "Hello " + val;
What About this ?
The handling of this is also different in arrow functions compared to regular functions.
In short, with arrow functions there are no binding of this .
In regular functions the this keyword represented the object that called the function, which could be the window, the document, a button or whatever.
With arrow functions the this keyword always represents the object that defined the arrow function.
Let us take a look at two examples to understand the difference.
Both examples call a method twice, first when the page loads, and once again when the user clicks a button.
The first example uses a regular function, and the second example uses an arrow function.
The result shows that the first example returns two different objects (window and button), and the second example returns the window object twice, because the window object is the "owner" of the function.
Example
With a regular function this represents the object that calls the function:
// Regular Function: hello = function() { document.getElementById("demo").innerHTML += this; }
// The window object calls the function: window.addEventListener("load", hello);
// A button object calls the function: document.getElementById("btn").addEventListener("click", hello);
Example
With an arrow function this represents the owner of the function:
// Arrow Function: hello = () => { document.getElementById("demo").innerHTML += this; }
// The window object calls the function: window.addEventListener("load", hello);
// A button object calls the function: document.getElementById("btn").addEventListener("click", hello);
Remember these differences when you are working with functions. Sometimes the behavior of regular functions is what you want, if not, use arrow functions.
Browser Support
The following table defines the first browser versions with full support for Arrow Functions in JavaScript:
|
|
|
|
Chrome 45
|
Edge 12
|
Firefox 22
|
Safari 10
|
Opera 32
|
Sep, 2015
|
Jul, 2015
|
May, 2013
|
Sep, 2016
|
Sep, 2015
|
ECMAScript 2015, also known as ES6, introduced JavaScript Classes.
JavaScript Classes are templates for JavaScript Objects.
JavaScript Class Syntax
Use the keyword class to create a class.
Always add a method named constructor() :
Syntax
class ClassName {
 constructor() { ... }
}
Example
class Car {
 constructor(name, year) {
   this.name = name;
   this.year = year;
 }
}
The example above creates a class named "Car".
The class has two initial properties: "name" and "year".
A JavaScript class is not an object.
It is a template for JavaScript objects.
Using a Class
When you have a class, you can use the class to create objects:
Example
const myCar1 = new Car("Ford", 2014);
const myCar2 = new Car("Audi", 2019);
The example above uses the Car class to create two Car objects.
The constructor method is called automatically when a new object is created.
The Constructor Method
The constructor method is a special method:
- It has to have the exact name "constructor"
- It is executed automatically when a new object is created
- It is used to initialize object properties
If you do not define a constructor method, JavaScript
will add an empty constructor method.
Class Methods
Class methods are created with the same syntax as object methods.
Use the keyword class to create a class.
Always add a constructor() method.
Then add any number of methods.
Syntax
class ClassName {
 constructor() { ... }
  method_1() { ... }
  method_2() { ... }
  method_3() { ... }
}
Create a Class method named "age", that returns the Car age:
Example
class Car {
 constructor(name, year) {
   this.name = name;
   this.year = year;
 }  age() {
   const date = new Date();
   return date.getFullYear() - this.year;
 } }
const myCar = new Car("Ford", 2014);
document.getElementById("demo").innerHTML =
"My car is " + myCar.age() + " years old.";
You can send parameters to Class methods:
Example
class Car { Â constructor(name, year) { Â Â Â
this.name = name; Â Â Â this.year = year; Â } Â
age(x) { Â Â Â return x - this.year; Â } }
const date = new Date();
let year = date.getFullYear();
const myCar = new
Car("Ford", 2014); document.getElementById("demo").innerHTML= "My car is
" + myCar.age(year) + " years old.";
Browser Support
The following table defines the first browser version with full support for
Classes in JavaScript:
|
|
|
|
|
Chrome 49 |
Edge 12 |
Firefox 45 |
Safari 9 |
Opera 36 |
Mar, 2016 |
Jul, 2015 |
Mar, 2016 |
Oct, 2015 |
Mar, 2016 |
You will learn a lot more about JavaScript Classes later in this tutorial.
Modules
JavaScript modules allow you to break up your code into separate files.
This makes it easier to maintain a code-base.
Modules are imported from external files with the import statement.
Modules also rely on type="module" in the <script> tag.
Example
<script type="module">
import message from "./message.js";
</script>
Export
Modules with functions or variables can be stored in any external file.
There are two types of exports: Named Exports and Default Exports.
Named Exports
Let us create a file named person.js , and
fill it with the things we want to export.
You can create named exports two ways. In-line individually, or all at once at the bottom.
In-line individually:
person.js
export const name = "Jesse";
export const age = 40;
All at once at the bottom:
person.js
const name = "Jesse";
const age = 40;
export {name, age};
Default Exports
Let us create another file, named message.js , and
use it for demonstrating default export.
You can only have one default export in a file.
Example
message.js
const message = () => {
const name = "Jesse";
const age = 40;
return name + ' is ' + age + 'years old.';
};
export default message;
Import
You can import modules into a file in two ways, based on if they are named
exports or default exports.
Named exports are constructed using curly braces. Default exports are not.
Import from named exports
Import named exports from the file person.js:
import { name, age } from "./person.js";
Import from default exports
Import a default export from the file message.js:
import message from "./message.js";
Note
Modules only work with the HTTP(s) protocol.
A web-page opened via the file:// protocol cannot use import / export.
JavaScript JSON
JSON is a format for storing and transporting data.
JSON is often used when data is sent from a server to a web
page.
What is JSON?
- JSON stands for JavaScript Object Notation
- JSON is a lightweight data interchange format
- JSON is language independent *
- JSON is "self-describing" and easy to understand
* The JSON syntax is derived from JavaScript object notation syntax, but the JSON format is text only.
Code for reading and generating JSON data can be written in any programming
language.
JSON Example
This JSON syntax defines an employees object: an array of 3 employee records (objects):
JSON Example
{ "employees":[
  {"firstName":"John", "lastName":"Doe"},
  {"firstName":"Anna", "lastName":"Smith"},
 {"firstName":"Peter", "lastName":"Jones"}
] }
The JSON Format Evaluates to JavaScript Objects
The JSON format is syntactically identical to the code for creating
JavaScript objects.
Because of this similarity, a JavaScript program
can easily convert JSON data into native
JavaScript objects.
JSON Syntax Rules
- Data is in name/value pairs
- Data is separated by commas
- Curly braces hold objects
- Square brackets hold arrays
JSON Data - A Name and a Value
JSON data is written as name/value pairs, just like JavaScript object
properties.
A name/value pair consists of a field name (in double quotes),
followed by a colon, followed by a value:
JSON names require double quotes. JavaScript names do not.
JSON Objects
JSON objects are written inside curly braces.
Just like in JavaScript, objects can contain multiple name/value pairs:
{"firstName":"John", "lastName":"Doe"}
JSON Arrays
JSON arrays are written inside square brackets.
Just like in JavaScript, an array can contain objects:
"employees":[
Â
{"firstName":"John", "lastName":"Doe"},
Â
{"firstName":"Anna", "lastName":"Smith"},
Â
{"firstName":"Peter", "lastName":"Jones"}
]
In the example above, the object "employees" is an array. It contains three
objects.
Each object is a record of a person (with a first name and a last name).
Converting a JSON Text to a JavaScript Object
A common use of JSON is to read data from a web server,
and display the data in a web page.
For simplicity, this can be demonstrated using a string as input.
First, create a JavaScript string containing JSON syntax:
let text = '{ "employees" : [' +
'{ "firstName":"John" , "lastName":"Doe" },' +
'{ "firstName":"Anna" , "lastName":"Smith" },' +
'{ "firstName":"Peter" , "lastName":"Jones" } ]}';
Then, use the JavaScript built-in function JSON.parse() to convert the string into a JavaScript object:
const obj = JSON.parse(text);
Finally, use the new JavaScript object in your page:
Example
<p id="demo"></p>
<script>
document.getElementById("demo").innerHTML =
obj.employees[1].firstName + " " + obj.employees[1].lastName;
</script>
You can read more about JSON in our .
JavaScript Debugging
Errors can (will) happen, every time you write some new computer code.
Code Debugging
Programming code might contain syntax errors, or logical errors.
Many of these errors are difficult to diagnose.
Often, when programming code contains errors, nothing will happen. There are
no error messages, and you will get no indications where to search for errors.
Searching for (and fixing) errors in programming code is called code debugging.
JavaScript Debuggers
Debugging is not easy. But fortunately, all modern browsers have a built-in
JavaScript debugger.
Built-in debuggers can be turned on and off, forcing errors to be reported to
the user.
With a debugger, you can also set breakpoints (places where code execution
can be stopped), and examine variables while the code is executing.
Normally (otherwise follow the steps at the bottom of this page), you activate debugging in your browser with
the F12 key, and select "Console" in the debugger menu.
The console.log() Method
If your browser supports debugging, you can use console.log() to
display JavaScript values in the debugger window:
Example
<!DOCTYPE html> <html> <body>
<h1>My First Web Page</h1>
<script> a = 5; b = 6; c = a + b; console.log(c); </script>
</body> </html>
Tip: Read more about the console.log() method in our .
Setting Breakpoints
In the debugger window, you can set breakpoints in the JavaScript code.
At each breakpoint, JavaScript will stop executing, and let you examine
JavaScript values.
After examining values, you can resume the execution of code (typically with
a play button).
The debugger Keyword
The debugger keyword stops the execution of JavaScript,
and calls (if available) the debugging function.
This has the same function as setting a breakpoint in the debugger.
If no debugging is available, the debugger statement has no effect.
With the debugger turned on, this code will stop executing before it
executes the third line.
Example
let x = 15 * 5; debugger; document.getElementById("demo").innerHTML = x;
Major Browsers' Debugging Tools
Normally, you activate debugging in your browser with F12, and select "Console" in the debugger menu.
Otherwise follow these steps:
Chrome
- Open the browser.
- From the menu, select "More tools".
- From tools, choose "Developer tools".
- Finally, select Console.
Firefox
- Open the browser.
- From the menu, select "Web Developer".
- Finally, select "Web Console".
Edge
- Open the browser.
- From the menu, select "Developer Tools".
- Finally, select "Console".
Opera
- Open the browser.
- From the menu, select "Developer".
- From "Developer", select "Developer tools".
- Finally, select "Console".
Safari
- Go to Safari, Preferences, Advanced in the main menu.
- Check "Enable Show Develop menu in menu bar".
- When the new option "Develop" appears in the menu:
Choose "Show Error
Console".
Did You Know?
Debugging is the process of testing, finding, and reducing bugs (errors) in computer programs.
The first known computer bug was a real bug (an insect) stuck in the electronics.
Always use the same coding conventions for all your JavaScript
projects.
JavaScript Coding Conventions
Coding conventions are style guidelines for programming.
They typically cover:
- Naming and declaration rules for variables and functions.
- Rules for the use of white space, indentation, and comments.
- Programming practices and principles.
Coding conventions secure quality:
- Improve code readability
- Make code maintenance easier
Coding conventions can be documented rules for teams to follow, or just be your individual coding practice.
This page describes the general JavaScript code conventions used by W3Schools.
You should also read the next chapter "Best Practices", and learn how to avoid coding pitfalls.
Variable Names
At W3schools we use camelCase for identifier names (variables and functions).
All names start with a letter.
At the bottom of this page, you will find a wider discussion about naming
rules.
firstName = "John"; lastName = "Doe";
price = 19.90;
tax = 0.20;
fullPrice = price + (price * tax);
Spaces Around Operators
Always put spaces around operators ( = + - * / ), and after commas:
Examples:
let x = y + z;
const myArray = ["Volvo", "Saab",
"Fiat"];
Code Indentation
Always use 2 spaces for indentation of code blocks:
Functions:
function toCelsius(fahrenheit) {
 return (5 / 9) * (fahrenheit - 32);
}
Do not use tabs (tabulators) for indentation. Different editors interpret tabs differently.
Statement Rules
General rules for simple statements:
- Always end a simple statement with a semicolon.
Examples:
const cars = ["Volvo", "Saab",
"Fiat"];
const person = { Â firstName: "John", Â
lastName: "Doe", Â age: 50, Â eyeColor:
"blue" };
General rules for complex (compound) statements:
- Put the opening bracket at the end of the first line.
- Use one space before the opening bracket.
- Put the closing bracket on a new line, without leading spaces.
- Do not end a complex statement with a semicolon.
Functions:
function toCelsius(fahrenheit) {
  return (5 / 9) * (fahrenheit - 32);
}
Loops:
for (let i = 0; i < 5; i++) { Â Â x += i; }
Conditionals:
if (time < 20) { Â Â greeting = "Good day"; } else {
Â
greeting = "Good evening"; }
Object Rules
General rules for object definitions:
- Place the opening bracket on the same line as the object name.
- Use colon plus one space between each property and its value.
- Use quotes around string values, not around numeric values.
- Do not add a comma after the last property-value pair.
- Place the closing bracket on a new line, without
leading spaces.
- Always end an object definition with a semicolon.
Example
const person = { Â firstName: "John", Â
lastName: "Doe", Â age: 50, Â eyeColor:
"blue" };
Short objects can be written compressed, on one line, using spaces only
between properties, like this:
const person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
Line Length < 80
For readability, avoid lines longer than 80
characters.
If a JavaScript statement does not fit on one line, the best place to break
it, is after an operator or a comma.
Example
document.getElementById("demo").innerHTML =
"Hello Dolly.";
Naming Conventions
Always use the same naming convention for all your code. For example:
- Variable and function names written as camelCase
- Global variables written in UPPERCASE (We don't, but it's
quite common)
- Constants (like PI) written in UPPERCASE
Should you use hyp-hens, camelCase, or
under_scores in variable names?
This is a question programmers often discuss. The answer depends on who you
ask:
Hyphens in HTML and CSS:
HTML5 attributes can start with data- (data-quantity, data-price).
CSS uses hyphens in property-names (font-size).
Hyphens can be mistaken as subtraction attempts. Hyphens are not allowed in JavaScript names.
Underscores:
Many programmers prefer to use underscores (date_of_birth), especially in SQL
databases.
Underscores are often used in PHP documentation.
PascalCase:
PascalCase is often preferred by C programmers.
camelCase:
camelCase is used by JavaScript itself, by jQuery, and other JavaScript
libraries.
Do not start names with a $ sign. It will put you in conflict with many JavaScript library names.
Loading JavaScript in HTML
Use simple syntax for loading external scripts (the type attribute is not
necessary):
<script src="myscript.js"></script>
Accessing HTML Elements
A consequence of using "untidy" HTML styles, might result in JavaScript errors.
These two JavaScript statements will produce different results:
const obj = getElementById("Demo")
const obj = getElementById("demo")
If possible, use the same naming convention (as JavaScript) in HTML.
.
File Extensions
HTML files should have a .html extension (.htm is allowed).
CSS files should have a .css extension.
JavaScript files should have a .js extension.
Use Lower Case File Names
Most web servers (Apache, Unix) are case sensitive about file names:
london.jpg cannot be accessed as London.jpg.
Other web servers (Microsoft, IIS) are not case sensitive:
london.jpg can be accessed as London.jpg or london.jpg.
If you use a mix of upper and lower case, you have to be extremely
consistent.
If you move from a case insensitive, to a case sensitive server, even small
errors can break your web site.
To avoid these problems, always use lower case file names (if possible).
Performance
Coding conventions are not used by computers. Most rules have
little impact on the execution of programs.
Indentation and extra spaces are not significant in small scripts.
For code in development, readability should be preferred. Larger production
scripts should be minimized.
JavaScript Best Practices
Avoid global variables, avoid new , avoid == , avoid eval()
Avoid Global Variables
Minimize the use of global variables.
This includes all data types, objects, and functions.
Global variables and functions can be overwritten by other scripts.
Use local variables instead, and learn how to use
.
Always Declare Local Variables
All variables used in a function should be declared as local variables.
Local variables must be declared with the var ,
the let , or the const keyword,
otherwise they will become global variables.
Strict mode does not allow undeclared variables.
Declarations on Top
It is a good coding practice to put all declarations at the top of each script
or function.
This will:
- Give cleaner code
- Provide a single place to look for local variables
- Make it easier to avoid unwanted (implied) global variables
- Reduce the possibility of unwanted re-declarations
// Declare at the beginning
let firstName, lastName, price, discount, fullPrice;
// Use later firstName = "John"; lastName = "Doe";
price = 19.90;
discount = 0.10;
fullPrice = price - discount;
This also goes for loop variables:
for (let i = 0; i < 5; i++)
{
Initialize Variables
It is a good coding practice to initialize variables when you declare them.
This will:
- Give cleaner code
- Provide a single place to initialize variables
- Avoid undefined values
// Declare and initiate at the beginning
let firstName = "";
let lastName = "";
let price = 0;
let discount = 0;
let fullPrice = 0,
const myArray = [];
const myObject = {};
Initializing variables provides an idea of the intended use (and intended data type).
Declare Objects with const
Declaring objects with const will prevent any accidental change of type:
Example
let car = {type:"Fiat", model:"500", color:"white"};
car = "Fiat";Â Â Â Â Â Â // Changes object to string
const car = {type:"Fiat", model:"500", color:"white"};
car = "Fiat";Â Â Â Â Â Â // Not possible
Declare Arrays with const
Declaring arrays with const will prevent any accidential change of type:
Example
let cars = ["Saab", "Volvo", "BMW"];
cars = 3;Â Â Â Â // Changes array to number
const cars = ["Saab", "Volvo", "BMW"];
cars = 3;Â Â Â Â // Not possible
Don't Use new Object()
- Use
"" instead of new String()
- Use
0 instead of new Number()
- Use
false instead of new Boolean()
- Use
{} instead of new Object()
- Use
[] instead of new Array()
- Use
/()/ instead of new RegExp()
- Use
function (){} instead of new Function()
Example
let x1 = "";Â Â Â Â Â Â Â Â Â Â Â Â // new primitive string
let x2 = 0;Â Â Â Â Â Â Â Â Â Â Â Â Â // new primitive number
let x3 = false;Â Â Â Â Â Â Â Â Â // new primitive boolean
const x4 = {};Â Â Â Â Â Â Â Â Â Â // new object
const x5 = [];Â Â Â Â Â Â Â Â Â Â // new array object
const x6 = /()/;Â Â Â Â Â Â Â Â // new regexp object
const x7 = function(){};Â // new function object
Beware of Automatic Type Conversions
JavaScript is loosely typed.
A variable can contain all data types.
A variable can change its data type:
Example
let x = "Hello";Â Â Â Â Â // typeof x is a string
x = 5;Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â // changes typeof x to a number
Beware that numbers can accidentally be converted to strings or NaN (Not a
Number).
When doing mathematical operations, JavaScript can convert numbers to strings:
Example
let x = 5 + 7;      // x.valueOf() is 12, typeof x is a number
let x = 5 + "7";    // x.valueOf() is 57, typeof x is a string
let x = "5" + 7;    // x.valueOf() is 57, typeof x is a string
let x = 5 - 7;      // x.valueOf() is -2, typeof x is a number
let x = 5 - "7";    // x.valueOf() is -2, typeof x is a number
let x = "5" - 7;    // x.valueOf() is -2, typeof x is a number
let x = 5 - "x";Â Â Â // x.valueOf() is NaN, typeof x is a number
Subtracting a string from a string, does not
generate an error but returns NaN (Not a Number):
Example
"Hello" - "Dolly" Â Â // returns NaN
Use === Comparison
The == comparison operator always converts (to matching types) before
comparison.
The === operator forces comparison of values and type:
Example
0 == "";Â Â Â Â Â Â Â // true
1 == "1"; Â Â Â Â Â // true
1 == true;Â Â Â Â Â // true
0 === "";Â Â Â Â Â Â // false
1 === "1"; Â Â Â // false
1 === true;Â Â Â Â
// false
Use Parameter Defaults
If a function is called with a missing argument, the value of the missing
argument is set to
undefined .
Undefined values can break your code. It is a good habit to assign default
values to arguments.
Example
function myFunction(x, y) {
 if (y === undefined) {
   y = 0;
 }
}
allows default parameters in the function definition:
function (a=1, b=1) {Â /*function code*/ }
Read more about function parameters and arguments at
End Your Switches with Defaults
Always end your switch statements with a default . Even if you think there is
no need for it.
Example
switch (new Date().getDay()) {
Â
case 0:
  day = "Sunday";
   break;
  case 1:
  day = "Monday";
   break;
  case 2:
  day = "Tuesday";
   break;
Â
case 3:
  day = "Wednesday";
   break;
Â
case 4:
   day = "Thursday";
   break;
  case 5:
  day = "Friday";
   break;
  case 6:
  day = "Saturday";
   break;
  default:    day =
"Unknown"; }
Avoid Number, String, and Boolean as Objects
Always treat numbers, strings, or booleans as primitive values. Not as
objects.
Declaring these types as objects, slows down execution speed,
and produces nasty side effects:
Example
let x = "John";Â Â Â Â Â Â Â Â Â Â Â Â Â
let y = new String("John");
(x === y) // is false because x is a string and y is an object.
Or even worse:
Example
let x = new String("John");Â Â Â Â Â Â Â Â Â Â Â Â Â
let y = new String("John");
(x == y) // is false because you cannot compare objects.
Avoid Using eval()
The eval() function is used to run text as code. In almost all cases, it
should not be necessary to use it.
Because it allows arbitrary code to be run, it also represents a security
problem.
JavaScript Common Mistakes
This chapter points out some common JavaScript mistakes.
Accidentally Using the Assignment Operator
JavaScript programs may generate unexpected results if a programmer
accidentally uses an assignment operator (= ), instead of a comparison operator
(== ) in an if statement.
This if statement returns false (as
expected) because x is
not equal to 10:
This if statement returns true (maybe not
as expected), because 10 is
true:
This if statement returns false (maybe not
as expected), because 0 is
false:
An assignment always returns the value of the assignment.
Expecting Loose Comparison
In regular comparison, data type does not matter. This if statement returns
true:
let x = 10;
let y = "10"; if (x == y)
In strict comparison, data type does matter. This if statement returns false:
let x = 10;
let y = "10"; if (x === y)
It is a common mistake to forget that switch statements use strict
comparison:
This case switch will display an alert:
let x = 10;
switch(x) { Â Â case 10: alert("Hello");
}
This case switch will not display an alert:
let x = 10; switch(x) { Â case "10": alert("Hello");
}
Confusing Addition & Concatenation
Addition is about adding numbers.
Concatenation is about adding strings.
In JavaScript both operations use the same + operator.
Because of this, adding a number as a number will produce a different
result from adding a number as a string:
let x = 10;
x = 10 + 5;Â Â Â Â Â Â //
Now x is 15
let y = 10;
y += "5";Â Â Â Â Â Â Â
// Now y is "105"
When adding two variables, it can be difficult to anticipate the result:
let x = 10;
let y = 5;
let z = x + y;Â Â Â Â Â // Now z is 15
let x = 10;
let y = "5";
let z = x + y;Â Â Â Â // Now z is "105"
Misunderstanding Floats
All numbers in JavaScript are stored as 64-bits Floating point numbers
(Floats).
All programming languages, including JavaScript, have difficulties with
precise floating point values:
let x = 0.1;
let y = 0.2; let z = x + y          Â
// the result in z will not be 0.3
To solve the problem above, it helps to multiply and divide:
Example
let z = (x * 10 + y * 10) / 10;Â Â Â Â Â Â // z will be 0.3
Breaking a JavaScript String
JavaScript will allow you to break a statement into two lines:
Example 1
let x = "Hello World!";
But, breaking a statement in the middle of a string will not work:
Example 2
let x = "Hello World!";
You must use a "backslash" if you must break a statement in a string:
Example 3
let x = "Hello World!";
Misplacing Semicolon
Because of a misplaced semicolon, this code block will execute regardless of
the value of x:
if (x == 19); {
 // code block }
Breaking a Return Statement
It is a default JavaScript behavior to close a statement automatically at the
end of a line.
Because of this, these two examples will return the same result:
Example 1
function myFunction(a) {
 let power = 10  return a * power }
Example 2
function myFunction(a) {
 let power = 10;  return a * power; }
JavaScript will also allow you to break a statement into two lines.
Because of this, example 3 will also return the same result:
Example 3
function myFunction(a) {
 let   power = 10;   return a * power; }
But, what will happen if you break the return statement in two lines like
this:
Example 4
function myFunction(a) {
 let  power = 10;  return  a * power; }
The function will return undefined !
Why? Because JavaScript thought you meant:
Example 5
function myFunction(a) {
 let  power = 10;   return;  a * power; }
Explanation
If a statement is incomplete like:
JavaScript will try to complete the statement by reading the next line:
But since this statement is complete:
JavaScript will automatically close it like this:
This happens because closing (ending) statements with semicolon is optional in
JavaScript.
JavaScript will close the return statement at the end of the line, because
it is a complete statement.
Never break a return statement.
Accessing Arrays with Named Indexes
Many programming languages support arrays with named indexes.
Arrays with named indexes are called associative
arrays (or hashes).
JavaScript does not support arrays with named indexes.
In JavaScript, arrays use numbered indexes:Â Â
Example
const person = [];
person[0] = "John";
person[1] = "Doe";
person[2] = 46; person.length;Â Â Â Â Â Â
// person.length will return 3 person[0];Â Â Â Â Â Â Â Â Â Â
// person[0] will return "John"
In JavaScript, objects use named indexes.
If you use a named index, when accessing an array, JavaScript will redefine
the array to a standard object.
After the automatic redefinition, array methods and properties will produce undefined or
incorrect results:
Example:
const person = [];
person["firstName"] = "John";
person["lastName"] = "Doe";
person["age"] = 46; person.length;Â Â Â Â Â // person.length will
return 0 person[0];Â Â Â Â Â Â Â Â Â
// person[0] will return undefined
Ending Definitions with a Comma
Trailing commas in object and array definition are legal in ECMAScript 5.
Object Example:
person = {firstName:"John", lastName:"Doe", age:46,}
Array Example:
points = [40, 100, 1, 5, 25, 10,];
WARNING !!
Internet Explorer 8 will crash.
JSON does not allow trailing commas.
JSON:
person = {"firstName":"John", "lastName":"Doe", "age":46}
JSON:
points = [40, 100, 1, 5, 25, 10];
Undefined is Not Null
JavaScript objects, variables, properties, and methods can be undefined .
In addition, empty JavaScript objects can have the value null .
This can make it a little bit difficult to test if an object is empty.
You can test if an object exists by testing if the type is undefined :
Example:
if (typeof myObj === "undefined")Â
But you cannot test if an object is null , because this will throw an error if the
object is undefined :
Incorrect:
if (myObj === null)Â
To solve this problem, you must test if an object is not null ,
and not undefined .
But this can still throw an error:
Incorrect:
if (myObj !== null && typeof myObj
!== "undefined")Â
Because of this, you must test for not undefined before you can
test for not null :
Correct:
if (typeof myObj !== "undefined" && myObj !== null)Â
JavaScript Performance
How to speed up your JavaScript code.
Reduce Activity in Loops
Loops are often used in programming.
Each statement in a loop, including the for statement, is executed for each iteration of the
loop.
Statements or assignments that can be placed outside the loop will make the
loop run faster.
Bad:
for (let i = 0; i < arr.length; i++) {
Better Code:
let l = arr.length;
for (let i = 0; i < l; i++) {
The bad code accesses the length property of an array each time the loop is
iterated.
The better code accesses the length property outside the loop and makes the
loop run faster.
Reduce DOM Access
Accessing the HTML DOM is very slow, compared to other JavaScript statements.
If you expect to access a DOM element several times, access it once, and use
it as a local variable:
Example
const obj = document.getElementById("demo"); obj.innerHTML = "Hello";
Reduce DOM Size
Keep the number of elements in the HTML DOM small.
This will always
improve page loading, and speed up rendering (page display), especially on smaller devices.
Every attempt to search the DOM (like getElementsByTagName) will benefit
from a smaller DOM.
Avoid Unnecessary Variables
Don't create new variables if you don't plan to save values.
Often you can replace code like this:
let fullName = firstName + " " + lastName;
document.getElementById("demo").innerHTML = fullName;
With this:
document.getElementById("demo").innerHTML = firstName + " " + lastName;
Delay JavaScript Loading
Putting your scripts at the bottom of the page body lets the browser load the
page first.
While a script is downloading, the browser will not start any other
downloads. In addition all parsing and rendering activity might be blocked.
The HTTP specification defines that browsers should not download more than
two components in parallel.
An alternative is to use defer="true" in the script tag. The
defer attribute specifies that the script should be executed after the page has
finished parsing, but it only works for external scripts.
If possible, you can add your script to the page by code, after the page has loaded:
Example
<script> window.onload = function() { Â
const element = document.createElement("script"); Â
element.src = "myScript.js"; Â
document.body.appendChild(element); }; </script>
Avoid Using with
Avoid using the with keyword. It has a negative effect on
speed. It also clutters up JavaScript scopes.
The with keyword is not allowed in strict mode.
In JavaScript you cannot use these reserved words as variables, labels, or function names:
abstract
|
arguments
|
await*
|
boolean
|
break
|
byte
|
case
|
catch
|
char
|
class*
|
const*
|
continue
|
debugger
|
default
|
delete
|
do
|
double
|
else
|
enum*
|
eval
|
export*
|
extends*
|
false
|
final
|
finally
|
float
|
for
|
function
|
goto
|
if
|
implements
|
import*
|
in
|
instanceof
|
int
|
interface
|
let*
|
long
|
native
|
new
|
null
|
package
|
private
|
protected
|
public
|
return
|
short
|
static
|
super*
|
switch
|
synchronized
|
this
|
throw
|
throws
|
transient
|
true
|
try
|
typeof
|
var
|
void
|
volatile
|
while
|
with
|
yield
|
Words marked with* was new in ECMAScript 5 and ECMAScript 6.
You can read more about the different JavaScript versions in the chapter JS Versions.
Removed Reserved Words
The following reserved words have been removed from the ECMAScript 5/6 standard:
abstract
|
boolean
|
byte
|
char
|
double
|
final
|
float
|
goto
|
int
|
long
|
native
|
short
|
synchronized
|
throws
|
transient
|
volatile
|
Do not use these words as variables. ECMAScript 5/6 does not have full support in all browsers.
JavaScript Objects, Properties, and Methods
You should also avoid using the name of JavaScript built-in objects, properties, and methods:
Array
|
Date
|
eval
|
function
|
hasOwnProperty
|
Infinity
|
isFinite
|
isNaN
|
isPrototypeOf
|
length
|
Math
|
NaN
|
name
|
Number
|
Object
|
prototype
|
String
|
toString
|
undefined
|
valueOf
|
Java Reserved Words
JavaScript is often used together with Java. You should avoid using some Java objects and properties as JavaScript identifiers:
getClass
|
java
|
JavaArray
|
javaClass
|
JavaObject
|
JavaPackage
|
|
|
Other Reserved Words
JavaScript can be used as the programming language in many applications.
You should also avoid using the name of HTML and Window objects and properties:
alert
|
all
|
anchor
|
anchors
|
JavaScript was invented by Brendan Eich in 1995, and became an ECMA standard in 1997.
ECMAScript is the official name of the language.
ECMAScript versions have been abbreviated to ES1, ES2, ES3, ES5, and ES6.
Since 2016, versions are named by year (ECMAScript 2016, 2017, 2018, 2019, 2020).
ECMAScript Editions
Ver
|
Official Name
|
Description
|
ES1
|
ECMAScript 1 (1997)
|
First edition
|
ES2
|
ECMAScript 2 (1998)
|
Editorial changes
|
ES3
|
ECMAScript 3 (1999)
|
Added regular expressions Added try/catch Added switch Added do-while
|
ES4
|
ECMAScript 4
|
Never released
|
ES5
|
ECMAScript 5 (2009)
?
|
Added "strict mode" Added JSON support Added String.trim() Added Array.isArray() Added Array iteration methods Allows trailing commas for object literals
|
ES6
|
ECMAScript 2015
?
|
Added let and const Added default parameter values Added Array.find() Added Array.findIndex()
|
|
ECMAScript 2016
?
|
Added exponential operator (**) Added Array.includes()
|
|
ECMAScript 2017
?
|
Added string padding Added Object.entries() Added Object.values() Added async functions Added shared memory Allows trailing commas for function parameters
|
|
ECMAScript 2018
?
|
Added rest / spread properties Added asynchronous iteration Added Promise.finally() Additions to RegExp
|
|
ECMAScript 2019
?
|
String.trimStart() String.trimEnd() Array.flat() Object.fromEntries Optional catch binding
|
|
ECMAScript 2020
?
|
The Nullish Coalescing Operator (??)
|
This tutorial covers every version of JavaScript:
· The Original JavaScript ES1 ES2 ES3 (1997-1999)
· The First Main Revision ES5 (2009)
· The Second Revision ES6 (2015)
· Yearly Additions (2016, 2017, 2018, 2019, 2020)
Browser Support
ECMAScript 1 - 6 is fully supported in all modern browsers.
Browser Support for ES5 (2009)
Browser
|
Version
|
From Date
|
Chrome
|
23
|
Nov 2012
|
Firefox
|
21
|
May 2013
|
IE
|
9*
|
Mar 2011
|
IE / Edge
|
10
|
Sep 2012
|
Safari
|
6
|
Jul 2012
|
Opera
|
15
|
Jul 2013
|
* Internet Explorer 9 does not support ECMAScript 5 "use strict".
Browser Support for ES6 (2015)
Browser
|
Version
|
Date
|
Chrome
|
51
|
May 2016
|
Firefox
|
52
|
Mar 2017
|
Edge
|
14
|
Aug 2016
|
Safari
|
10
|
Sep 2016
|
Opera
|
38
|
Jun 2016
|
Internet Explorer does not support ECMAScript 2015.
Browser Support for ECMAScript 2016
Browser
|
Version
|
Date
|
Chrome
|
52
|
Jul 2016
|
Firefox
|
54
|
Jun 2017
|
Edge
|
14
|
Aug 2016
|
Safari
|
10.1
|
Mar 2017
|
Opera
|
39
|
Aug 2016
|
ECMAScript 2009, also known as ES5, was the first major revision to JavaScript.
This chapter describes the most important features of ES5.
ES5 Features
- "use strict"
- String[number] access
- Multiline strings
- String.trim()
- Array.isArray()
- Array forEach()
- Array map()
- Array filter()
- Array reduce()
- Array reduceRight()
- Array every()
- Array some()
- Array indexOf()
- Array lastIndexOf()
- JSON.parse()
- JSON.stringify()
- Date.now()
- Date toISOString()
- Date toJSON()
- Property getters and setters
- Reserved words as property names
- Object methods
- Object defineProperty()
- Function bind()
- Trailing commas
Browser Support
ES5 (JavaScript 2009) fully supported in all modern browsers since July 2013:
|
|
|
|
|
Chrome 23
|
IE/Edge 10
|
Firefox 21
|
Safari 6
|
Opera 15
|
Sep 2012
|
Sep 2012
|
Apr 2013
|
Jul 2012
|
Jul 2013
|
The "use strict" Directive
"use strict" defines that the JavaScript code should be executed in "strict mode".
With strict mode you can, for example, not use undeclared variables.
You can use strict mode in all your programs. It helps you to write cleaner code, like preventing you from using undeclared variables.
"use strict" is just a string expression. Old browsers will not throw an error if they don't understand it.
Read more in JS Strict Mode.
Property Access on Strings
The charAt() method returns the character at a specified index (position) in a string:
Example
var str = "HELLO WORLD"; str.charAt(0); // returns H
- »
ES5 allows property access on strings:
Example
var str = "HELLO WORLD"; str[0]; // returns H
- »
Property access on string might be a little unpredictable.
Read more in JS String Methods.
Strings Over Multiple Lines
ES5 allows string literals over multiple lines if escaped with a backslash:
Example
"Hello Dolly!";
- »
The method might not have universal support. Older browsers might treat the spaces around the backslash differently. Some older browsers do not allow spaces behind the character.
A safer way to break up a string literal, is to use string addition:
Example
"Hello " + "Dolly!";
- »
Reserved Words as Property Names
ES5 allows reserved words as property names:
Object Example
var obj = {name: "John", new: "yes"}
- »
String trim()
The trim() method removes whitespace from both sides of a string.
Example
var str = " Hello World! "; alert(str.trim());
- »
Read more in JS String Methods.
Array.isArray()
The isArray() method checks whether an object is an array.
Example
function myFunction() { var fruits = ["Banana", "Orange", "Apple", "Mango"]; var x = document.getElementById("demo"); x.innerHTML = Array.isArray(fruits); }
- »
Read more in JS Arrays.
Array forEach()
The forEach() method calls a function once for each array element.
Example
var txt = ""; var numbers = [45, 4, 9, 16, 25]; numbers.forEach(myFunction);
function myFunction(value) { txt = txt + value + "<br>"; }
- »
Learn more in JS Array Iteration Methods.
Array map()
This example multiplies each array value by 2:
Example
var numbers1 = [45, 4, 9, 16, 25]; var numbers2 = numbers1.map(myFunction);
ECMAScript 2015 was the second major revision to JavaScript.
ECMAScript 2015 is also known as ES6 and ECMAScript 6.
This chapter describes the most important features of ES6.
New Features in ES6
- The let keyword
- The const keyword
- Arrow Functions
- The ... Operator
- For/of
- Map Objects
- Set Objects
- Classes
- Promises
- Symbol
- Default Parameters
- Function Rest Parameter
- String.includes()
- String.startsWith()
- String.endsWith()
- Array.from()
- Array keys()
- Array find()
- Array findIndex()
- New Math Methods
- New Number Properties
- New Number Methods
- New Global Methods
- Object entries
- JavaScript Modules
Browser Support for ES6 (2015)
ES6 is fully supported in all modern browsers since June 2017:
|
|
|
|
|
Chrome 51
|
Edge 15
|
Firefox 54
|
Safari 10
|
Opera 38
|
May 2016
|
Apr 2017
|
Jun 2017
|
Sep 2016
|
Jun 2016
|
ES6 is not supported in Internet Explorer.
JavaScript let
The let keyword allows you to declare a variable with block scope.
Example
var x = 10; // Here x is 10 { let x = 2; // Here x is 2 } // Here x is 10
- »
Read more about let in the chapter: JavaScript Let.
JavaScript const
The const keyword allows you to declare a constant (a JavaScript variable with a constant value).
Constants are similar to let variables, except that the value cannot be changed.
Example
var x = 10; // Here x is 10 { const x = 2; // Here x is 2 } // Here x is 10
- »
Read more about const in the chapter: JavaScript Const.
Arrow Functions
Arrow functions allows a short syntax for writing function expressions.
You don't need the function keyword, the return keyword, and the curly brackets.
Example
// ES5 var x = function(x, y) { return x * y; }
// ES6 const x = (x, y) => x * y;
- »
Arrow functions do not have their own this. They are not well suited for defining object methods.
Arrow functions are not hoisted. They must be defined before they are used.
Using const is safer than using var, because a function expression is always a constant value.
You can only omit the return keyword and the curly brackets if the function is a single statement. Because of this, it might be a good habit to always keep them:
Example
const x = (x, y) => { return x * y };
- »
Learn more about Arrow Functions in the chapter: JavaScript Arrow Function.
The Spread (...) Operator
The ... operator expands an iterable (like an array) into more elements:
Example
const q1 = ["Jan", "Feb", "Mar"]; const q2 = ["Apr", "May", "Jun"]; const q3 = ["Jul", "Aug", "Sep"]; const q4 = ["Oct", "Nov", "May"];
const year = [...q1, ...q2, ...q3, ...q4];
- »
The ... operator can be used to expand an iterable into more arguments for function calls:
Example
const numbers = [23,55,21,87,56]; let maxValue = Math.max(...numbers);
- »
The For/Of Loop
The JavaScript for/of statement loops through the values of an iterable objects.
for/of
JavaScript Version Numbers
Old ECMAScript versions was named by numbers: ES5 and ES6.
From 2016, versions are named by year: ES2016, 2018, 2020 ...
New Features in ECMAScript 2016
This chapter introduces the new features in ECMAScript 2016:
- JavaScript Exponentiation (**)
- JavaScript Exponentiation assignment (**=)
- JavaScript Array includes()
Browser Support
ES 2016 is fully supported in all modern browsers since March 2017:
|
|
|
|
|
Chrome 52
|
Edge 15
|
Firefox 52
|
Safari 10.1
|
Opera 39
|
Jul 2016
|
Apr 2017
|
Mar 2017
|
May 2017
|
Aug 2016
|
ES 2016 is not supported in Internet Explorer.
Exponentiation Operator
The exponentiation operator (**) raises the first operand to the power of the second operand.
Example
let x = 5; let z = x ** 2;
-
x ** y produces the same result as Math.pow(x, y):
Example
let x = 5; let z = Math.pow(x,2);
-
Exponentiation Assignment
The exponentiation assignment operator (**=) raises the value of a variable to the power of the right operand.
Example
let x = 5; x **= 2;
-
The Exponentiation Operator is supported in all modern browsers since March 2017:
|
|
|
|
|
Chrome 52
|
Edge 14
|
Firefox 52
|
Safari 10.1
|
Opera 39
|
Jul 2016
|
Aug 2016
|
Mar 2017
|
Mar 2017
|
Aug 2016
|
JavaScript Array includes()
ECMAScript 2016 introduced Array.includes to arrays.
This allows us to check if an element is present in an array:
Example
const fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.includes("Mango");
-
Array.includes is supported in all modern browsers since August 2016:
|
|
|
|
|
Chrome 47
|
Edge 14
|
Firefox 43
|
Safari 9
|
Opera 34
|
Dec 2015
|
Aug 2016
|
Dec 2015
|
Oct 2015
|
Dec 2015
|
JavaScript Version Numbers
Old ECMAScript versions was named by numbers: ES5 and ES6.
From 2016, versions are named by year: ES2016, 2018, 2020 ...
New Features in ECMAScript 2017
This chapter introduces the new features in ECMAScript 2017:
- JavaScript String padding
- JavaScript Object entries()
- JavaScript Object values()
- JavaScript async and await
- Trailing Commas in Functions
- JavaScript Object.getOwnPropertyDescriptors
ES 2017 is fully supported in all modern browsers since September 2017:
|
|
|
|
|
Chrome 57
|
Edge 15
|
Firefox 48
|
Safari 11
|
Opera 44
|
Mar 2017
|
Apr 2017
|
Aug 2016
|
Sep 2017
|
Mar 2017
|
JavaScript String Padding
ECMAScript 2017 added two string methods to JavaScript: padStart() and padEnd() to support padding at the beginning and at the end of a string.
Examples
let text = "5"; text = text.padStart(4,0);
-
let text = "5"; text = text.padEnd(4,0);
-
JavaScript string padding is supported in all modern browsers since April 2017:
|
|
|
|
|
Chrome 57
|
Edge 15
|
Firefox 48
|
Safari 10
|
Opera 44
|
Mar 2017
|
Apr 2017
|
Aug 2016
|
Sep 2016
|
Mar 2017
|
JavaScript Object Entries
ECMAScript 2017 added the Object.entries() method to objects.
Object.entries() returns an array of the key/value pairs in an object:
Example
const person = { firstName : "John", lastName : "Doe", age : 50, eyeColor : "blue" };
let text = Object.entries(person);
-
Object.entries() makes it simple to use objects in loops:
Example
const fruits = {Bananas:300, Oranges:200, Apples:500};
let text = ""; for (let [fruit, value] of Object.entries(fruits)) { text += fruit + ": " + value + "<br>"; }
-
Object.entries() also makes it simple to convert objects to maps:
Example
const fruits = {Bananas:300, Oranges:200, Apples:500};
const myMap = new Map(Object.entries(fruits));
-
Object.entries() is supported in all modern browsers since March 2017:
|
|
|
|
|
Chrome 47
|
Edge 14
|
Firefox 47
|
Safari 10.1
|
Opera 41
|
Jun 2016
|
Aug 2016
|
Jun 2016
|
Mar 2017
|
Oct 2016
|
JavaScript Object Values
Object.values() are similar to Object.entries(), but returns a single dimension array of the object values:
Example
const person = { firstName : "John", lastName : "Doe", age : 50, eyeColor : "blue" };
let text = Object.values(person);
-
Object.values() is supported in all modern browsers since March 2017:
|
|
|
|
|
Chrome 54
|
Edge 14
|
Firefox 47
|
JavaScript Version Numbers
Old ECMAScript versions was named by numbers: ES5 and ES6.
From 2016, versions are named by year: ES2016, 2018, 2020 ...
New Features in ECMAScript 2018
This chapter introduces the new features in ECMAScript 2018:
- Asynchronous Iteration
- Promise Finally
- Object Rest Properties
- New RegExp Features
- JavaScript Shared Memory
JavaScript Asynchronous Iteration
ECMAScript 2018 added asynchronous iterators and iterables.
With asynchronous iterables, we can use the await keyword in for/of loops.
Example
for await () {}
JavaScript asynchronous iteration is supported in all modern browsers since January 2020:
|
|
|
|
|
Chrome 63
|
Edge 79
|
Firefox 57
|
Safari 11
|
Opera 50
|
Dec 2017
|
Jan 2020
|
Nov 2017
|
Sep 2017
|
Jan 2018
|
JavaScript Promise.finally
ECMAScript 2018 finalizes the full implementation of the Promise object with Promise.finally:
Example
let myPromise = new Promise();
myPromise.then(); myPromise.catch(); myPromise.finally();
Promise.finally is supported in all modern browsers since November 2018:
|
|
|
|
|
Chrome 63
|
Edge 18
|
Firefox 58
|
Safari 11.1
|
Opera 50
|
Dec 2017
|
Nov 2018
|
Jan 2018
|
Mar 2018
|
Jan 2018
|
JavaScript Object Rest Properties
ECMAScript 2018 added rest properties.
This allows us to destruct an object and collect the leftovers onto a new object:
Example
let { x, y, ...z } = { x: 1, y: 2, a: 3, b: 4 }; x; // 1 y; // 2 z; // { a: 3, b: 4 }
Object rest properties is supported in all modern browsers since January 2020:
|
|
|
|
|
Chrome 60
|
Edge 79
|
Firefox 55
|
Safari 11.1
|
Opera 47
|
Jul 2017
|
Jan 2020
|
Aug 2017
|
Mar 2018
|
Aug 2017
|
New JavaScript RegExp Features
ECMAScript 2018 added 4 new RegExp features:
- Unicode Property Escapes (p{...})
- Lookbehind Assertions (?<= ) and (?<! )
- Named Capture Groups
- s (dotAll) Flag
The new RegExp features is supported in all modern browsers since June 2020:
|
|
|
|
|
Chrome 64
|
Edge 79
|
Firefox 78
|
Safari 12
|
Opera 51
|
Jan 2018
|
Jan 2020
|
Jun 2020
|
Sep 2018
|
Feb 2018
|
JavaScript Threads
In JavaScript you use the Web Workers API to create threads.
Worker threads are used to execute code in the background so that the main program can continue execution.
Worker threads run simultaneously with the main program. Simultaneous execution of different parts of a program can be time-saving.
JavaScript Shared Memory
Shared memory is a feature that allows threads (different parts of a program) to access and update the same data in the same memory.
Instead of passing data between threads, you can pass a SharedArrayBuffer object that points to the memory where data is saved.
SharedArrayBuffer
A SharedArrayBuffer object represents a fixed-length raw binary data buffer similar to the ArrayBuffer object.
JavaScript Version Numbers
Old ECMAScript versions was named by numbers: ES5 and ES6.
From 2016, versions are named by year: ES2016, 2018, 2020 ...
New Features in ES2019
- String.trimStart()
- String.trimEnd()
- Object.fromEntries
- Optional catch binding
- Array.flat()
- Array.flatMap()
- Revised Array.Sort()
- Revised JSON.stringify()
- Separator symbols allowed in string litterals
- Revised Function.toString()
Warning
These features are relatively new.
Older browsers may need an alternative code (Polyfill)
JavaScript String trimStart()
ES2019 added the String method trimStart() to JavaScript.
The trimStart() method works like trim(), but removes whitespace only from the start of a string.
Example
let text1 = " Hello World! "; let text2 = text1.trimStart();
-
JavaScript String trimStart() is supported in all modern browsers since January 2020:
|
|
|
|
|
Chrome 66
|
Edge 79
|
Firefox 61
|
Safari 12
|
Opera 50
|
Apr 2018
|
Jan 2020
|
Jun 2018
|
Sep 2018
|
May 2018
|
JavaScript String trimEnd()
ES2019 added the String method trimEnd() to JavaScript.
The trimEnd() method works like trim(), but removes whitespace only from the end of a string.
Example
let text1 = " Hello World! "; let text2 = text1.trimEnd();
-
JavaScript String trimEnd() is supported in all modern browsers since January 2020:
|
|
|
|
|
Chrome 66
|
Edge 79
|
Firefox 61
|
Safari 12
|
Opera 50
|
Apr 2018
|
Jan 2020
|
Jun 2018
|
Sep 2018
|
May 2018
|
JavaScript Object fromEntries()
ES2019 added the Object method fromEntries() to JavaScript.
The fromEntries() method creates an object from iterable key / value pairs.
Example
const fruits = [ ["apples", 300], ["pears", 900], ["bananas", 500] ];
const myObj = Object.fromEntries(fruits);
-
JavaScript Object fromEntries() is supported in all modern browsers since January 2020:
|
|
|
|
|
Chrome 73
|
Edge 79
|
Firefox 63
|
Safari 12.1
|
Opera 60
|
Mar 2019
|
Jan 2020
|
Oct 2018
|
Mar 2019
|
Apr 2019
|
Optional catch Binding
From ES2019 you can omit the catch parameter if you don't need it:.
Example
Before 2019:
try { // code } catch (err) { // code }
After 2019:
try { // code } catch { // code }
Optional catch binding is supported in all modern browsers since January 2020:
|
|
|
|
|
Chrome 66
|
Edge 79
|
Firefox 58
|
Safari 11.1
|
Opera 53
|
Apr 2018
|
JavaScript Version Numbers
Old ECMAScript versions was named by numbers: ES5 and ES6.
From 2016, versions are named by year: ES2016, 2018, 2020 ...
New Features in ES2020
- BigInt
- String matchAll()
- The Nullish Coalescing Operator (??)
- The Optional Chaining Operator (?.)
- Logical AND Assignment Operator (&&=)
- Logical OR Assignment (||=)
- Nullish Coalescing Assignment (??=)
- Promise.allSettled()
- Dynamic Import
Warning
These features are relatively new.
Older browsers may need an alternative code (Polyfill)
JavaScript BigInt
JavaScript BigInt variables are used to store big integer values that are too big to be represented by a a normal JavaScript Number.
JavaScript integers are only accurate up to about 15 digits.
Integer Example
let x = 999999999999999; let y = 9999999999999999; // too big
-
BigInt Example
let x = 9999999999999999; let y = 9999999999999999n;
-
To create a BigInt, append n to the end of an integer or call BigInt():
Example
let x = 1234567890123456789012345n; let y = BigInt(1234567890123456789012345)
-
The JavaScript typeof a BigInt is "bigint":
Example
let x = BigInt(999999999999999); let type = typeof x;
-
BigInt is supported in all modern browsers since September 2020:
|
|
|
|
|
Chrome 67
|
Edge 79
|
Firefox 68
|
Safari 14
|
Opera 54
|
May 2018
|
Jan 2020
|
Jul 2019
|
Sep 2020
|
Jun 2018
|
JavaScript String matchAll()
Before ES2020 there was no string method that could be used to search for all occurrences of a string in a string.
Example
const iterator = text.matchAll("Cats");
-
If the parameter is a regular expression, the global flag (g) must be set set, otherwise a TypeError is thrown.
Example
const iterator = text.matchAll(/Cats/g);
-
If you want to search case insensitive, the insensitive flag (i) must be set:
Example
const iterator = text.matchAll(/Cats/gi);
-
Note
ES2021 introduced the string method replaceAll().
The Nullish Coalescing Operator (??)
The ?? operator returns the first argument if it is not nullish (null or undefined).
Otherwise it returns the second.
Example
let name = null; let text = "missing"; let result = name ?? text;
-
The nullish operator is supported in all modern browsers since March 2020:
|
|
|
|
|
Chrome 80
|
Edge 80
|
Firefox 72
|
Safari 13.1
|
Opera 67
|
Feb 2020
|
Feb 2020
|
Jan 2020
|
Mar 2020
|
Mar 2020
|
The Optional Chaining Operator (?.)
The Optional Chaining Operator returns undefined if an object is undefined or null (instead of throwing an error).
Example
const car = {type:"Fiat", model:"500", color:"white"}; let name = car?.name;
-
The ?.= operator is supported in all modern browsers since March 2020:
|
|
|
|
|
Chrome 80
|
JavaScript Version Numbers
Old ECMAScript versions was named by numbers: ES5 and ES6.
From 2016, versions are named by year: ES2016, 2018, 2020 ...
New Features in ES2021
- Promise.any()
- String replaceAll()
- Numeric Separators (_)
Warning
These features are relatively new.
Older browsers may need an alternative code (Polyfill)
JavaScript Promise.any()
Example
// Create a Promise const myPromise1 = new Promise((resolve, reject) => { setTimeout(resolve, 200, "King"); });
// Create another Promise const myPromise2 = new Promise((resolve, reject) => { setTimeout(resolve, 100, "Queen"); });
// Run when any promise fulfill Promise.any([myPromise1, myPromise2]).then((x) => { myDisplay(x); });
-
Promise.any() is supported in all modern browsers since September 2020:
|
|
|
|
|
Chrome 85
|
Edge 85
|
Firefox 79
|
Safari 14
|
Opera 71
|
Aug 2019
|
Aug 2020
|
Jul 2020
|
Sep 2020
|
Sep 2020
|
JavaScript String ReplaceAll()
ES2021 introduced the string method replaceAll():
Example
text = text.replaceAll("Cats","Dogs"); text = text.replaceAll("cats","dogs");
-
The replaceAll() method allows you to specify a regular expression instead of a string to be replaced.
If the parameter is a regular expression, the global flag (g) must be set, otherwise a TypeError is thrown.
Example
text = text.replaceAll(/Cats/g,"Dogs"); text = text.replaceAll(/cats/g,"dogs");
-
Note
ES2020 introduced the string method matchAll().
JavaScript Numeric Separator (_)
ES2021 intoduced the numeric separator (_) to make numbers more readable:
Example
const num = 1_000_000_000;
-
The numeric separator is only for visual use.
Example
const num1 = 1_000_000_000; const num2 = 1000000000; (num1 === num2);
-
The numeric separator can be placed anywhere in a number:
Example
const num1 = 1_2_3_4_5;
-
Note
The numeric separator is not allowed at the beginning or at the end of a number.
In JavaScript only variables can start with _.
The numeric separator is supported in all modern browsers since January 2020:
|
|
|
|
|
Chrome 75
|
Edge 79
|
Firefox 74
|
Safari 13.1
|
Opera 67
|
Jun 2019
|
Jan 2020
|
Oct 2019
|
Sep 2019
|
Jun 2019
|
Microsoft ended all support for Internet Explorer June 15, 2022.
Internet Explorer Retirement Dates:
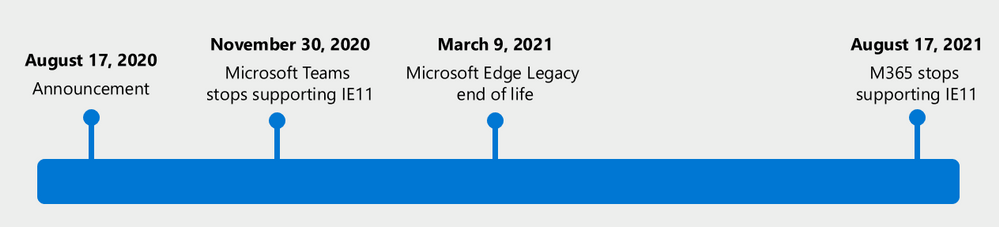
17-08-2020
|
This announcement
|
30-11-2020
|
MS Teams ended support for IE 11
|
31-12-2020
|
Azure DevOps Services ended support for IE 11
|
31-03-2021
|
Azure Portal ended support for IE 11
|
17-08-2021
|
Microsoft ended support for IE 11 across web services: Office 365 - OneDrive Outlook
|
15-06-2022
|
Microsoft ends all support for IE 11
|
-
Earlier Announcements
Windows 11 Removes Internet Explorer
Reported 24-06-2021:
Internet Explorer is disabled in Windows 11
Source: Windows 11 specifications
|
Windows 10 Replaces Internet Explorer
Reported 15-06-2020:
Internet Explorer is "replaced" by Edge in Windows 10
-
|
Internet Explorer Retires
Reported 15-06-2020:
IE 11 goes out of support on June 15 2022
-
|
Microsoft Edge Legacy
Edge was the default browser for Windows 10.
It was built with Microsoft's browser engine EdgeHTML and their Chakra JavaScript engine.
The first versions of Edge (12-18), are now referred to as "Edge Legacy".
The Microsoft support for Edge Legacy ended on March 9, 2021.
Future Windows updates will replace Edge Legacy with The New Edge.
Retirement dates published August 17, 2020:
31-12-2020
|
Azure DevOps Services stops supporting Edge Legacy
|
09-03-2021
|
End of Windows updates for Edge Legacy
|
13-04-2021
|
Future Windows updates will remove Edge Legacy
|
-
The New Edge
The new Microsoft Edge is Chromium based with Blink and V8 engines.
It was released in January 2020, and is available for Windows 7, 8, and 10.
It can also be downloaded for macOS, iOS, and Android.
The new Edge follows the Modern Lifecycle Policy (updates on an approx. six-week cycle).
Google
Google Ad Manager
As of March 28, 2022, Google Ad Manager no longer supports ad serving for Internet Explorer versions 11 and below.
-
Google Search
As of October 1, 2021, Google Search no longer supports Internet Explorer 11.
-
Google Workspace
As of March 15, 2021, Google Workspace no longer supports Internet Explorer 11.
-
Old Operating Systems
Internet Explorer 11 is still a component in some old Windows operating system and follows the Lifecycle Policy for these products:
System
|
Default Browser
|
Windows 7
|
Internet Explorer 11
|
Windows 8.X
|
Internet Explorer 11
|
Windows 10
|
Internet Explorer 11
|
Windows Server 2012
|
Internet Explorer 11
|
Windows Server 2012 R2
|
Internet Explorer 11
|
Windows Server 2016
|
Internet Explorer 11
|
Windows Embedded Standard 7
|
Internet Explorer 11
|
Windows Embedded POSReady 7
|
Internet Explorer 11
|
Windows Thin PC
|
Internet Explorer 11
|
Windows Embedded 8 Standard
|
Internet Explorer 11
|
Windows 8.1 Industry Update
|
Internet Explorer 11
|
Old Applications
Some old PCs (like in public libraries) are still using Internet Explorer.
Some legacy web applications are using Internet Explorer.
Some legacy web applications are using Internet Explorer runtime DLLs.
Some AJAX based applications are using Microsoft ActiveX components.
In order to ease the migration from Internet Explorer, Microsoft Edge offers Internet Explorer Mode, providing backward compatibility and enabling customers to continue to run legacy web applications.
JavaScript / ECMAScript
JavaScript was invented by Brendan Eich in 1995.
It was developed for Netscape 2, and became the ECMA-262 standard in 1997.
After Netscape handed JavaScript over to ECMA, the Mozilla foundation continued to develop JavaScript for the Firefox browser. Mozilla's latest version was 1.8.5. (Identical to ES5).
Internet Explorer (IE4) was the first browser to support ECMA-262 Edition 1 (ES1).
Year
|
ECMA
|
Browser
|
1995
|
|
JavaScript was invented by Brendan Eich
|
1996
|
|
Netscape 2 was released with JavaScript 1.0
|
1997
|
|
JavaScript became an ECMA standard (ECMA-262)
|
1997
|
ES1
|
ECMAScript 1 was released
|
1997
|
ES1
|
IE 4 was the first browser to support ES1
|
1998
|
ES2
|
ECMAScript 2 was released
|
1998
|
|
Netscape 42 was released with JavaScript 1.3
|
1999
|
ES2
|
IE 5 was the first browser to support ES2
|
1999
|
ES3
|
ECMAScript 3 was released
|
2000
|
ES3
|
IE 5.5 was the first browser to support ES3
|
2000
|
|
Netscape 62 was released with JavaScript 1.5
|
2000
|
|
Firefox 1 was released with JavaScript 1.5
|
2008
|
ES4
|
ECMAScript 4 was abandoned
|
2009
|
ES5
|
ECMAScript 5 was released
|
2011
|
ES5
|
IE 9 was the first browser to support ES5 *
|
2011
|
ES5
|
Firefox 4 was released with JavaScript 1.8.5
|
2012
|
ES5
|
Full support for ES5 in Safari 6
|
2012
|
ES5
|
Full support for ES5 in IE 10
|
2012
|
ES5
|
Full support for ES5 in Chrome 23
|
2013
|
ES5
|
Full support for ES5 in Firefox 21
|
2013
|
ES5
|
Full support for ES5 in Opera 15
|
2014
|
ES5
|
Full support for ES5 in all browsers
|
2015
|
ES6
|
ECMAScript 6 was released
|
2016
|
ES6
|
Full support for ES6 in Chrome 51
|
2016
|
ES6
|
Full support for ES6 in Opera 38
|
2016
|
ES6
|
Full support for ES6 in Safari 10
|
2017
|
ES6
|
Full support for ES6 in Firefox 54
|
2017
|
ES6
|
Full support for ES6 in Edge 15
|
2018
|
ES6
|
Full support for ES6 in all browsers **
|
Note
* Internet Explorer 9 did not support ES5 "use strict".
** Internet Explorer 11 does not support ES6.
The ECMA Technical Committee 39
In 1996, Netscape and Brendan Eich took JavaScript to the ECMA international standards organization, and a technical committee (TC39) was created to develop the language.
ECMA-262 Edition 1 was released in June 1997.
From ES4 to ES6
When the TC39 committee got together in Oslo in 2008, to agree on ECMAScript 4, they were divided into 2 very different camps:
The ECMAScript 3.1 Camp: Microsoft and Yahoo who wanted an incremental upgrade from ES3.
The ECMAScript 4 Camp: Adobe, Mozilla, Opera, and Google who wanted a massive ES4 upgrade.
August 13 2008, Brendan Eich wrote an email:
It's no secret that the JavaScript standards body, Ecma's Technical Committee 39, has been split for over a year, with some members favoring ES4, a major fourth edition to ECMA-262, and others advocating ES3.1 based on the existing ECMA-262 Edition 3 (ES3) specification. Now, I'm happy to report, the split is over.
The solution was to work together:
- ECMAScript 4 was renamed to ES5
- ES5 should be an incremental upgrade of ECMAScript 3.
- Features of ECMAScript 4 should be picked up in later versions.
- TC39 should develop a new major release, bigger in scope than ES5.
The planned new release (ES6) was codenamed "Harmony" (Because of the split it created?).
ES5 was a huge success. It was released in 2009, and all major browsers (including Internet Explorer) were fully compliant by July 2013:
|
|
|
|
|
Chrome 23
|
IE10 / Edge
|
Firefox 21
|
Safari 6
|
Opera 15
|
Nov 2012
|
Sep 2012
|
May 2013
|
JavaScript Objects
In JavaScript, objects are king. If you understand objects, you understand JavaScript.
In JavaScript, almost "everything" is an object.
- Booleans can be objects (if defined with the
new keyword)
- Numbers can be objects (if defined with the
new keyword)
- Strings can be objects (if defined with the
new keyword)
- Dates are always objects
- Maths are always objects
- Regular expressions are always objects
- Arrays are always objects
- Functions are always objects
- Objects are always objects
All JavaScript values, except primitives, are objects.
JavaScript Primitives
A primitive value is a value that has no properties or methods.
3.14 is a primitive value
A primitive data type is data that has a primitive value.
JavaScript defines 7 types of primitive data types:
Examples
string
number
boolean
null
undefined
symbol
bigint
Immutable
Primitive values are immutable (they are hardcoded and cannot be changed).
if x = 3.14, you can change the value of x, but you cannot change the value of 3.14.
Value |
Type |
Comment |
"Hello" |
string |
"Hello" is always "Hello" |
3.14 |
number |
3.14 is always 3.14 |
true |
boolean |
true is always true |
false |
boolean |
false is always false |
null |
null (object) |
null is always null |
undefined |
undefined |
undefined is always undefined |
Objects are Variables
JavaScript variables can contain single values:
Example
let person = "John Doe";
JavaScript variables can also contain many values.
Objects are variables too. But objects can contain many
values.
Object values are written as name : value pairs (name and value separated by a
colon).
Example
let person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
A JavaScript object is a collection of named values
It is a common practice to declare objects with the const keyword.
Example
const person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
Object Properties
The named values, in JavaScript objects, are called properties.
Property |
Value |
firstName |
John |
lastName |
Doe |
age |
50 |
eyeColor |
blue |
Objects written as name value pairs are similar to:
- Associative arrays in PHP
- Dictionaries in Python
- Hash tables in C
- Hash maps in Java
- Hashes in Ruby and Perl
Object Methods
Methods are actions that can be performed on objects.
Object properties can be both primitive values, other objects, and functions.
An object method is an object property containing a function
definition.
Property |
Value |
firstName |
John |
lastName |
Doe |
age |
50 |
eyeColor |
blue |
fullName |
function() {return this.firstName + " " + this.lastName;} |
JavaScript objects are containers for named values, called properties and methods.
You will learn more about methods in the next chapters.
Creating a JavaScript Object
With JavaScript, you can define and create your own objects.
There are different ways to create new objects:
- Create a single object, using an object literal.
- Create a single object, with the keyword
new .
- Define an object constructor, and then create objects of the constructed type.
- Create an object using
Object.create() .
Using an Object Literal
This is the easiest way to create a JavaScript Object.
Using an object literal, you both define and create an object in one
statement.
An object literal is a list of name:value pairs (like age:50) inside curly braces {}.
The following example creates a new JavaScript object with four properties:
Example
const person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
Spaces and line breaks are not important. An object definition can span multiple lines:
Example
const person = { Â Â firstName: "John", Â Â lastName: "Doe", Â Â age: 50, Â Â eyeColor: "blue" };
This example creates an empty JavaScript object,
and then adds 4 properties:
Example
const person = {};
person.firstName = "John";
person.lastName = "Doe";
person.age = 50;
person.eyeColor = "blue";
Using the JavaScript Keyword new
The following example create a new JavaScript object
using new Object() ,
and then adds 4 properties:
Example
const person = new Object();
person.firstName = "John";
person.lastName = "Doe";
person.age = 50;
person.eyeColor = "blue";
The examples above do exactly the same.
But there is no need to use new Object() .
For readability, simplicity and execution speed, use the object literal method.
JavaScript Objects are Mutable
Objects are mutable: They are addressed by reference, not by value.
If person is an object, the following statement will not create a copy of person:
const x = person;Â // Will not create a copy of person.
The object x is not a copy of person. It is
person.
Both x and person are the same object.
Any changes to x will also change person, because x and person are the same object.
Example
const person = {
 firstName:"John",
 lastName:"Doe",
 age:50, eyeColor:"blue"
}
const x = person;
x.age = 10;Â Â Â Â Â // Will change both x.age and person.age
JavaScript Object Properties
Properties are the most important part of any JavaScript object.
JavaScript Properties
Properties are the values associated with a JavaScript object.
A JavaScript object is a collection of unordered properties.
Properties can usually be changed, added, and deleted, but some are read only.
Accessing JavaScript Properties
The syntax for accessing the property of an object is:
objectName.property      // person.age
or
objectName["property"]Â Â Â // person["age"]
or
objectName[expression]Â Â // x = "age"; person[x]
The expression must evaluate to a property name.
Example 1
person.firstname + " is " + person.age + " years old.";
Example 2
person["firstname"] + " is " + person["age"] + " years old.";
JavaScript for...in Loop
The JavaScript for...in statement loops through the properties of an object.
Syntax
for (let variable in object) {
 // code to be executed
}
The block of code inside of the for...in loop will be executed once for each property.
Looping through the properties of an object:
Example
const person = {
 fname:" John",
 lname:" Doe",
 age: 25
};
for (let x in person) {
 txt += person[x];
}
Adding New Properties
You can add new properties to an existing object by simply giving it a value.
Assume that the person object already exists -
you can then give it new properties:
Example
person.nationality = "English";
Deleting Properties
The delete keyword deletes a property from an object:
Example
const person = {
 firstName: "John",
 lastName: "Doe",
 age: 50,
 eyeColor: "blue"
};
delete person.age;
or delete person["age"];
Example
const person = {
 firstName: "John",
 lastName: "Doe",
 age: 50,
 eyeColor: "blue"
};
delete person["age"];
The delete keyword deletes both the value of the property and the property itself.
After deletion, the property cannot be used before it is added back again.
The
delete operator is designed to be used on object properties. It has no effect on
variables or functions.
The delete operator should not be used on predefined JavaScript object
properties. It can crash your application.
Nested Objects
Values in an object can be another object:
Example
myObj = {
  name:"John",  age:30,
Â
cars: { Â Â Â car1:"Ford",
  Â
car2:"BMW", Â Â Â car3:"Fiat"
  } }
You can access nested objects using the dot notation or the bracket notation:
or:
Example
myObj.cars["car2"];
or:
Example
myObj["cars"]["car2"];
or:
Example
let p1 = "cars";
let p2 = "car2";
myObj[p1][p2];
Nested Arrays and Objects
Values in objects can be arrays, and values in arrays can be objects:
Example
const myObj =
{ Â Â name: "John", Â age: 30,
Â
cars: [ Â Â Â Â {name:"Ford",
models:["Fiesta", "Focus", "Mustang"]}, Â Â Â
{name:"BMW", models:["320", "X3", "X5"]},
  Â
{name:"Fiat", models:["500", "Panda"]}
 ] }
To access arrays inside arrays, use a for-in loop for each array:
Example
for (let i in myObj.cars) { Â x += "<h1>" + myObj.cars[i].name
+ "</h1>"; Â for (let j in myObj.cars[i].models) { Â Â Â
x += myObj.cars[i].models[j]; Â } }
Property Attributes
All properties have a name. In addition they also have a value.
The value is one of the property's attributes.
Other attributes are: enumerable, configurable, and writable.
These attributes define how the property can be accessed (is it readable?, is
it writable?)
In JavaScript, all attributes can be read, but only the value attribute can
be changed (and only if the property is writable).
( ECMAScript 5 has methods for both getting and setting all property
attributes)
Prototype Properties
JavaScript objects inherit the properties of their prototype.
The delete keyword does not delete inherited properties, but if you delete a
prototype property, it will affect all objects
inherited from the prototype.
JavaScript Object Methods
Example
const person = {
 firstName: "John",
  lastName: "Doe",
  id: 5566,
  fullName: function() {
   return this.firstName + " " +
this.lastName; Â Â }
};
What is this?
In JavaScript, the this keyword refers to an object.
Which object depends on how this is being invoked (used or called).
The this keyword refers to different objects depending on how it is used:
In an object method, this refers to the object. |
Alone, this refers to the global object. |
In a function, this refers to the global object. |
In a function, in strict mode, this is undefined . |
In an event, this refers to the element that received the event. |
Methods like call() , apply() ,
and bind() can refer this to any object. |
Note
this is not a variable. It is a keyword. You cannot change the value of this .
See Also:
JavaScript Methods
JavaScript methods are actions that can be performed on objects.
A JavaScript method is a property containing a function
definition.
Property |
Value |
firstName |
John |
lastName |
Doe |
age |
50 |
eyeColor |
blue |
fullName |
function() {return this.firstName + " " + this.lastName;} |
Methods are functions stored as object properties.
Accessing Object Methods
You access an object method with the following syntax:
You will typically describe fullName() as a method of the person object, and
fullName as a property.
The fullName property will execute (as a function) when it is invoked with ().
This example accesses the fullName() method of a person object:
Example
name = person.fullName();
If you access the fullName property, without (), it
will return the function definition:
Example
name = person.fullName;
Adding a Method to an Object
Adding a new method to an object is easy:
Example
person.name = function () { Â Â return this.firstName + " " + this.lastName; };
Using Built-In Methods
This example uses the toUpperCase() method of the String object, to convert a text
to uppercase:
let message = "Hello world!";
let x = message.toUpperCase();
The value of x, after execution of the code above will be:
Example
person.name = function () {
  return (this.firstName + " " + this.lastName).toUpperCase();
};
How to Display JavaScript Objects?
Displaying a JavaScript object will output [object Object].
Example
const person = {
 name: "John",
 age: 30,
 city: "New York"
};
document.getElementById("demo").innerHTML = person;
Some common solutions to display JavaScript objects are:
- Displaying the Object Properties by name
- Displaying the Object Properties in a Loop
- Displaying the Object using Object.values()
- Displaying the Object using JSON.stringify()
Displaying Object Properties
The properties of an object can be displayed as a string:
Example
const person = {
 name: "John",
 age: 30,
 city: "New York"
};
document.getElementById("demo").innerHTML =
person.name + "," + person.age + "," + person.city;
Displaying the Object in a Loop
The properties of an object can be collected in a loop:
Example
const person = {
 name: "John",
 age: 30,
 city: "New York"
};
let txt = "";
for (let x in person) {
txt += person[x] + " ";
};
document.getElementById("demo").innerHTML = txt;
You must use person[x] in the loop.
person.x will not work (Because x is a variable).
Using Object.values()
Any JavaScript object can be converted to an array using Object.values() :
const person = {
 name: "John",
 age: 30,
 city: "New York"
};
const myArray = Object.values(person);
myArray is now a JavaScript array, ready to be displayed:
Example
const person = {
 name: "John",
 age: 30,
 city: "New York"
};
const myArray = Object.values(person);
document.getElementById("demo").innerHTML = myArray;
Object.values() is supported in all major browsers since 2016.
|
|
|
|
|
54 (2016) |
14 (2016) |
47 (2016) |
10 (2016) |
41 (2016) |
Using JSON.stringify()
Any JavaScript object can be stringified (converted to a string) with the JavaScript function
JSON.stringify() :
const person = {
 name: "John",
 age: 30,
 city: "New York"
};
let myString = JSON.stringify(person);
myString is now a JavaScript string, ready to be displayed:
Example
const person = {
 name: "John",
 age: 30,
 city: "New York"
};
let myString = JSON.stringify(person);
document.getElementById("demo").innerHTML = myString;
The result will be a string following the JSON notation:
{"name":"John","age":50,"city":"New York"}
JSON.stringify() is included in JavaScript and supported in all major browsers.
Stringify Dates
JSON.stringify converts dates into strings:
Example
const person = {
 name: "John",
 today: new Date()
};
let myString = JSON.stringify(person);
document.getElementById("demo").innerHTML = myString;
Stringify Functions
JSON.stringify will not stringify functions:
Example
const person = {
 name: "John",
 age: function () {return 30;}
};
let myString = JSON.stringify(person);
document.getElementById("demo").innerHTML = myString;
This can be "fixed" if you convert the functions into strings before stringifying.
Example
const person = {
 name: "John",
 age: function () {return 30;}
};
person.age = person.age.toString();
let myString = JSON.stringify(person);
document.getElementById("demo").innerHTML = myString;
Stringify Arrays
It is also possible to stringify JavaScript arrays:
Example
const arr = ["John", "Peter", "Sally", "Jane"];
let myString = JSON.stringify(arr);
document.getElementById("demo").innerHTML = myString;
The result will be a string following the JSON notation:
["John","Peter","Sally","Jane"]
JavaScript Accessors (Getters and Setters)
ECMAScript 5 (ES5 2009) introduced Getter and Setters.
Getters and setters allow you to define Object Accessors (Computed
Properties).
JavaScript Getter (The get Keyword)
This example uses a lang property to get
the value of the language property.
Example
// Create an object:
const person = {
 firstName: "John",
 lastName: "Doe",
 language: "en",
 get lang() {
   return this.language;
 } };
// Display data from the object using a getter:
document.getElementById("demo").innerHTML = person.lang;
JavaScript Setter (The set Keyword)
This example uses a lang property to set
the value of the language property.
Example
const person = {
 firstName: "John",
  lastName: "Doe",
  language: "",
 set lang(lang) {
    this.language = lang;
  } };
// Set an object
property using a setter:
person.lang = "en";
// Display data from the object:
document.getElementById("demo").innerHTML = person.language;
JavaScript Function or Getter?
What is the differences between these two examples?
Example 1
const person = {
 firstName: "John",
 lastName: "Doe",
 fullName: function() {
   return this.firstName + " " +
this.lastName; Â Â }
};
// Display data from the object using a method:
document.getElementById("demo").innerHTML = person.fullName();
Example 2
const person = {
 firstName: "John",
 lastName: "Doe",
 get fullName() {
   return this.firstName + " " +
this.lastName; Â }
};
// Display data from the object using a getter:
document.getElementById("demo").innerHTML = person.fullName;
Example 1 access fullName as a function: person.fullName().
Example 2 access fullName as a property: person.fullName.
The second example provides a simpler syntax.
Data Quality
JavaScript can secure better data quality when using getters and setters.
Using the lang property, in this example, returns the value
of the language property in upper case:
Example
// Create an object:
const person = {
  firstName: "John",
  lastName: "Doe",
 language: "en",
 get lang() {
   return this.language.toUpperCase();
  } };
// Display data from the object using a getter:
document.getElementById("demo").innerHTML = person.lang;
Using the lang property, in this example, stores an upper case
value in the language property:
Example
const person = {
 firstName: "John",
  lastName: "Doe",
 language: "",
  set lang(lang) {
   this.language = lang.toUpperCase();
 } };
// Set an object
property using a setter:
person.lang = "en";
// Display data from the object:
document.getElementById("demo").innerHTML = person.language;
Why Using Getters and Setters?
- It gives simpler syntax
- It allows equal syntax for properties and methods
- It can secure better data quality
- It is useful for doing things behind-the-scenes
Object.defineProperty()
The Object.defineProperty() method can also be used to add Getters and
Setters:
A Counter Example
// Define object
const obj = {counter : 0};
// Define setters and getters
Object.defineProperty(obj, "reset", {
  get : function () {this.counter = 0;} });
Object.defineProperty(obj, "increment", {
  get : function () {this.counter++;} });
Object.defineProperty(obj, "decrement", {
  get : function () {this.counter--;} });
Object.defineProperty(obj, "add", {
  set : function (value) {this.counter += value;} });
Object.defineProperty(obj, "subtract", {
  set : function (value) {this.counter -= value;} });
// Play with the counter:
obj.reset;
obj.add = 5;
obj.subtract = 1;
obj.increment;
obj.decrement;
JavaScript Object Constructors
Example
function Person(first, last, age, eye) {
  this.firstName = first;
  this.lastName = last;
  this.age = age;
  this.eyeColor = eye;
}
Notes
It is considered good practice to name constructor functions with an upper-case first letter.
About this
In a constructor function this does not have a value.
It is a substitute for the new object. The value of this will become the new object when
a new object is created.
See Also:
Object Types (Blueprints) (Classes)
The examples from the previous chapters are limited. They only create single objects.
Sometimes we need a "blueprint" for creating many objects of the same "type".
The way to create an "object type", is to use an object constructor function.
In the example above, function Person() is an object constructor function.
Objects of the same type are created by calling the constructor function with the new keyword:
const myFather = new Person("John", "Doe", 50, "blue");
const myMother = new Person("Sally", "Rally", 48, "green");
What is this?
In JavaScript, the this keyword refers to an object.
Which object depends on how this is being invoked (used or called).
The this keyword refers to different objects depending on how it is used:
In an object method, this refers to the object. |
Alone, this refers to the global object. |
In a function, this refers to the global object. |
In a function, in strict mode, this is undefined . |
In an event, this refers to the element that received the event. |
Methods like call() , apply() ,
and bind() can refer this to any object. |
Note
this is not a variable. It is a keyword. You cannot change the value of this .
See Also:
Adding a Property to an Object
Adding a new property to an existing object is easy:
Example
myFather.nationality = "English";
The property will be added to myFather. Not to myMother. (Not to any other person objects).
Adding a Method to an Object
Adding a new method to an existing object is easy:
Example
myFather.name = function () { Â Â return this.firstName + " " + this.lastName; };
The method will be added to myFather. Not to myMother. (Not to any other person objects).
Adding a Property to a Constructor
You cannot add a new property to an object constructor the same way you
add a new property to an existing object:
Example
Person.nationality = "English";
To add a new property to a constructor, you must add it to the
constructor function:
Example
function Person(first, last, age, eyecolor) {
Â
this.firstName = first;
Â
this.lastName = last;
Â
this.age = age;
  this.eyeColor = eyecolor;   this.nationality = "English";
}
This way object properties can have default values.
Adding a Method to a Constructor
Your constructor function can also define methods:
Example
function Person(first, last, age, eyecolor) {
Â
this.firstName = first;
Â
this.lastName = last;
Â
this.age = age;
Â
this.eyeColor = eyecolor; Â Â this.name = function() {
    return this.firstName + " " + this.lastName;
  };
}
You cannot add a new method to an object constructor the same way you add a
new method to an existing object.
Adding methods to an object constructor must be done inside the
constructor function:
Example
function Person(firstName, lastName, age, eyeColor) {
  this.firstName = firstName;Â
Â
this.lastName = lastName;
Â
this.age = age;
  this.eyeColor = eyeColor;
  this.changeName = function (name) {
  Â
this.lastName = name;
  };
}
The changeName() function assigns the value of name to the person's
lastName property.
Now You Can Try:
myMother.changeName("Doe");
JavaScript knows which person you are
talking about by "substituting" this with myMother.
Built-in JavaScript Constructors
JavaScript has built-in constructors for native objects:
new String()Â Â Â // A new String object
new Number()Â Â Â // A new Number object
new Boolean()Â Â // A new Boolean object
new Object()Â Â Â // A new Object object
new Array()Â Â Â Â // A new Array object
new RegExp()Â Â Â // A new RegExp object
new Function()Â // A new Function object
new Date()Â Â Â Â Â // A new Date object
The Math() object is not in the list. Math is a global object. The new keyword cannot be used on
Math .
Did You Know?
As you can see above, JavaScript has object versions of the primitive
data types String , Number , and Boolean . But there is no reason to create complex objects. Primitive values
are much faster:
Use string literals "" instead of new String() .
Use number literals 50 instead of new Number() .
Use boolean literals true / false instead of new Boolean() .
Use object literals {} instead of new Object() .
Use array literals [] instead of new Array() .
Use pattern literals /()/ instead of new RegExp() .
Use function expressions () {} instead of new Function() .
Example
let x1 = "";Â Â Â Â Â Â Â Â Â Â Â Â // new primitive string
let x2 = 0;Â Â Â Â Â Â Â Â Â Â Â Â Â // new primitive number
let x3 = false;Â Â Â Â Â Â Â Â Â // new primitive boolean
const x4 = {};Â Â Â Â Â Â Â Â Â Â // new Object object
const x5 = [];Â Â Â Â Â Â Â Â Â Â // new Array object
const x6 = /()/Â Â Â Â Â Â Â Â Â // new RegExp object
const x7 = function(){};Â // new function
String Objects
Normally, strings are created as primitives: firstName = "John"
But strings can also be created as objects using the new keyword:
firstName = new String("John")
Learn why strings should not be created as object in the chapter
.
Number Objects
Normally, numbers are created as primitives: x = 30
But numbers can also be created as objects using the new keyword:
x = new
Number(30)
Learn why numbers should not be created as object in the chapter
.
Boolean Objects
Normally, booleans are created as primitives: x =
false
But booleans can also be created as objects using the new keyword:
x = new Boolean(false)
Learn why booleans should not be created as object in the chapter
.
JavaScript Object Prototypes
All JavaScript objects inherit properties and methods
from a prototype.
In the previous chapter we learned how to use an object constructor:
Example
function Person(first, last, age, eyecolor) {
Â
this.firstName = first;
  this.lastName = last;
Â
this.age = age;
Â
this.eyeColor = eyecolor;
}
const myFather = new Person("John", "Doe", 50, "blue");
const myMother = new Person("Sally", "Rally", 48, "green");
We also learned that you can not add a new property to an existing object constructor:
Example
Person.nationality = "English";
To add a new property to a constructor, you must add it to the
constructor function:
Example
function Person(first, last, age, eyecolor) {
Â
this.firstName = first;
Â
this.lastName = last;
Â
this.age = age;
Â
this.eyeColor = eyecolor; Â Â this.nationality = "English";
}
Prototype Inheritance
All JavaScript objects inherit properties and methods from a prototype:
-
Date objects inherit from Date.prototype
-
Array objects inherit from Array.prototype
-
Person objects inherit from Person.prototype
The Object.prototype is on the top of the prototype inheritance chain:
Date objects, Array objects, and Person objects inherit from Object.prototype .
Adding Properties and Methods to Objects
Sometimes you want to add new properties (or methods) to all existing objects of a given type.
Sometimes you want to add new properties (or methods) to an object
constructor.
Using the prototype Property
The JavaScript prototype property allows you to add new properties to object
constructors:
Example
function Person(first, last, age, eyecolor) {
 this.firstName = first;
 this.lastName = last;
 this.age = age;
 this.eyeColor = eyecolor;
}
Person.prototype.nationality = "English";
The JavaScript prototype property also allows you to add new methods to objects
constructors:
Example
function Person(first, last, age, eyecolor) {
 this.firstName = first;
 this.lastName = last;
 this.age = age;
 this.eyeColor = eyecolor;
}
Person.prototype.name = function() {
 return this.firstName + " " + this.lastName;
};
Only modify your own prototypes. Never modify the prototypes of
standard JavaScript objects.
Iterable objects are objects that can be iterated over with for..of .
Technically, iterables must implement the Symbol.iterator method.
Iterating Over a String
You can use a for..of loop to iterate over the elements of a string:
Example
for (const x of "W3Schools") {
  // code block to be executed
}
Iterating Over an Array
You can use a for..of loop to iterate over the elements of an Array:
Example
for (const x of [1,2,3,4,5]) {
  // code block to be executed
}
JavaScript Iterators
The iterator protocol defines how to produce a sequence of values from an object.
An object becomes an iterator when it implements a next() method.
The next() method must return an object with two properties:
- value (the next value)
- done (true or false)
value |
The value returned by the iterator
(Can be omitted if done is true) |
done |
true if the iterator has completed
false if the iterator has produced a new value |
Home Made Iterable
This iterable returns never ending: 10,20,30,40,.... Everytime
next() is called:
Example
// Home Made Iterable
function myNumbers() {
  let n = 0;
  return {
    next: function() {
      n += 10;
      return {value:n, done:false};
    }
  };
}
// Create Iterable
const n = myNumbers();
n.next(); // Returns 10
n.next(); // Returns 20
n.next(); // Returns 30
The problem with a home made iterable:
It does not support the JavaScript for..of statement.
A JavaScript iterable is an object that has a Symbol.iterator.
The Symbol.iterator is a function that returns a next() function.
An iterable can be iterated over with the code: for (const x of iterable) { }
Example
// Create an Object
myNumbers = {};
// Make it Iterable
myNumbers[Symbol.iterator] = function() {
  let n = 0;
  done = false;
  return {
    next() {
      n += 10;
      if (n == 100) {done = true}
      return {value:n, done:done};
    }
  };
}
Now you can use for..of
for (const num of myNumbers) {
  // Any Code Here
}
The Symbol.iterator method is called automatically by for..of .
But we can also do it "manually":
Example
let iterator = myNumbers[Symbol.iterator]();
while (true) {
  const result = iterator.next();
  if (result.done) break;
  // Any Code Here
}
A JavaScript Set is a collection of unique values.
Each value can only occur once in a Set.
A Set can hold any value of any data type.
Set Methods
Method
|
Description
|
new Set()
|
Creates a new Set
|
add()
|
Adds a new element to the Set
|
delete()
|
Removes an element from a Set
|
has()
|
Returns true if a value exists
|
clear()
|
Removes all elements from a Set
|
forEach()
|
Invokes a callback for each element
|
values()
|
Returns an Iterator with all the values in a Set
|
keys()
|
Same as values()
|
entries()
|
Returns an Iterator with the [value,value] pairs from a Set
|
Property
|
Description
|
size
|
Returns the number elements in a Set
|
How to Create a Set
You can create a JavaScript Set by:
- Passing an Array to new Set()
- Create a new Set and use add() to add values
- Create a new Set and use add() to add variables
The new Set() Method
Pass an Array to the new Set() constructor:
Example
// Create a Set const letters = new Set(["a","b","c"]);
-
Create a Set and add literal values:
Example
// Create a Set const letters = new Set();
// Add Values to the Set letters.add("a"); letters.add("b"); letters.add("c");
-
Create a Set and add variables:
Example
// Create Variables const a = "a"; const b = "b"; const c = "c";
// Create a Set const letters = new Set();
// Add Variables to the Set letters.add(a); letters.add(b); letters.add(c);
-
The add() Method
Example
letters.add("d"); letters.add("e");
-
If you add equal elements, only the first will be saved:
Example
letters.add("a"); letters.add("b"); letters.add("c"); letters.add("c"); letters.add("c"); letters.add("c"); letters.add("c"); letters.add("c");
-
The forEach() Method
The forEach() method invokes a function for each Set element:
Example
// Create a Set const letters = new Set(["a","b","c"]);
// List all entries let text = ""; letters.forEach (function(value) { text += value; })
-
The values() Method
The values() method returns an Iterator object containing all the values in a Set:
Example
letters.values() // Returns [object Set Iterator]
-
Now you can use the Iterator object to access the elements:
Example
// Create an Iterator const myIterator = letters.values();
// List all Values let text = ""; for (const entry of myIterator) { text += entry; }
-
The keys() Method
A Set has no keys.
keys() returns the same as values().
This makes Sets compatible with Maps.
Example
letters.keys() // Returns [object Set Iterator]
-
The entries() Method
A Set has no keys.
entries() returns [value,value] pairs instead of [key,value] pairs.
This makes Sets compatible with Maps:
Example
A Map holds key-value pairs where the keys can be any datatype.
A Map remembers the original insertion order of the keys.
A Map has a property that represents the size of the map.
Map Methods
Method
|
Description
|
new Map()
|
Creates a new Map object
|
set()
|
Sets the value for a key in a Map
|
get()
|
Gets the value for a key in a Map
|
clear()
|
Removes all the elements from a Map
|
delete()
|
Removes a Map element specified by a key
|
has()
|
Returns true if a key exists in a Map
|
forEach()
|
Invokes a callback for each key/value pair in a Map
|
entries()
|
Returns an iterator object with the [key, value] pairs in a Map
|
keys()
|
Returns an iterator object with the keys in a Map
|
values()
|
Returns an iterator object of the values in a Map
|
Property
|
Description
|
size
|
Returns the number of Map elements
|
How to Create a Map
You can create a JavaScript Map by:
- Passing an Array to
new Map()
- Create a Map and use
Map.set()
new Map()
You can create a Map by passing an Array to the new Map() constructor:
Example
-
Map.set()
You can add elements to a Map with the set() method:
Example
-
The set() method can also be used to change existing Map values:
Example
fruits.set("apples", 500);
-
Map.get()
The get() method gets the value of a key in a Map:
Example
fruits.get("apples");
-
Map.size
The size property returns the number of elements in a Map:
Example
fruits.size;
-
Map.delete()
The delete() method removes a Map element:
Example
fruits.delete("apples");
-
Map.clear()
The clear() method removes all the elements from a Map:
Example
fruits.clear();
-
Map.has()
The has() method returns true if a key exists in a Map:
Example
fruits.has("apples");
-
Try This:
fruits.delete("apples"); fruits.has("apples");
-
Maps are Objects
typeof returns object:
Example
-
instanceof Map returns true:
Example
-
JavaScript Objects vs Maps
Differences between JavaScript Objects and Maps:
Object
|
Map
|
Not directly iterable
|
Directly iterable
|
Do not have a size property
|
Have a size property
|
Keys must be Strings (or Symbols)
|
Keys can be any datatype
|
Keys are not well ordered
|
Keys are ordered by insertion
|
Have default keys
|
Do not have default keys
|
Map.forEach()
The forEach() method invokes a callback for each key/value pair in a Map:
Example
-
Map.entries()
The entries() method returns an iterator object with the [key,values] in a Map:
Example
| | | | | | | |